- Lab
- Core Tech
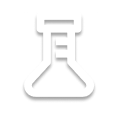
Guided: Using Structures, Unions, and Custom Data Types in C
In order to take advantage of the full power of C as a programming language, you need to learn how to create custom data types. By creating your own inventory management system, this lab will teach you how to use structs, custom data types, and unions in a real C program.
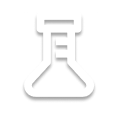
Path Info
Table of Contents
-
Challenge
### Introduction to Structures and Custom Data Types
Welcome to "Using Structures, Unions, and Custom Data Types in C"! In this Code Lab, you will journey through the creation of an inventory management CLI program, utilizing structures to define your products and inventory. Structures in C allow you to group related data items into a single cohesive unit, making it easier for you to manage complex data.
Benefits, Drawbacks, and Foundational Aspects
Structures offer significant benefits in your inventory system by providing a way to encapsulate product details in one place, enhancing code organization and readability. However, managing memory for dynamic structures can be complex, and improper handling might lead to memory leaks or data corruption. You need to understand how to structure data for products, manage inventory size, and access data efficiently. This step is crucial for anyone looking to handle more than just simple data types in C.
Domain Language
One of the best things about software development is that you can tailor the way you write your code such that it fits any given domain. Every domain, from business applications to undersea acoustics, comes with its own language that is specific to that domain. Custom data types via structures allow you to model your programs after the domain you are working in - both in how the data model looks and in how you name your data types.
In this lab you are working in the business domain. You will create both the Inventory and Product data types on your way to creating a CLI tool for managing products! Along the way, you’ll learn how to effectively use structures and union types to model data in your programs.
info > Note that you can check out the solution for this lab in the
solution
folder if you get stuck! -
Challenge
### Creating Custom Data Types with Structures
It's time to define the foundational data types for your inventory management CLI. Structures will be your tool for encapsulating product information in a way that's both intuitive and efficient. By defining these custom types, you'll create a clear, organized representation of an inventory system where each product can be treated as a single entity. With
typedef
, you'll simplify how you declare and use these structures, making your code more readable. This step is crucial as it sets the stage for all subsequent operations, from adding products to managing them dynamically in memory. Defining a structure for a product is the first step in organizing your inventory data. This structure will serve as the blueprint for each item in your system, allowing you to manage details like ID, name, and price in a cohesive manner. By placing this definition in a header file, you ensure that your product structure can be utilized across multiple source files, promoting code reuse and maintainability. This practice also helps in separating the interface from the implementation, making your project structure cleaner and more modular. Usingtypedef
to create an alias for your Product structure simplifies your code significantly. It reduces the verbosity when declaring variables or passing them as function parameters, making the code more readable and less prone to errors. This step is about enhancing your developer experience, allowing you to writeProduct item
instead ofstruct Product item
every time you reference your product structure. This practice is especially beneficial in larger projects where brevity and clarity can save a lot of time and reduce mistakes. The Inventory structure is your next step in building the CLI system's backbone. It will manage a collection of products, allowing you to add, view, or delete items dynamically. Usingtypedef
with this structure definition ensures consistency and ease of use throughout your codebase. This structure will be pivotal in managing the size and capacity of your inventory, providing a way to scale based on the number of products you handle, making your system adaptable to changing requirements. Functions that operate on your structures are essential for manipulating inventory data. Here, you'll define a function to add products to the inventory, which is one of the primary operations users will perform. This function will not only add a product but also manage the inventory's dynamic growth, ensuring you don't exceed capacity or waste memory. It's an excellent example of how structures and functions work together. -
Challenge
### Modeling Data with Unions
Now, you're going to utilize unions to model different product conditions within your inventory system. Unions offer a unique way to store one of several possible data types in the same memory space, which is perfect for representing varying product states where not all data is relevant at once. This approach will make your Product structure more flexible, allowing for conditions like new, used, or refurbished without wasting memory on unused fields. By integrating unions, you ensure your data model is both space-efficient and capable of handling diverse product scenarios. A union for product conditions allows you to represent different attributes based on the product's state, which is vital for an inventory where items come in various forms. This not only conserves memory but also reflects real-world scenarios where a product's attributes change based on its condition. By defining this in a header file, you ensure that this union can be shared across your project, maintaining consistency and reducing redundancy. Incorporating your union into the Product structure extends its capabilities, making it more versatile. This step is crucial because it allows you to model products in a way that closely matches real-world scenarios, where the same product might have different relevant attributes depending on its condition. This approach will make your inventory system more accurate and useful for end-users, providing them with the exact information they need based on the product's state. Having a function that can interpret the current state of a product based on its union data is essential for user interaction in your CLI. It allows for dynamic responses to queries about product conditions, making your system more interactive and user-friendly. This function will be particularly useful when users want to view or filter products based on specific conditions. This function will give you the stringified version of your product state which can then be used to determine the exact information that your union type member holds within it.
-
Challenge
### Managing Dynamic Structures
Dynamic memory management is where your inventory system truly comes to life, allowing it to adapt to user needs in real-time. Here, you'll delve into using
malloc
to allocate memory for your structures on the heap, enabling your inventory to grow or shrink as products are added or removed.The
malloc
function is the primary function provided to you by the C standard library when it comes to allocating memory dynamically. You just givemalloc
a size (in bytes) and it will hand you back a pointer to a block of memory at that size! For example, the following code will hand you back a pointer to four bytes of memory that is. meant to hold one integer:int *pointer = malloc(sizeof(int));
info > Note that it is a best practice to use the
sizeof
function with types to determine their byte sizes. This is especially useful when allocating memory for structures like your Product struct.This step is key to creating a responsive CLI program that can handle an unknown number of products efficiently, ensuring you only consume the memory you need while providing the flexibility for expansion. Initializing an inventory with dynamic allocation allows your system to start with a minimal footprint and expand as needed. This is fundamental for applications where the number of items can vary widely; it prevents over-allocation of memory at the outset while still allowing for growth. This task sets the foundation for a scalable inventory management system, directly impacting performance and resource usage. Dynamically creating products on the heap is essential for adding new items to your inventory without predefined limits. This capability ensures your system can handle user inputs for adding products at runtime, managing memory effectively. Handling arrays of products dynamically is where your inventory system becomes flexible but so far, you haven’t handled the case where you need to resize your
products
array. This task is pivotal for dealing with bulk operations or when loading existing inventories, making your system adaptable to different scales of use. A print function for the inventory is your window into the system's state. It's crucial for verifying data entries, debugging, or simply for you to interact with the inventory. This function will be called frequently through the CLI, providing feedback on the system's operation, making it an essential part of user interaction and system usability. Great work! If you have been able to validate all of these tasks, you should now be able to use theproduct-bot
program! You can compile and run this program by clicking the "Run" button in your terminal.The
product-bot
inventory CLI you just finished implementing lets you type the following commands interactively:add
: Prompts you for new product information- 'delete': Will prompt you for a product id and then will delete that product
view
: Will use theprintInventory
function you just finished implementing to let you view your inventory.quit
: Will end theproduct-bot
session.
Go ahead and try it out! Add a few products and view them so see your print function in action.
-
Challenge
### Conclusion
You've traversed through the landscape of structures, unions, and dynamic memory allocation to build an inventory management system in C. You've learned to encapsulate data with structures, flexibly store condition data with unions, and manage growth with dynamic memory. This journey has equipped you with the skills to handle complex data models in C programming.
More Advanced Structure Topics
For those looking to deepen your knowledge, consider exploring topics like linked lists for dynamic inventory management, structure padding for performance optimization, or just see how far you can take this program. Can you implement more functionality like the ability to edit products?
Also, it is recommend that you explore the concept of function pointers. Function pointers can give your custom data types behaviors! For example, you could have a function pointer in your Inventory structure for custom printing formats or sorting algorithms, making the system highly adaptable to user needs or different business rules.
Thank you for sticking around to the end of this Guided Code Lab. Please continue learning C by exploring more code labs and video course here, at Pluralsight.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.