- Lab
- Core Tech
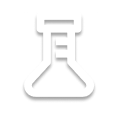
Guided: Understanding Variables and Scope with JavaScript
This guided lab serves to solidify your grasp on the fundamental concepts of variables and scope, which are integral aspects of programming that all developers should be familiar with. Throughout this exercise, you'll leverage your understanding of these foundational principles to construct a straightforward quiz application using JavaScript.
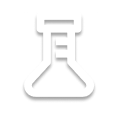
Path Info
Table of Contents
-
Challenge
Introduction
Welcome to the Guided: Understanding Variables and Scope with JavaScript lab.
The utilization of variables and scope within programming is a core concept when developing any application. Nonetheless, it is a fairly straightforward and intuitive concept to understand. You will be working with JavaScript for this lab, but know that the usage of variables and scope applies to every other programming language out there as well.
Once you have an understanding on variables and scope, you will work with JavaScript to complete a simple quiz application webpage.
There is a solution directory that you can refer to if you are stuck or want to check your implementations at any time. Keep in mind that the solution provided in this directory is not the only solution, so it is acceptable for you to have a different solution so long as the application functions as intended.
You can see the results of your code in the Web Browser.
-
Challenge
Variables
Overview
In programming, variables are symbolic names that refer to a memory address that contains information.
For example, say you had a filing cabinet with multiple different folders. One folder might be labelled "Taxes", another might be labelled "Insurance", or a third labelled as "Medical". In this scenario, the folders represent the containers in memory while the files within would represent the data held. Variables represent the labels that we put on these containers.
Just as the files in these folders may or may not change over time, so too does the data within memory, hence the name variable.
Names
As stated previously, variables are names that are attached to pieces of data. This means that there are a few rules they must follow.
- They must be unique in the context they are used. This is because variables are how programmers utilize the data within. Computer programs will spout errors if there are multiple variables with the same name.
- They are case-sensitive. A variable named
example
and a variable namedExample
are two separate variables. - They must start with a letter(or underscore
_
) and cannot contain spaces. They can contain numbers but cannot start with a number. - Generally speaking, they cannot contain special characters. However, some special characters are allowed depending on the language. These typically include the underscore(
_
) and dollar sign$
. Other special characters cannot be used unless explicitly allowed by the language in question. - Naming should be meaningful. This means names like
studentGrades
orfounding_year
are better thandata1
ortest_var
. - They should follow language specific naming conventions. Common naming conventions include PascalCase/UpperCamelCase(
StudentGrades
), lowerCamelCase/dromedaryCase(studentGrades
), and snake_case(student_grades
).
Types
When variables are created, or declared, they must also have a specific type. In certain static typed languages like Java, this means that variables must specify their type when declared, such as
int numberOfShoes = 4; string customerName = "Bob"; boolean isNightTime = false;
Attempting to assign values that are not of the specified type will result in compilation errors. Thinking back to the filing cabinet analogy, you wouldn't want to have test results for students in Grade 11 in the Grade 12 folder or vice versa.
On the other hand, dynamically typed languages like Python or JavaScript do not require you to specify the type of the variable as the compiler can infer based on the value.
// Python numberOfShirts = 6 // integer password = "bacon" // string bananaPrice = 0.99 // float(decimal) isScared = true // boolean // JavaScript var numberOfShirts = 6 // integer const password = "bacon" // string let bananaPrice = 0.99 // float(decimal) let isScared = true // boolean
We'll cover the
var
,const
, andlet
keywords for JavaScript in the next section.
Values
Variables usually have values they are assigned to. Aside from primitive singular values, they can also be custom objects. For instance, a car variable could be
const exampleCar = { make: "Toyota", model: "Camry", modelYear: 2013, isNew: False } // Properties of object can be accessed using the . operator exampleCar.isNew = true; exampleCar.model = "Civic"; exampleCar.make = "Honda";
In some cases, variables can have a value of "nothing", or may not be assigned a value at all. When it's value is "nothing" this is typically known as
null
. If the variable does not have a value assigned at all, then it isundefined
.int a = null; int b; // undefined as it has been declared without an assigned value
Referring back to our filing cabinet analogy, a
null
variable would be a variable attached to an empty folder. Anundefined
variable is a label that is not even attached to a folder at all, it's simply the label by itself. -
Challenge
Scope
Overview
Scope refers to the region or context in a program where a particular variable can be accessed or modified. It defines the visibility and lifetime of a variable, determining which parts of the code can use the variable and where it is no longer accessible.
It helps in avoiding naming conflicts and ensures that variables are used appropriately within their intended context.
Types of Scope
The hierarchy of scope, or contexts, are global scope, local scope, and block scope .
Variables that are block scoped are variables that are valid within a certain block of code, which is defined as a set of statements within a set of curly braces
{}
. This can include loops or if/if-else statements. Once these statements end, any variables declared within these blocks are no longer valid. This means that block scope is the lowest level of context, as variables defined here can go out of scope within a function.Variables that are local scoped are variables that are valid within an entire function. This means that any blocks of code within the function, such as the aforementioned loops or if statements, can utilize these variables without having to declare them. When these blocks finish and go out of scope, the variables will still be valid for reference until the function itself ends.
Lastly, variables that are global scoped means they are defined in the program outside of any function. This means that they can be used and referred to by any functions, blocks, and other global variables. They only go out of scope once the program itself finishes.
Keywords and Examples
As you saw before, defining JavaScript variables utilizes 3 possible keywords:
var
,let
, andconst
. The first keywordvar
was introduced since JavaScripts conception. Variables declared with this keyword are typically local scoped and reassignable. Reassignable means you can redeclare a variable with the same name, and instead of having duplicates the compiler will simply overwrite the previous value with this new value.var test = 0; var test = "hello" // Valid because there aren't actually // two variables with the same name, // the `test` variable has just been // reassigned to "hello"
The
let
andconst
keywords were introduced in ES6, a JavaScript standard that released in 2015. Both of these keywords are considered block scoped and are not reassignable. However,let
variables can still have their values changed whileconst
variables cannot have their values changed(unless they refer to objects).let anotherVar = "hello"; const secondVar= 15 const listOfNumbers = [5, 10, 15, 20]; let anotherVar = 12.2 // invalid, anotherVar has already been declared anotherVar = 12.2 // valid const secondVar = "test" // invalid, secondVar has already been declared secondVar = 13 // invalid, const cannot have its value changed listOfNumbers.pop() // [5, 10, 15] // valid, even though it is a const // the array itself is not being changed, just the elements within. // This also applies to objects.
Furthermore, scopes are not necessary mutually exclusive. For instance, all three keywords can be used when defining globally scoped variables regardless of the typical scopes that they are typically used for.
// All 3 are global variables const globalConst = 5 let globalLet = 2 var globalVar = 8 function exampleFunction() { }
Lastly, here is an in-depth example regarding how separate scopes may interact.
const globalConst = "globalConst" let globalLet = 2 var globalVar = 3 function exampleFunction() { globalVar = 4 // valid const globalConst = "localConst" // invalid, global variables exist in function context globalConst = "localConst" // invalid let globalLet = "localLet" // also invalid globalLet = "localLet" // valid if(true) { // new block scope is made console.log(globalVar); // 4 globalVar = 5; console.log(globalVar); // 5 var localVar = "exists"; let exampleLet = "does not exist" const exampleConst = "does not exist" let globalLet = "blockLet" // valid, globalLet is declared in a new context console.log(globalLet) // "blockLet" const globalConst = "blockConst"; // valid console.log(globalConst); // "blockConst" } // scope ends console.log(globalLet); // "localLet", "blockLet" has gone out of scope console.log(globalConst); // "globalConst" console.log(localVar); // "exists", var is function wide even if declared in a block console.log(exampleLet) // invalid, does not exist anymore console.log(exampleConst) // invalid, does not exist anymore } //function ends console.log(localVar) // invalid, does not exist out of function console.log(globalLet) // "localLet", has been changed by executing the function console.log(globalConst) // "globalConst"
-
Challenge
Quiz Application
Overview
Now you will work on the JavaScript quiz application. You will be working on
js-quiz.js
and you will be able to see your results in the Web Browser. There are comments injs-quiz.js
that tell you where to start and end.For troubleshooting purposes, it is highly recommended to open the web browser in a new browser tab. Then, right-click the page and select "Inspect". Then navigate to the "Console" tab in the resulting panel. Errors in your code with the respective line numbers will be reported here. If you see the message
Failed to load resource: the server responded with a status of 404 ()
you can ignore it.Otherwise, you can refer to the solution file in the solution directory.
Defining Global Variables
First, you will need to define the necessary global variables in
js-quiz.js
.There are 4 HTML elements that need to be stored in global variables.
Instructions
1. Use `document.getElementById(elementID)` to retrieve each element. The `elementID`s are `'quiz-container'`, `'question-container'`, `'choices-container'`, and `start-button'`. 2. Store each element in a variable with an appropriate name. 3. An example would be `const quizContainer = document.getElementById('quiz-container')`.Next, you will want to shuffle all the quiz questions.
Instructions
1. Use the `shuffle(input)` function to shuffle `allQuestions`. 2. Store this result in a variable called `quizQuestions`.Lastly, you will want to define two variables that keep track of quiz score and the current question.
Instructions
1. Create a variable called `score` and a variable called `currentQuestionIndex`. Use the `let` keyword as their values will change. 2. Set them to `0`.
Implementing Functions
Now you will need to incorporate your variables into 4 key functions.
The first function to complete is
displayQuestion()
. This function has aquestionIndex
parameter, which you will use to retrieve a question from yourquizQuestions
variable. This function is necessary for displaying the current question at hand and the possible answer choices.Instructions
1. Declare a variable called `currentQuestion`. Assign it to the element at `questionIndex` in `quizQuestions`. 2. You can retrieve the an element from an array using `arrayName[index]`. 3. Declare a second variable called `currentAnswers`. This variable should contain the randomized answer choices for the `currentQuestion`. Do this by performing `shuffle()` on `currentQuestion.choices`.Next is
startQuiz()
, which will be called when clicking on theStart Quiz
button.Instructions
1. First, set `startButton.style.visibility` to `"hidden"`. 2. Then call `displayQuestion()` passing in your `currentQuestionIndex` global variable as the parameter.Next, you'll need to setup the variables for
checkAnswer
to determine whether the selected answer was correct.Instructions
1. At the start of `checkAnswer`, declare a variable called `currentQuestion`. Like in `displayQuestion()`, use the `questionIndex` parameter to retrieve the element from `quizQuestions` and store it in `currentQuestion`. 2. Declare a second variable called `correctAnswer`. This variable should contain the `correctAnswer` property of `currentQuestion`. Remember to use the `.` operator to access properties of an object.Lastly, you will need to complete
nextQuestion()
.Instructions
1. At the start, increment `currentQuestionIndex`. Options to do this include `currentQuestionIndex++`, or `currentQuestionIndex += 1`, or `currentQuestionIndex = currentQuestionIndex + 1`. 2. After the `choicesContainer.classList.remove()` statement, create an `if-else` statement. 3. The condition to check in the `if` statement is whether `currentQuestionIndex < quizQuestions.length` If it is, use `displayQuestion()` with the `currentQuestionIndex`. 4. In the `else` statement, call `endQuiz()`.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.