- Lab
- Core Tech
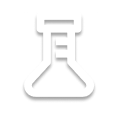
Guided: Searching and Sorting in a Java Book Catalog App
This hands-on lab is designed to help you practice your understanding of fundamental searching and sorting algorithms in Java. A series of guided steps will give you a walkthrough on how to use these sorting and searching algorithms to optimize the time and resources needed to search for and optimize a set of data within a Java environment. By the end of the lab, you will have demonstrated your ability to build a functioning Java book catalog application that uses these algorithms to efficiently manage its data.
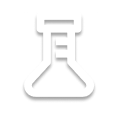
Path Info
Table of Contents
-
Challenge
Introduction
You have been given a mostly complete Book Catalog Application in Java. In this lab, you will be responsible for finishing up the rest of the application by implementing algorithms such as quick sort, merge sort, and binary search. These algorithms are a must-know for any developer as they provide efficient ways for ordering or retrieving data in progressively larger data sets.
There are two main classes to take note of. First is in
Book.java
, which contains an implementation for theBook
objects that are stored in the catalog. It has already been completed for you and is fairly straightforward. Take note of itscompareTo
method which compares books by title for alphabetical ordering.Second is the
Catalog.java
file which contains theCatalog
class. This implementation is mostly complete with the exception of a few functions you will need to complete to finish the implementation.The app can be launched at any time by clicking the Run button at the bottom right of the Terminal.
-
Challenge
Sorting Algorithm: Merge Sort
The first of the two sorting algorithms you will need to implement is a merge sort. The idea behind a merge sort involves continuously splitting a list in half until it can no longer be divided (meaning either the list is empty or the split sublist only contains one element). Then, you sort each sublist before merging the sublists together until eventually you have reformed the original list in sorted form.
To do this, you will also need a secondary
merge()
function for your merge sort to combine the sublists. The merge sort function itself then becomes a recursive call to each of its halves before calling themerge()
function.Details
- Create three index variables:
i
forleft
,j
forright
, andk
foroutput
. Initialize them to 0. - Start a
while
loop that continues as long as bothi
is less thanleft.size()
andj
is less thanright.size()
. - Inside the loop, compare the elements at indices
i
andj
inleft
andright
, respectively. - If the element at
left[i]
is less than or equal to the element atright[j]
( See Hint #1):- Assign the element at
left[i]
tooutput[k]
(See Hint #2). - Increment both
i
andk
.
- Assign the element at
- Otherwise, if the element at
left[i]
is greater than the element atright[j]
:- Assign the element at
right[j]
tooutput[k]
. - Increment both
j
andk
.
- Assign the element at
- After the while loop, add any remaining elements from
left
tooutput
using another while loop. - After the while loop, add any remaining elements from
right
tooutput
using another while loop.
Hint #1
Use the
Book
classescompareTo
to compare books. A value of 0 or less should be placed in theoutput
array first.Hint #2
Hint: The
set(index, value)
method of theList
class can be used for placing elements in theoutput
list. Theget(index)
method can be used to retrieve values from a specified index.Details
- Check if the size of
arr
is less than 2. If so,return
since the list is already sorted or empty. - Calculate the middle index of
arr
. You can do this by dividing the size of the list by 2 and rounding down if necessary. Let's call this indexmi
. - Create two new lists,
list1
andlist2
. - Put all elements from index 0 to
mi
(exclusive) inlist1
. - Put all elements from
mi
to the end ofarr
inlist2
. - Call
mergeSort
recursively onlist1
. - Call
mergeSort
recursively onlist2
. - Call the
merge
function witharr
,list1
, andlist2
to merge the two sorted lists back intoarr
.
- Create three index variables:
-
Challenge
Sorting Algorithm: Quick Sort
The second sorting algorithm you will be implementing here will be a quick sort. Like merge sort, quick sort is also a divide and conquer algorithm that involves dividing the input data into smaller sublists, sorting them individually and then combining them. The quick sort process involves picking a "pivot" element, usually the first or last element of the list, and splitting the list such that all smaller elements come before the pivot, all greater elements come after, and the pivot itself is placed in its correct final position.
To do this, you will once again need an auxiliary function called
partition()
, which has already been defined for you. Another function calledswap()
for swapping elements has already been provided for you for use inpartition()
.Details
- Select the pivot element from the end of
arr
(book at indexhigh
). - Create a variable, such as
ci
, and set it tolow - 1
. - Start a loop from
low
tohigh - 1
. - Inside the loop, if
arr[x]
is less than the pivot, incrementci
andswap
arr[ci]
witharr[x]
. - After the loop,
swap
arr[ci + 1]
witharr[high]
. - Return
ci + 1
as the updated index of the pivot
Details
- Check if
low
is less thanhigh
. If not, return since the current partition has zero or one element. - Call the
partition
function and store its return value in a variable, such aspivotIndex
. - Recursively call
quickSort
witharr
,low
, andpivotIndex - 1
as parameters. - Recursively call
quickSort
witharr
,pivotIndex + 1
, andhigh
as parameters.
- Select the pivot element from the end of
-
Challenge
Search Algorithm: Binary Search
The final step will be for you to implement a search algorithm known as binary search. For larger data sets, binary search offers much better performance compared to a linear search when retrieving data. However, it can only be performed on sorted data sets (which makes the previous two algorithms come in handy!).
Like the previous algorithms, binary search is also a divide and conquer algorithm. It involves selecting an element from the middle of the array and comparing it to the element to be retrieved. If the target element is less, then binary search is performed again from start to the mid element. If the target element was more, then binary search is performed from the midpoint to the end. This continues until a match is found or is not found in the list.
Details
- Set a
start
as the first index of the list. - Set an
end
as the last index of the list. - While
start
is less than or equal toend
, do steps 6-9. - Calculate
mid
as theaverage
ofstart
andend
. - If the value at index
mid
is equal to the target value, returnmid
. - If the value at index
mid
is greater than the target value, setend
tomid - 1
. - If the value at index
mid
is less than the target value, setstart
tomid + 1
. - Return
-1
outside of thewhile
loop
- Set a
-
Challenge
Epilogue
Great job on completing the lab. Now you should be familiar with search and sort algorithms such as merge sort, quick sort, and binary search. These algorithms, along with other search and sort algorithms, are very important for maintaining performance in terms of resources and time when working with progressively larger data sets.
The use cases for search and sort algorithms are also fairly specific to the scenario, as some algorithms perform significantly better than others under certain circumstances while others could perform significantly worse than they typically would. It's your job as the developer to assess the situation when determining the best approach!
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.