- Lab
- Core Tech
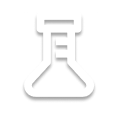
Guided: Remix Foundations
Remix is a JavaScript Metaframework that aspires to provide the responsiveness of reactive web applications without the complex architecture that such applications often must contend with to manage application state and communicate with the server. This guided lab is designed to allow you to build your own Remix application and help you decide if it is a good fit for your next project.
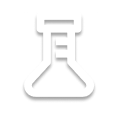
Path Info
Table of Contents
-
Challenge
Introduction
Welcome to the lab Guided: Remix Foundations.
In this lab, you will complete the implementation of a Remix application that tracks the daily runs of an amateur athlete. This application allows runners to view, add, edit, and delete runs from their training log. You will start with several pages and supporting code modules provided to you to get you going. You will then finish the implementation of the remaining pages while practicing Remix's core lifecycle - load -> render -> action.
To complete this lab, you will need to do the following:
-
Implement the detail page. This page will show the details of an individual run and requires two changes:
- Finish the
loader
function that will make the run details available to the page - Populate the page with the requested run's data
- Finish the
-
Implement the edit page. This page will be used to update the details of a specific run. It needs the following updates:
- Update a
<Form />
on the detail page to navigate to the edit page - Update the
loader
function to retrieve the run data - Use the run data to prefill the edit form's fields
- Implement an
action
that will save the updated run
- Update a
-
Implement the delete action.
- Update a
<Form />
on the detail page to navigate to trigger the delete action - Implement the
action
that will delete the requested run
While you are working on the application, you can view your progress by using the
Run
button in the lower right-hand corner of the screen. This will start a development server that will run your program and automatically update when you make any changes. You can view the application by opening a browser window to {{localhost:5173}}. - Update a
-
-
Challenge
Complete the Detail Page
The detail page's source code can be found in
/app/routes/runs_.$activeMonday.run.@id.tsx
. The file name is unusually long since it contains the route information that Remix uses to map URL paths to specific pages. In this case, the file name correlates to the route/runs/:activeMonday/run/:id
where "activeMonday" and "id" are parameters that provide specific information about what data should be shown on the page. TheactiveMonday
parameter is used by the sidebar menu to determine which week is currently active and theid
parameter specifies which specific run is being viewed.Your first task on this page is to retrieve the current run data and make that available to the page. In Remix, this work is done by a special function called
loader
. This function runs on the server side and is used to provide data to the client page or module for rendering. The run data is provided to the page via theuseLoaderData
function. Now that theloader
is feeding this data from the server to the client you can show that data to the user. You can find the correct field names by examining theRun
type in the/app/data.ts
file. -
Challenge
Complete the Edit Page
The edit page is used to allow a runner to change the details of a specific run such as updating mileage or their average pace. You will update the
loader
and HTML sections of this page to pre-populate it with the current details. Saving changes, however, will require anaction
that will present the new details to the server which will then make the required updates. Before you get into those changes, you need to enable the navigation from the detail page to the edit page. The next few steps are almost identical to changes that you have already made on the detail page. These, however, will be done on the edit page, located at/app/routes/runs_.$activeMonday.run.$id_.edit.tsx
. Now you will need to connect the edit page's<Form/>
to the server. This is done in two steps. Remix uses a<Form/>
action to determine how to handle the submission of a form. By default, forms submit their data using a GET request. In Remix, this will trigger a navigation event, but will not fire andactions
. When the method is set to POST, however, Remix will look for anyaction
functions and call those. These are normally used to trigger changes on the server based on what the user submitted. After theaction
updates the server, the application needs to be told where to go next. By default, the user will stay on the edit page. It is more common, however, to send the user back to a view-only page after they are done with edits. You will use aredirect
to accomplish this. -
Challenge
Implement the Destroy Action
That final feature that the application needs is the ability to delete a run. Since there is no specific view for this activity, you will use a standalone action to implement this behavior. The Delete form will now send a POST request to the URL path
/runs/:activeMonday/run/:id/destroy
. This will call anyactions
on that page which is where you will implement the logic to delete the specified run. After theaction
updates the server, the application needs to be told where to go next. By default, the user would stay on the delete page, but there is no HTML on this page to show them. You also can't redirect the user to the detail page, since that was just deleted. Instead, you will redirect the user to the current week's overview page. -
Challenge
Conclusion
Congratulations on completing this lab!
Throughout this lab, you've had a chance to experiment with some of the key features that Remix offers including:
- Full-stack web development without constant context switching between the client and server
- The responsiveness of a single-page application combined with the straight forward forms-based state management system.
- Nested routing system that allows page components to be tightly bound with server-side data and action.
Feel free to continue to experiment in the lab environment to discover more about Remix. Remember that the {{localhost:5173}} link can be used to view the application as it develops.
Links
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.