- Lab
- Core Tech
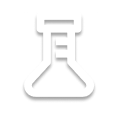
Guided: Playwright Foundations with Node.js
Unlock the full potential of end-to-end testing with Playwright and Node.js in this comprehensive, Guided Code Lab. You'll learn to perform critical tests on an e-commerce website, covering user registration, login, and shopping cart functionalities. By the end of this lab, you'll have hands-on experience with Playwright's powerful features and be well-equipped to ensure your web applications run flawlessly.
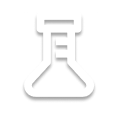
Path Info
Table of Contents
-
Challenge
Introduction
Welcome to the Guided: Playwright Foundations with Node.js Code Lab!
Playwright is a framework that enables reliable end-to-end testing and automation for modern web applications.
info> The prerequisites for this lab include familiarity with JavaScript and HTML.
Playwright Test was created specifically to accommodate the needs of end-to-end testing, and it supports all modern rendering engines including Chromium, WebKit, Firefox, and mobile browser engines.
In this Code Lab, you will become a QA engineer tasked with verifying the functionality of a prototype e-commerce site for ABC Mobile Carrier. Starting from the ground up, you'll:
-
Write and run tests for user registration, including validation checks.
-
Implement tests for user login/registration.
-
Perform actions such as filling out forms, clicking buttons, and verifying success messages.
-
Ensure that your tests cover all necessary validation and business logic.
Completing these tasks will give you practical experience with Playwright's robust testing framework and learn to write reliable, maintainable end-to-end tests.
The files for the ABC Mobile Carrier website can be found under the
src/public
folder.You will write the code for this project under the
src/apptests
folder, where you will find the following files:
The `src/apptests/index.spec.js` file is where you will write the code for testing the functionality of `src/public/index.html`.index.spec.js
Test command:npx playwright test src/apptests/index.spec.js
The ```src/apptests/login.spec.js``` file is where you will write the code for testing the functionality of the ```src/public/login.html``` and ```src/public/login.js``` files.login.spec.js
Test command:npx playwright test src/apptests/login.spec.js
The ```src/apptests/register.spec.js``` file is where you will write the code for testing the functionality of the ```src/public/register.html``` and ```src/public/register.js``` files.register.spec.js
Test command:npx playwright test src/apptests/register.spec.js
The
catalog.spec.js
files includes the finished testing code forcatalog.html
, as an example.Before anything, please familiarize yourself with the ABC Mobile Carrier website, which is accessible by running the
node src/index.js
command from the Terminal, and refreshing the Web Browser tab, You may click on the Open in new browser tab button on the Web Browser address bar, to view the site in your browser.Play with the ABC Mobile Carrier website and see how it works.
info>The solution for each step in this lab are available for your reference in the
solution
directory.
Each step might have one or more tasks. For example, the solution for Step 2 - Task 1 can be found in thesolution/step02/task01.spec.js
file.
Within thesolution/final
folder, you will find all of the finished project files.
You may also test the finished code using thenpx playwright test
command, which will run all of the tests. If you want to run a specific project, you can specify it at the end of the command. For example:npx playwright test solution/final/index.spec.js
-
-
Challenge
Creating Index Testing
In this step, you will create the foundation for the Playwright tests and automations for validating the logic of the
src/public/index.html
file, which is a fundamental part of creating the Playwright tests for the whole solution.You will write the Playwright tests and automations for testing in the
index.spec.js
file located in thesrc/apptests
directory.First, you will need to import the required Playwright functions and describe the test suite for the ABC Mobile Carrier Homepage to ensure that you will be indeed testing the
src/public/index.html
file. To test the functionality ofsrc/public/index.html
, it is essential for Playwright to navigate to the location of the page, by accessing thehttp://localhost:3000/index.html
URL.Once you have accessed the URL using Playwright, you can verify that the
index.html
page has the expected title:'ABC Mobile Carrier'
.The
src/public/index.html
file also contains anh1
tag, which includes the'Welcome to ABC Mobile Carrier'
text. You'll also have to check that this text is included. Next, you need to check if the Register button is present and has correct attributes. When you click on the Register button, you should be redirected toregister.html
. You will also need to check if the Login button is present and has correct attributes. By clicking on the Login button, you should be redirected tologin.html
. -
Challenge
Expanding Index Testing
Continuing with the testing of the
src/public/index.html
file, you must check if by clicking the Register and Login buttons, theindex.html
page redirects toregister.html
andlogin.html
, respectively.This is crucially important because it checks whether if the URLs match those respective
register.html
andlogin.html
pages.Therefore, throughout this step, you will continue to expand the
src/apptests/index.spec.js
file and add additional logic to it. After checking that the URLs matchregister.html
andlogin.html
, you must now create a test to verify if the Bootstrap and custom stylesheets are linked correctly. -
Challenge
Creating Login Testing
Now that you have written all the logic required for testing
src/public/index.html
, it is now time to put some of this gained knowledge for creating the tests for the Login functionality. You'll write these tests inlogin.spec.js
located in thesrc/apptests
directory.First, navigate to
login.html
and check for the page title. Next, you will write test code for checking all the fields to be filled. Now, you will write test code for checking that the Login page shows an error message for invalid credentials. Now, you will test that thelogin.html
page should log in successfully with valid credentials. To wrap up the tests for thelogin.html
page, your next test should validate that the user is navigated tocatalog.html
when clicking the success link. -
Challenge
Creating Register Testing
You are now ready to validate the
register.html
page by writing tests in theregister.spec.js
file.You must first test the display of the registration form with the correct fields.
This test verifies that all the required input fields are present and visible on the registration form. It also checks that the labels associated with these fields have the correct text. Next, you must ensure that when the registration form is submitted with empty fields, validation error messages are displayed for each required field, indicating that they need to be filled in. Next, you must verify that the form displays appropriate validation error messages when invalid data is entered into the fields, ensuring that users are informed of input mistakes.
-
Challenge
Expanding Register Testing
Moving on, you'll now explore how to create a test to successfully register with a valid input and display a success message.
This test ensures that the form can be successfully submitted with valid inputs, displaying a success message, and confirming the presence of a link to navigate to the catalog page.
There's one final test to perform. This test confirms that after a successful registration, clicking the link in the success message navigates the user to the catalog page, verifying the link's functionality. Congratulations! You can now check to see if all of your tests pass correctly:
- Ensure the application is running locally on localhost, by running the
node src/index.js
command. - Run the tests using
npx playwright test
. - Debug any issues by running tests with the
--headed
flag. - Review the results! Once the tests pass, you should see output in the terminal showing that all tests have passed successfully. If everything works as expected, your test cases are complete.
By following these steps, you'll be able to ensure your Playwright tests are correctly implemented and verify that all of them pass.
- Ensure the application is running locally on localhost, by running the
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.