- Lab
- Core Tech
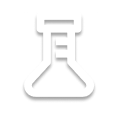
Guided: Leveraging Go Modules for Project Management
Dive into this Code Lab to master Go Modules and elevate your Go project management skills. Discover how to efficiently manage dependencies, version your modules, and ensure consistency with go.mod and go.sum files. Through hands-on exercises, you'll learn how to handle real-world challenges, such as integrating and updating dependencies, and employing vendoring for offline scenarios. Perfect for developers seeking to enhance their Go project workflows and maintain a clean, reliable codebase.
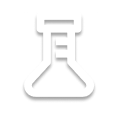
Path Info
Table of Contents
-
Challenge
Introduction
Embark on a journey to master Go Modules for seamless project management. Starting with the basics of package management, you'll gradually advance to harness the full potential of Go Modules for project organization, dependency management, and vendoring.
Throughout the lab, you'll work through the following topics:
Introduction to Go Modules
Understand the fundamentals of Go Modules and their importance in modern Go development.Creating and Managing Projects with Go Modules
Dive into practical exercises to create new projects using Go Modules and explore best practices for project organization.Versioning and Dependency Management
Learn to version your modules and manage dependencies efficiently, ensuring project stability.Vendoring
Discover how to use vendoring to include dependencies directly in your project, enhancing reproducibility and offline access.By the end of this lab, you'll emerge with a comprehensive understanding of Go Modules, equipped to streamline your project management workflow and tackle complex projects with confidence.
-
Challenge
Go Modules
Go modules provide a powerful and flexible way to manage dependencies, ensuring that your projects are reliable, reproducible, and maintainable.
A Go module is a collection of related Go packages that are versioned together as a unit. Modules are defined by a
go.mod
file located at the root of the module's directory. This file specifies the module's path and the versions of dependencies it requires.Go Modules were introduced in Go 1.11 as a solution to the challenges of dependency management and versioning. Prior to Go Modules, dependency management in Go was handled using tools like
dep
andGOPATH
, which had limitations, particularly in terms of reproducibility and handling multiple versions of dependencies.Benefits of Using Go Modules
Go Modules offer several advantages over the previous GOPATH-based approach:
- Versioning : Modules allow you to specify exact versions of dependencies, ensuring that your project builds reproducibly.
- Dependency Isolation : Each module has its own set of dependencies, which eliminates conflicts that could arise from shared dependencies in GOPATH.
- Support for Multiple Modules : Go Modules enable you to work on multiple projects, each with its own dependencies, without the need for complex environment management.
- Easy Publishing : Modules make it straightforward to publish your Go packages and manage their versions.
Initilizing a Go Module
You can initialize a module using the
go mod init <module_path>
command, Go creates ago.mod
file in your project directory. This file will track the module's dependencies and their versions. When you build your project, Go will automatically download the necessary dependencies and store them in a local cache, making subsequent builds faster.After initializing a module, a
go.mod
file is created. In the following step, you'll learn more about this file. -
Challenge
Understanding the `go.mod` File
When managing a Go project, it's useful to understand the
go.mod
andgo.sum
files. These files help ensure that your project’s dependencies are consistent and well-managed, making your Go projects reproducible and easier to maintain.The
go.mod
FileThe
go.mod
file defines the module’s properties, including its module path, dependencies, and the Go version required. It is typically located at the root of your project and is automatically created when you run theinit
command.
Key Components of the
go.mod
File:1. Module Declaration
The first line of the
go.mod
file specifies the module’s path, typically the root URL of your repository or a custom path:module github.com/user/mymodule
2. Go Version
Specifies the version of Go that the module is compatible with:
go 1.19
3. Require Directive
Lists the dependencies of your module and their versions:
require ( github.com/dustin/go-humanize v1.0.1 rsc.io/quote v1.5.2 )
4. Replace Directive
Allows you to replace a dependency’s module path with a different module, which can be useful for testing local changes:
replace example.com/dependency => ../local_dependency
5. Exclude Directive
Prevents a specific version of a module from being used in your project:
exclude example.com/dependency v1.4.0
Next, you will add the
rsc.io/quote
of versionv1.5.2
dependency to your Go project.info> Since internet connectivity is restricted in this lab environment, you will reference dependencies from a local directory instead of downloading them from the internet.
Use the
replace
directive to point the dependency to a local folder. Now that you've set the settings for thersc.io
module to load from local directory, you can add this dependency in your project. Great! You've successfully added thersc.io/quote
dependency to your project.
In Go, the
go get
command offers several methods to manage dependencies:1. Fetching a Specific Version: Use
go get github.com/some/package@v1.1.0
to fetch a specific version.2. Fetching the Latest Version: Run
go get github.com/some/package
to get the latest release of a package.3. Fetching a Specific Commit: Use
go get github.com/some/package@<commit-hash>
to get a specific commit.These commands update the
go.mod
file with the dependency information and thego.sum
file with the checksum, which you'll learn more about in the following steps. After adding the dependency, if you check thego.mod
file, you will notice some additional dependencies that were not added by you. In the next step, you'll learn about transitive dependencies. -
Challenge
Transitive Dependencies
Transitive dependencies are libraries that your direct dependencies rely on. For instance, if your project uses package
A
, and packageA
depends on packageB
, then packageB
is a transitive dependency.The
go.mod
File Contents:module github.com/user/mymodule go 1.19 require ( golang.org/x/text v0.0.0-20170915032832-14c0d48ead0c // indirect rsc.io/quote v1.5.2 // indirect rsc.io/sampler v1.3.0 // indirect )
In Go, a dependency is marked with
// indirect
in thego.mod
file for two primary reasons:1. Transitive Dependency: The dependency is required by another dependency, not directly by your code. It gets included automatically to ensure the entire dependency chain is satisfied, even though your code doesn't import it directly.
For example, when you added the
rsc.io/quote
dependency, thersc.io/sampler
andgolang.org/x/text
dependencies were automatically added as well.2. First-Time Addition: When you first add a dependency using
go get
, but haven't yet used it in your code, Go marks it as// indirect
, indicating the dependency is included but not directly referenced.For example, the
rsc.io/quote
dependency has this comment because it isn't currently used anywhere in the project.Next, you will update the
main.go
file to includersc.io/quote
and utilize one of its functions. ---The
go mod why
Commandgo mod why
is a Go command that provides insight into why a specific package is included as a dependency in your project. It helps you trace the chain of imports that brought a particular package into your module, making it easier to understand your project's dependency tree and manage it effectively.Key Features:
Tracing Dependencies: The primary use of the
go mod why
command is to reveal the path of dependencies that lead to the inclusion of a specific package. This is particularly useful when you see a package listed in yourgo.mod
orgo.sum
files and want to know why it is there.Simplifying Debugging: If your project has unexpected or unnecessary dependencies,
go mod why
can help you determine where they are coming from. This makes it easier to clean up your dependencies and remove any that are no longer needed.Understanding Indirect Dependencies: Sometimes, your code does not directly import a package, but one of your direct dependencies does. The
go mod why
command will show you how such indirect dependencies are linked to your project.Now, you will try to find the dependency information for the
rsc.io/sampler
package usinggo mod why
. In this case,rsc.io/sampler
is included in your project because it's a dependency ofrsc.io/quote
, which you directly imported in your project.
Having used the
rsc.io/quote
package, you will now run thego mod tidy
command and observe the changes in thego.mod
file. After running thego mod tidy
command, the// indirect
comment was removed from thersc.io/quote
package because Go identified its usage in the relevant files and updated the comment accordingly.module github.com/user/mymodule go 1.19 require rsc.io/quote v1.5.2 require ( golang.org/x/text v0.0.0-20170915032832-14c0d48ead0c // indirect rsc.io/sampler v1.3.0 // indirect )
Similarly, if you add a dependency that is not used, Go will automatically remove it from the
go.mod
file once you run the commandgo mod tidy
. In the next step, you'll learn about thego.sum
file and how it helps in ensuring the integrity and consistency of your project's dependencies. -
Challenge
Understanding the `go.sum` File
The
go.sum
file is an automatically generated file that contains the checksums (cryptographic hashes) of the exact module versions your project depends on. It ensures that the code you download hasn’t been tampered with and is consistent across builds.The
go.sum
File Contents :golang.org/x/text v0.0.0-20170915032832-14c0d48ead0c h1:qgOY6WgZOaTkIIMiVjBQcw93ERBE4m30iBm00nkL0i8= golang.org/x/text v0.0.0-20170915032832-14c0d48ead0c/go.mod h1:NqM8EUOU14njkJ3fqMW+pc6Ldnwhi/IjpwHt7yyuwOQ= rsc.io/quote v1.5.2 h1:w5fcysjrx7yqtD/aO+QwRjYZOKnaM9Uh2b40tElTs3Y= rsc.io/quote v1.5.2/go.mod h1:LzX7hefJvL54yjefDEDHNONDjII0t9xZLPXsUe+TKr0= rsc.io/sampler v1.3.0 h1:7uVkIFmeBqHfdjD+gZwtXXI+RODJ2Wc4O7MPEh/QiW4= rsc.io/sampler v1.3.0/go.mod h1:T1hPZKmBbMNahiBKFy5HrXp6adAjACjK9JXDnKaTXpA=
Key Points About the
go.sum
File:-
Checksum Verification: Each entry in the
go.sum
file is a record of a module version and its hash. The Go toolchain uses these checksums to verify that the modules you download match the versions you expect. -
Managing the
go.sum
File: You don’t typically edit thego.sum
file manually. It is updated automatically when you run commands likego mod tidy
,go get
, orgo build
. -
Reproducible Builds: By locking down the exact versions of dependencies, the
go.sum
file ensures consistent builds across different environments. -
Security: The
go.sum
file helps protect against supply chain attacks by confirming that the modules you use are unchanged from what the original authors intended.
In summary, the
go.sum
file is automatically managed by Go to provide secure, consistent, and reproducible builds by verifying your project’s dependencies.If you encounter issues with corrupted checksums, you can use the
go mod tidy
command. This command will remove any unnecessary dependencies and regenerate thego.sum
file.--- #### Best Practices for Dependency Management
- Use the
go mod tidy
Command Regularly: Clean up unused dependencies to keep yourgo.mod
andgo.sum
files minimal. - Pin Exact Versions: Always specify exact versions of your dependencies to avoid unexpected updates.
- Review Changes in the
go.sum
File: Ensure that changes in thego.sum
file are expected and don’t introduce unintended updates. - Use Replace and Exclude Wisely: These directives are powerful tools for managing dependencies, but should be used with caution to avoid breaking your build. In this step, you learned how the
go.sum
file ensures the integrity of your dependencies by storing checksums. In the next step, you'll learn about versioning in Go.
-
-
Challenge
Versioning in Go
Versioning is a crucial aspect of managing dependencies in any software project. In Go, the module system uses semantic versioning (SemVer) to manage package versions, ensuring that your projects are stable and that updates do not introduce unexpected breaking changes.
Semantic Versioning (SemVer)
Go modules adhere to the principles of semantic versioning, where each version number follows the format
vMAJOR.MINOR.PATCH
. This versioning system provides clear guidelines on how versions should be incremented based on the changes made:- MAJOR version (
v1.0.0
,v2.0.0
, etc.): Incremented when you make incompatible API changes - MINOR version (
v1.1.0
,v1.2.0
, etc.): Incremented when you add functionality in a backward-compatible manner - PATCH version (
v1.0.1
,v1.0.2
, etc.): Incremented when you make backward-compatible bug fixes
Creating and Publishing Versions
When you develop a Go module, you should tag versions in your version control system (typically Git). These tags correspond to the version numbers of your module.
Step 1: Tagging a Version
To tag a version in Git, navigate to your module's root directory and run:
git tag v1.0.0
This tags the current commit as
v1.0.0
.Step 2: Publishing a New Version
When you add new features or fix bugs, you’ll want to create a new version. The process is similar to the initial tagging:
- Make Your Changes: Develop and commit the changes you want to include in the new version.
- Tag the New Version: Based on the nature of the changes (major, minor, or patch), tag a new version:
git tag v1.1.0
- Update the Version in Your
go.mod
File: If you're working on a new major version (e.g., v2.0.0), you need to update your module path in thego.mod
file:
module github.com/username/projectname/v2
For minor or patch versions, the module path remains the same.
Step 3: Releasing a Major Version
In Go, major versions, v2 and above, require a special treatment because Go expects the major version to be included in the module path. This is done by adding
/v2
,/v3
, etc., to the module path in yourgo.mod
file.For example to release v2.0.0, you would update your
go.mod
file:module github.com/username/projectname/v2
You should also tag the release:
git tag v2.0.0
This indicates to Go that the v2.0.0 version is a major update and can introduce breaking changes. ---
Handling Pre-releases
Pre-release versions are versions that are not yet considered stable, such as
v1.0.0-alpha
,v1.0.0-beta
, orv1.0.0-rc1
. These are tagged similarly to regular versions:git tag v1.0.0-beta
To use a pre-release version in your project, use the following command:
go get github.com/username/projectname@v1.0.0-beta
--- Best Practices for Versioning
- Use Semantic Versioning Consistently: Follow SemVer rules strictly to ensure your users understand the impact of upgrading your module.
- Avoid Breaking Changes in Minor/Patch Versions: Breaking changes should only be introduced in major versions.
- Document Changes: Maintain a clear changelog to help users understand what has changed in each version.
- Test Pre-releases: Use pre-release versions (-alpha, -beta, etc.) to test new features and gather feedback before making a stable release.
Versioning in Go is simple but effective with semantic versioning. Properly tagging versions and managing dependencies ensures reliability and ease of upgrades for your Go projects.
--- In the next step, you'll learn about Vendoring, which includes dependencies directly in your repository.
- MAJOR version (
-
Challenge
Vendoring
Vendoring is a method of managing dependencies in Go projects by copying all the external dependency code directly into a
vendor
directory within your project's repository. This approach can be beneficial in scenarios where you need to ensure that your builds are reproducible without internet access or when dependencies are no longer available online. When Go builds your project, it first checks thevendor
directory for dependencies before attempting to download them from their original sources.Vendoring can be particularly useful in the following situations:
- Reproducibility: It ensures that your builds are consistent and don't depend on the availability of external servers.
- Offline Builds: It allows you to build your project without internet access since all dependencies are locally available.
- Dependency Version Control: It ensures that your project uses exact versions of dependencies without relying on external package managers or repositories.
- Compliance and Auditing: Some organizations require all third-party code to be stored in-house, which vendoring facilitates.
Step 1: Enable Vendoring
To enable vendoring in a Go module, use the following command:
go mod vendor
This command will create a
vendor
directory in your project and populate it with all the dependencies listed in yourgo.mod
file.Step 2: Verify Vendoring Setup
You can verify that vendoring is working correctly by building your project with the
-mod=vendor
flag:go build -mod=vendor
This command forces Go to use the dependencies in the
vendor
directory rather than fetching them from the internet.If you update or add a dependency in your
go.mod
file, rungo mod vendor
again to update thevendor
directory.When the
vendor
directory is present, Go automatically gives preference to the vendored dependencies. There’s no need to modify import paths, instead you can simply use the dependencies as usual in your code.
Now, you will vendor the dependencies. Observe the
vendor
directory created after running thego mod vendor
command.Inside the
vendor
directory, you'll find all the dependent modules and amodules.txt
file, which has detailed record of vendored modules and their versions.
You can run the build with dependencies referenced by the
vendor
directory using thego build -mod=vendor
command.This command instructs Go to use the dependencies located in the
vendor
directory. ---Best Practices for Vendoring
- Regularly Update Dependencies: While vendoring requires manual updates, it’s important to periodically update your dependencies to get the latest security patches and features.
- Run
go mod tidy
Before Vendoring: This ensures that you’re only vendoring the dependencies that your project actually uses. - Review Vendor Directory Before Committing: Check the contents of your
vendor
directory before committing to ensure that only necessary files are included.
-
Challenge
Conclusion & Next Steps
Conclusion
In this lab, you've learned how to effectively leverage Go modules for project management. By understanding how to initialize, manage, and version your Go modules, you've ensured that your projects are well-organized, dependencies are efficiently managed, and builds remain consistent across different environments. Go modules provide a powerful framework for maintaining and scaling your projects, allowing you to focus more on development and less on dependency management.
You've learned how to use
go.mod
to define your module's dependencies and howgo.sum
ensures their integrity. You’ve also covered transitive dependencies and their management, as well as dependency management and versioning practices to keep your project stable and predictable.By introducing vendoring, you’ve enhanced your ability to manage dependencies by storing all required modules locally. This approach ensures consistency and reliability, especially in environments with restricted internet access.
By applying these tools and techniques, you can better manage your Go projects, reduce dependency issues, and maintain a clean, organized codebase.
Next Steps
-
Practice with Real Projects: Apply what you’ve learned by managing dependencies and modules in a real Go project. Experiment with creating, updating, and vendoring dependencies to gain hands-on experience.
-
Explore Advanced Topics: Dive deeper into advanced Go module topics, such as module replacement strategies, working with private modules, and optimizing module usage.
-
Stay Updated: Keep up with evolving Go modules and dependency management practices by following Go’s official documentation and community updates.
-
Optimize Build and Test Processes: Delve into optimizing Go builds and tests when using Go modules, including how to reduce build times and manage test dependencies.
Keep practicing, stay updated, and gradually build your expertise in managing Go projects.
Congratulations on completing the lab!
-
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.