- Lab
- Core Tech
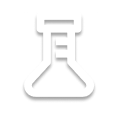
Guided: Implementing Object-oriented Data Management with Pointers in C
Welcome to Implementing Object-oriented Data Management with Pointers in C , a hands-on Code Lab that bridges fundamental programming knowledge with practical application of critical, object orientation, and memory management-related C concepts. Have you ever struggled with managing memory in an object-oriented application? Or wondered how to use pointers in C to provide elegant yet effective access to your data? This lab tackles these challenges by guiding you through building an inventory management system from scratch, using pointers to manage collections of data dynamically. In this Code Lab, you'll implement classes that encapsulate data and behavior, create relationships between objects using pointers, and manage memory allocation and deallocation. You'll learn to avoid common pitfalls like memory leaks and dangling pointers while building a practical system that could be used in real-world applications. By the end of this lab, you'll have a deeper understanding of how objects interact in memory, how pointers can help you with dynamic data management, and how proper encapsulation leads to more maintainable code. These foundational skills are essential for any C developer and will prepare you for more advanced programming challenges.
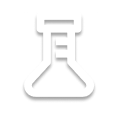
Path Info
Table of Contents
-
Challenge
Introduction
Welcome
In this lab, you'll build a simplified inventory management system that demonstrates core C++ concepts, including object-oriented programming and pointer-based memory management.
The pointer is a fundamental concept in C++ that allows you to manipulate memory directly. Unlike most high-level programming languages, where memory management is handled automatically, C++ gives you direct control through pointers.
This power comes with responsibility - you'll need to carefully manage memory allocation and deallocation to avoid issues like memory leaks.
The starter code provides the basic structure and some partially implemented functions. Your task is to complete the implementation, focusing on proper pointer usage and memory management. The steps in this lab will guide you to successfully complete this exercise.
Tasks can be validated by clicking on the Validate button next to them. A
solution
directory has been provided for reference if you get stuck or would like to compare your implementation. ### Project Structure You'll begin by understanding the overall structure of the inventory management system project. The starter project provides a foundation that you'll build upon throughout this lab.Your inventory system consists of:
- An
InventoryItem
class representing products with properties like ID, name, and quantity - An
InventoryManager
class that maintains a collection of items using pointers - Basic operations, including adding, retrieving, and displaying items
The project is organized into the following folder and file structure:
workspace/
: The root project foldersrc/
: Contains the source files you'll be working onInventoryItem.h
: Header file for theInventoryItem
classInventoryItem.cpp
: Implementation of theInventoryItem
classInventoryManager.h
: Header file for theInventoryManager
classInventoryManager.cpp
: Implementation of theInventoryManager
classmain.cpp
: Main program entry pointbuild/
: Directory for compilation and executable
This structure follows a common pattern for C++ projects:
- Header files (
.h
) define the class interfaces (what methods and properties they have). - Implementation files (
.cpp
) contain the actual code for those methods. - The
main.cpp
file serves as the entry point and demonstrates how to use our classes.
The inventory system is designed to:
- Create and manage inventory items with properties like ID, name, and quantity.
- Store these items in a central manager using pointers.
- Allow operations like adding, retrieving, and displaying items.
Take a moment to navigate through the directory structure to get familiar with it. Throughout this lab, you'll be implementing key functionality in these files to create a working inventory management system. ### Understanding the
InventoryItem
ClassOpen the
InventoryItem.h
file by double-clicking it in the FileTree. You can review its contents in the editor. This header file declares theInventoryItem
class, which represents a product in your inventory.Next, open
InventoryItem.cpp.
This file defines the class’s constructors, getters, anddisplay()
function.Note how this class follows object-oriented principles by encapsulating and hiding data by declaring the corresponding member variables private. The
InventoryItem
class provides public member functions to access and modify these properties. ### Reviewing theInventoryManager
ClassOpen and examine the
InventoryManager.h
andInventoryManager.cpp
files. TheInventoryManager
class is designed to maintain a collection of inventory items, but most of its implementation is missing. Your task in this lab will be to implement this missing functionality.Here are the the key components of the
InventoryManager
class:Member Variables:
items
: An array of pointers toInventoryItem
objectscapacity
: The maximum number of items the manager can holditemCount
: The current number of items in the manager
Member Functions
- Constructor: Initializes the manager with a specified capacity
- Destructor: Cleans up dynamically allocated memory
addItem()
: Creates and adds a new item to the inventorygetItemById()
: Finds and returns an item by its IDdisplayAllItems()
: Shows all items in the inventorysearchByName()
: Looks up an item in the inventory by its nameupdateItem()
: Updates an item's name and quantity at onceremoveItem()
: Removes an item from the inventory
You might notice something unusual in the class's member variables:
InventoryItem** items; // Array of pointers to InventoryItem objects
The double asterisks (
**
) can be confusing if you're new to C++. Here is a breakdown:Single Pointer (
InventoryItem*
): A variable that stores the memory address of anInventoryItem
object. For example:InventoryItem* singleItem
points to one inventory item.Double Pointer (
InventoryItem**
) In your case, this creates an array of pointers.items
points to the beginning of an array- Each element in this array is itself a pointer to an
InventoryItem
When you create the manager with a capacity of 10:
// Create an inventory manager with capacity for 10 items InventoryManager manager(10);
Behind the scenes, the
InventoryManager
constructor gets called, which allocates memory for an array of 10 pointers:InventoryItem** items = new InventoryItem*[10];
This approach gives you significant flexibility:
- You can create new
InventoryItem
objects dynamically with the new operator. - Each item exists independently in memory.
- You can add, access, and remove items individually.
- The manager takes ownership of all these objects, and it's responsible for their deletion.
Look at the
InventoryManager.cpp
file to see that most methods haveTODO
comments. You'll implement these functions to make the inventory manager fully functional while ensuring proper memory management. ### The Program FlowNow, double-click
main.cpp
in the FileTree to examine its contents.This exercise will help you understand how the different components work together and what functionality you need to implement in the
InventoryManager
class.Here's what the main program does:
- Creates an
InventoryManager
with a capacity of 10 items - Adds three items to the inventory (a keyboard, mouse, and monitor)
- Displays all items in the inventory
- Retrieves an item by its ID (102) and updates its quantity
- Looks up an item by its name ("Mouse")
- Updates an item's (id 103) name and quantity
- Removes an item from the inventory (101)
- Displays the updated inventory to verify the changes
The program uses the
InventoryManager
member functions you need to implement. Currently, the program will display error messages or placeholder output.As you complete each function in the
InventoryManager
class, you'll be able to run the program again to test if your implementation works correctly. Your goal is to make all operations inmain.cpp
work properly - adding items, retrieving them, updating them, and removing them from the inventory. ### Building and Running the CodeYou can compile and run the program at any point during the lab by pressing the Run button in the lower-right corner of the Terminal panel.
Alternatively, you can build the code using the
make -C src
command. TheMakefile
is included in thesrc
folder. You'll notice the program compiles, but has limited functionality. Throughout this lab, you'll implement the missing pieces to create a fully functional inventory management system. - An
-
Challenge
Adding Basic Functionality
Implementing the Destructor
Memory management is a critical aspect of C++ programming. The
InventoryManager
class allocates memory dynamically in its constructor, so you need to ensure this memory is properly released when the manager is destroyed.Start by implementing the
destructor
. OpenInventoryManager.cpp
and locate the destructor:// Destructor InventoryManager::~InventoryManager() { // TODO: Clean up dynamically allocated memory // 1. Delete each InventoryItem object // 2. Delete the array of pointers }
The destructor needs to:
- Delete each
InventoryItem
object that was dynamically allocated using thenew
operator. - Delete the array of pointers itself.
When working with dynamically allocated memory, you must ensure that:
- Every object created with
new
is deleted withdelete
. - Every array created with
new[]
is deleted withdelete[]
. - You avoid deleting
nullptr
values (although deletingnullptr
is safe in C++).
You will now implement the destructor.
Implementing the
addItem
FunctionFind the
addItem
function inInventoryManager.cpp
. In the next task, you will add the missing implementation. ### Complete thegetItemById
FunctionLocate the
getItemById
function inInventoryManager.cpp
and add the missing functionality. ### Complete thedisplayAllItems
FunctionFind the
displayAllItems
function inInventoryManager.cpp
and add the missing functionality. ### Build and Run the ProgramNow that you've implemented all the required functionality for the
InventoryManager
class, build and run the program to see it in action. To see how your changes affect the system's behavior, click Run in the lower-right corner of the Terminal. Alternatively, execute the following commands:make -C src src/build/inventory_system ``` Congratulations on completing all the tasks for implementing the basic functionality of the `InventoryManager` class! You've successfully: 1. Implemented the destructor to prevent memory leaks 2. Implemented the `addItem` method to create and add new items to the inventory 3. Implemented the `getItemById` method to find and return items by their ID 4. Implemented the `displayAllItems` method to show all items in the inventory 5. Implemented the `removeItem` method to remove items from the inventory This demonstrate your understanding of key memory management concepts in C++. Next up, you'll implement some more advanced features.
- Delete each
-
Challenge
Implementing Advanced Features
In this step, you'll implement more advanced features for the
InventoryManager
class, focusing on complex pointer operations and memory management. ### Adding Search-by-name CapabilitiesYour next task is to implement the
searchByName
function, which will enable searching for items by name instead of just by ID. ### Batch-updating an ItemThe
updateItem
function allows you to update multiple properties of an item at once. However, you'll need to provide the missing implementation first. ### Implement theremoveItem
FunctionRemoving items from a dynamically allocated array requires careful pointer manipulation. Your next task is to implement the
removeItem
function that deletes an item and reorganizes the remaining pointers.Start by locating the
removeItem
function inInventoryManager.cpp
. To see how your changes affect the system's behavior, click Run in the lower-right corner of the Terminal. Alternatively, execute the following commands:make -C src src/build/inventory_system ``` ### Summary In this step, you've implemented three advanced features for the inventory management system: 1. The`1searchByName` method, which allows searching for items by name 2. The `updateItem` method, which enables updating multiple properties of an item at once 3. The `removeItem` method, which deletes an item and reorganizes the remaining pointers These features build on the basic functionality you implemented in Step 2 and demonstrate more complex pointer operations and memory management techniques. The ability to properly manipulate pointers, reorganize arrays, and handle memory allocation and deallocation is essential for C++ programming. These skills will serve you well in more advanced C++ projects.
-
Challenge
Where to Go From Here
Congratulations!
Well done on completing the Inventory Management System! You've successfully:
- Implemented a class for managing inventory items
- Created a system that dynamically allocates and manages memory using pointers
- Built features for adding, searching, displaying, updating, and removing items
- Gained practical experience with C++ memory management
These skills form the foundation of more advanced C++ programming and will serve you well as you continue your journey.
Next Steps in Your C++ Journey
The manual memory management you've practiced in this lab is important for understanding how C++ works "under the hood".
As you grow as a developer, you'll likely use the modern C++ features that make memory management easier and safer:
- Smart Pointers automatically handle memory cleanup when objects are no longer needed.
- Container Classes, such as
std::vector
, manage their own memory. - Modern C++ Techniques lead to safer code.
Learning Resources
To continue building your C++ skills, check out the courses and paths available in Pluralsight's library.
Wishing you luck with your C++ projects!
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.