- Lab
- Core Tech
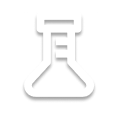
Guided: Exploring Branching with JavaScript
In this lab, you’ll develop a dynamic web application designed to handle restaurant orders and calculate total costs, incorporating discounts under certain conditions. This Code Lab offers a practical scenario that leverages branching, conditional logic, and DOM manipulation in JavaScript, providing hands-on experience with real-world applications of programming concepts.
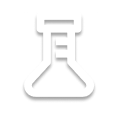
Path Info
Table of Contents
-
Challenge
Introduction
In this Code Lab, you are provided with a pre-built Restaurant Order System. This interactive web application is designed to allow users to enter their order details and dynamically calculate the total cost with applicable discounts based on the order type and amount. Your goal will be to implement the core functionalities using JavaScript to handle user inputs and conditional logic for discount application.
How Discounts Are Calculated:
- Dine-In Orders: A 10% discount is applied for dine-in orders over $50.
- Takeaway Orders: A 5% discount is applied for takeaway orders exceeding $100.
- Default: No discounts are applied if the conditions above are not met.
You Will Work Within the Following Files:
Within the
src
directory, you will find:script.js
: Contains skeleton functions that you will complete to process ordersstyle.css
: Provides initial styling for the application
Within the
public
directory, you will find:index.html
: Features the form elements for user input and placeholders for displaying results
Excited? Time to dive in!
-
Challenge
Implement Order Submission
First, you need to declare constants
orderAmount
andorderType
to store the order amount and order type from the form inputs within thesubmitOrder
function. These two are essential for determining the final price of an order.orderAmount
helps in applying quantity-based discounts, whileorderType
allows for different pricing strategies depending on whether the order is for dine-in or takeaway. Once you haveorderAmount
, you should validate it to make sure the data used in computations is correct and sensible. This is important since it is the value you will use for discount calculations and to get the final price. After validating theorderAmount
, the next step is to get the final price based on the order type and amount. For this, you will be invoking thecalculateFinalPrice
function using the values retrieved fromorderAmount
andorderType
. -
Challenge
Apply Conditional Discounts
What is Branching in Programming?
Branching allows your code to make decisions, similar to how you decide what to wear based on the weather. It equips your program with the ability to choose between actions based on conditions.
In JavaScript, these decisions are guided by conditional statements.
Basic Structure of
if
StatementsThe simplest form of branching is the
if
statement. Here’s how it looks:if (condition) { // Code to execute if the condition is true }
- Condition: This test evaluates to either true or false. The expression used here should result in a boolean value, either true or false.
- Statements between curly braces: This code executes if the condition is true.
For example:
if (temperature > 75) { console.log("It's warm outside!"); }
In this example, if the temperature exceeds 75 degrees, JavaScript will print "It's warm outside!" to the console.
Extending with
else if
To handle scenarios where the if condition does not hold true, you can use
else if
.else if
provides a way to check another condition if the first condition is false.if (temperature > 75) { console.log("It's warm outside!"); } else if (temperature < 60) { console.log("It's cold outside!"); }
Making Use of Logical Operators
In JavaScript, conditions within an
if
statement return a boolean value — true or false. To manage complex logic efficiently without numerouselse if
clauses, you can use logical operators like&&
(logical AND),||
(logical OR), and!
(logical NOT). These operators allow you to combine multiple conditions into a single expression, simplifying and enhancing the decision-making process in your code. Once you are done with the order submission, you need to implement logic to determine the discount eligibility in thecalculateFinalPrice
function in thesrc/script.js
file. Eligibility needs to be based on the order type and the order amount. This function will check if the order qualifies for discounts according to predefined criteria for dine-in and takeaway options. This value will be used in yoursubmitOrder
function that you worked on. Now its time that you get the logic for discounting set. Remember, you need to apply a 10% discount for dine-in orders that exceed $50 and a 5% discount for takeaway orders that are more than $100. After determining and setting the discount rate based on the order type and amount, your next task is to calculate the final price considering any discounts applied and return this value. This is the final step in the function and ensures that the computed value is passed back correctly for further use or display in the application. -
Challenge
Display the Final Order Total
Once you have the final price with the right discount applied, it's important that you dynamically display the calculated order total immediately after computations. This will involve retrieving the computed value from the
calculateFinalPrice
function and updating the relevant HTML element to show this total. You already havefinalPrice
holding the final order total after callingcalculateFinalPrice
. You need to use this variable to update the content of an HTML element that is intended to display the order total. Wow! You are almost done. Now you need to make your application interactive. You should not only display the final total, but also format it properly as currency with a nice message. This will involve enhancing the text content to include descriptive text and ensuring the price is prefixed with a dollar sign ($). Well, you are done! Head over to the Web Browser tab and use the Open in new browser tab icon to try out the application! Enter in different order amounts in the input and selecting different values for order type to see different discounts being applied based on your selection.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.