- Lab
- Core Tech
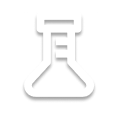
Guided: Exploring API Versioning Strategies
Discover the essentials of effective API versioning in this Guided Code lab. You'll explore several strategies, including versioning via URI, query parameters, headers, and content negotiation. Through practical tasks, you'll gain insights into the advantages and disadvantages of each method, enabling you to choose the optimal versioning strategy for your APIs.
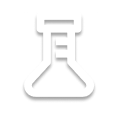
Path Info
Table of Contents
-
Challenge
Introduction
In this Guided Code Lab, you will explore various API versioning strategies. Starting with an introduction to the need for versioning and its importance in API development, you'll progressively dive into different strategies for implementing versioning. Through hands-on tasks, you will learn how to apply each strategy, evaluate their benefits and drawbacks, and understand best practices for maintaining backward compatibility, while iterating on your API design.
You will go through the following in this lab:
- Understand API Versioning
- Learn why versioning is essential for API evolution and stability.
- Versioning via URI
- Explore the most common versioning strategy where the version number is included in the URI path.
- Versioning Using Query Parameters
- Learn how to implement versioning by adding version information in query parameters.
- Versioning with Headers
- Explore a more flexible versioning strategy using custom headers.
- Content Negotiation Versioning
- Discover how to use content negotiation to manage API versions by specifying the version in the
Accept
header.
- Discover how to use content negotiation to manage API versions by specifying the version in the
- Evaluating Strategies
- Understand the pros and cons of each versioning method. Learn best practices for selecting and implementing a versioning strategy that suits your API's needs.
By the end of this lab, you'll have a thorough understanding of different API versioning strategies, empowering you to design APIs that can evolve gracefully without breaking existing clients.
Before you begin, here are some key points:
- Your task involves implementing code in the Java files located in the
/src/main/java/com/pluralsight/controller/
directory. - If you encounter any challenges along the way, feel free to consult the
solution
directory. - To simplify locating changes, comments are added to indicate the required modifications for each task.
- Understand API Versioning
-
Challenge
Importance and Need for Versioning
Application Programming Interfaces (APIs) are crucial in modern software development, enabling different systems to communicate. As APIs evolve, changes can break existing clients. Versioning addresses this by allowing APIs to change without disrupting clients.
The Need for Versioning
-
Maintaining Backward Compatibility:
- Client Dependencies: Ensures existing clients continue to function
- Incremental Improvements: Introduces changes gradually to ensure stability
-
Handling Deprecated Features:
- Graceful Decommissioning: Phases out old features without immediate disruption
- Clear Communication: Informs users about feature lifecycles
-
Facilitating Continuous Development:
- Parallel Development: Allows teams to work on different API versions simultaneously
- A/B Testing and Experimentation: Tests new features on a subset of users
Importance of Versioning in API Development
-
Ensuring Stability and Reliability:
- Minimizing Downtime: Maintains service reliability during updates
- Risk Mitigation: Isolates changes to minimize widespread failures
-
Enhancing Flexibility and Scalability:
- Customizable Client Experience: Caters to diverse client needs with different API versions
- Supporting Multiple Platforms: Efficiently supports various platform requirements
-
Improving Developer Productivity:
- Streamlined Development Process: Manages changes effectively, reducing complexity
- Enhanced Collaboration: Allows multiple teams to work concurrently without interference
-
Building Trust with Clients:
- Transparent Evolution: Clearly communicates changes to build trust
- Customer Satisfaction: Provides a stable API experience, enhancing client satisfaction
Versioning is essential in API development to maintain compatibility, manage deprecated features, and support continuous improvement. It ensures stability, enhances flexibility, improves productivity, and builds client trust, enabling a robust and seamless API experience. Now that you understand the importance of API versioning, you'll explore each versioning strategy in detail through a real-life scenario in the following steps.
-
-
Challenge
Scenario
Consider a scenario where you are developing an online store application, and you have APIs that currently support a single version of the API that returns information in a simple format.
UserController
GET /api/users/123
Examine the
UserController.java
file. It retrieves user data based on the providedid
in the request, returning the user's full name by concatenating thefirstName
andlastName
.However, you have received requests from clients to provide more structured data, separating
firstName
andlastName
in the next version of your API.To ensure backward compatibility with multiple clients as you keep on modifying and introducing the new format, you need to implement API versioning. This will allow clients to choose which version of the API they want to use and provide them with the data in the required format.
Current API Response For the current version (v1), the API response for a user is:
// v1 - Firstname Lastname together { "name": "John Doe" }
New API Requirement For the new version (v2), the API response should be:
// v2 -Firstname and Lastname separate { "firstName": "John", "lastName": "Doe" }
To achieve this goal, you'll implement API versioning with separate endpoints for each strategy:
/api1
: URI versioning/api2
: Query parameter versioning/api3
: Header versioning/api4
: Content negotiation versioning
This approach enables a thorough exploration and assessment of each strategy's strengths and weaknesses, providing insights into their effectiveness.
-
Challenge
URI Versioning
Uniform Resource Identifier (URI) versioning entails embedding the version number directly in the URL path, making it a straightforward and widely adopted versioning strategy. This technique helps manage and evolve APIs over time by including the version number in the URI of the API endpoint. It maintains backward compatibility and allows clients to specify which version of the API they want to interact with.
Key aspects of URI versioning include:
1. Version Identifier in URI:
- The version number is included directly in the URI path, such as
v1
in the example below:
GET /api1/v1/users/123 GET /api1/v2/users/123
2. Backward Compatibility:
- Older API versions continue to function even as new versions are developed.
- Clients can use an older version without being affected by changes in newer versions. For instance,
/v1
will still work while/v2
is being released.
3. Client Flexibility:
- Clients can specify which version of the API they are using.
- This allows for gradual migration to newer versions.
Advantages:
1. Clear Versioning:
- It's easy to see which version of the API is being used just by looking at the URI (e.g.,
/v1/
,/v2/
).
2. Isolation of Changes:
- Changes to the API are isolated to specific versions, reducing the risk of breaking existing clients when updating the API.
3. Simple to Implement:
- Implementing versioning in the URI is straightforward and doesn’t require complex header configurations or query parameters.
Disadvantages:
1. URI Pollution:
- Multiple versions can lead to a cluttered and less readable URI structure.
- Maintaining several versions can be cumbersome.
2. Resource Duplication:
- There may be duplication of resources across different versions, leading to increased maintenance overhead.
3. Client Update Required:
- Clients need to update their code to point to the new version URI, which might be inconvenient if frequent updates are required.
Great! Next, you'll look into query parameter versioning.
- The version number is included directly in the URI path, such as
-
Challenge
Query Parameter Versioning
Query parameter versioning involves embedding the version number in the query string of the URL. This is another common strategy for managing and evolving APIs over time. This method allows for flexibility and backward compatibility, enabling clients to specify which version of the API they want to interact with.
Key aspects of query parameter versioning include:
1. Version Identifier in Query Parameter:
- The version number is included in the query string of the URL, such as
?version=1
in the example below:
GET /api/users?version=1 GET /api/users?version=2
2. Backward Compatibility:
- Older API versions continue to function even as new versions are developed.
- Clients can use an older version without being affected by changes in newer versions. For instance,
?version=1
will still work while?version=2
is being released.
3. Client Flexibility:
- Clients can specify which version of the API they are using.
- This allows for gradual migration to newer versions.
Advantages:
1. Clear Versioning:
- It's easy to specify the API version using query parameters (e.g.,
?version=1
,?version=2
, etc.).
2. Isolation of Changes:
- Changes to the API are isolated to specific versions, reducing the risk of breaking existing clients when updating the API.
3. Minimal Impact on URI Structure:
- Query parameter versioning does not clutter the URI path, keeping the base path clean.
4. Simple to Implement:
- Implementing versioning with query parameters is straightforward and doesn’t require complex configurations.
Disadvantages:
1. Less Discoverable:
- It may be less obvious which version of the API is being used compared to URI versioning.
2. Potential for Confusion:
- Clients might overlook specifying the version parameter, leading to potential inconsistencies.
3. Client Update Required:
- Clients need to update their code to include the new version parameter, which might be inconvenient if frequent updates are required.
4. Possible Query String Pollution:
- Multiple query parameters can lead to a cluttered and less readable URL structure.
Now that you understand the query parameter versioning, which uses request param, the next two strategies will focus on using request headers for versioning.
- The version number is included in the query string of the URL, such as
-
Challenge
HTTP Header Versioning
HTTP header versioning involves specifying the API version using custom HTTP headers in the request. This approach offers a structured and standardized way to manage and evolve APIs over time. By embedding the version number in HTTP headers, backward compatibility is preserved, and clients can explicitly indicate which version of the API they intend to use.
Key aspects of HTTP header versioning include:
1. Version Identifier in HTTP Headers:
- The version number is included as a custom HTTP header, such as
X-API-Version: 1
in the example below:
GET /users/123 HTTP/1.1 Host: api.pluralsight.com X-API-Version: 1
2. Backward Compatibility:
- Older API versions continue to function as new versions are introduced.
- Clients can specify an older version in the header (
X-API-Version: 1
), while newer versions (X-API-Version: 2
) are developed.
3. Client Flexibility:
- Clients have the flexibility to specify which version of the API they want to interact with.
- This allows for gradual migration to newer versions at their own pace.
Advantages:
1. Clean URI Structure:
- The URI remains clean and version-free, improving readability and aesthetics.
2. Isolation of Changes:
- Changes to the API are isolated to specific versions defined in the headers, reducing the risk of breaking existing clients.
3. Standardized Approach:
- Using HTTP headers for versioning adheres to HTTP protocol standards, ensuring compatibility and interoperability.
4. Extensibility:
- Additional metadata or versioning strategies can be included in headers without impacting the URI or query parameters.
Disadvantages:
1. Less Discoverable:
- Identifying the API version requires inspecting the headers, which may be less intuitive compared to URI-based versioning.
2. Header Dependency:
- Clients must correctly set and interpret headers, adding complexity compared to URL-based versioning.
3. Potential Overhead:
- Managing and processing headers can introduce additional overhead in both client and server implementations.
4. Client Update Required:
- Clients need to update their applications to include or modify headers when switching to a new API version, which can be cumbersome if updates are frequent.
The next content negotiation strategy is similar to the header versioning strategy, but instead of using a custom header, you will utilize the
Accept
header for versioning.
- The version number is included as a custom HTTP header, such as
-
Challenge
Content Negotiation Versioning
Content negotiation versioning involves specifying the API version through the
Accept
header in the HTTP request. This method allows clients to request a specific version of the API response format, facilitating flexible and version-aware interactions.Key aspects of content negotiation versioning include:
1. Version Specification in Accept Header:
- The API version is indicated in the
Accept
header using a media type parameter, such asapplication/vnd.company.api.v1+json
in the example below:
GET /users/123 HTTP/1.1 Host: api.pluralsight.com Accept: application/vnd.company.api.v1+json
2. Backward Compatibility:
- Older API versions continue to be supported alongside newer versions.
- Clients can request a specific version format (
v1
,v2
, etc.) depending on their needs and compatibility requirements.
3. Client Flexibility:
- Clients have granular control over which API version and response format they wish to interact with.
- This approach supports gradual migration to newer API versions based on client capabilities and preferences.
Advantages:
1. Granular Version Control:
- Clients can specify precise API versions and response formats through structured media type parameters.
2. Enhanced Compatibility Management:
- Content negotiation helps manage compatibility challenges between different API versions more effectively.
3. Standardized Approach:
- Using media type parameters in the
Accept
header aligns with HTTP protocol standards and RESTful principles.
4. Flexible Response Formats:
- Clients can negotiate not only API versions, but also preferred content types (JSON, XML, etc.) in a single request.
Disadvantages:
1. Complexity in Header Management:
- Managing and interpreting complex
Accept
headers requires careful attention to ensure correct versioning and content type negotiation.
2. Potential Overhead:
- Content negotiation may introduce additional overhead in processing requests and responses compared to simpler versioning methods.
3. Learning Curve:
- Understanding and implementing content negotiation effectively may require additional learning for developers and API consumers.
4. Client Update Required:
- Clients need to update their applications to specify the appropriate
Accept
header format when switching to a new API version, which may require significant changes in some cases.
- The API version is indicated in the
-
Challenge
Pros and Cons of each versioning strategy
Evaluation of Versioning Strategies
| Strategy | Pros | Cons | | -------- | -------- | -------- | | URI Versioning | Simple and clear, easy to understand and document | URL structure changes with versions, could lead to cluttered API paths | | Query Parameter Versioning | Keeps base URL clean, versions can be managed more flexibly | Not as immediately visible as URI versioning, can complicate caching mechanisms | | Header Versioning | Clean URL, allows for sophisticated versioning strategies | Hidden from URL, requires clients to manage headers correctly, harder to test manually | | Content Negotiation Versioning | Granular control over API versions and response formats, aligns with HTTP standards, supports flexible response formats | Complexity in managing
Accept
headers, potential overhead, steeper learning curve for implementation, requires clients to update headers correctly |
Guidelines for Choosing a Strategy:
-
URI Versioning:
- Simple and clear, suitable for public APIs where readability and straightforwardness are essential
-
Query Parameter Versioning:
- Keeps the base URL clean and allows flexible version management, useful for APIs with multiple versions
-
Header Versioning:
- Offers a clean URL and sophisticated versioning strategies, ideal for complex scenarios where decoupling versioning from the URL structure is beneficial
-
Content Negotiation Versioning:
- Provides granular control over API versions and response formats, aligning with HTTP standards, suitable for APIs needing flexible response formats
-
-
Challenge
Conclusion & Next Steps
By completing this lab, you have learned how to implement various API versioning strategies and understand the benefits and trade-offs of URI versioning, query parameter versioning, header versioning, and content negotaion versioning. Effective API versioning is essential for allowing APIs to evolve without disrupting existing clients. Each strategy has its own strengths and weaknesses, and the best choice depends on the specific needs and constraints of your API.
Next Steps:
- Evaluate Your Requirements: Analyze the specific needs of your API, including the target audience, complexity, and the expected lifecycle of the API versions.
- Choose a Versioning Strategy: Select the versioning strategy that best aligns with your requirements. Consider factors such as ease of implementation, client impact, and long-term maintenance.
- Implement the Chosen Strategy: Develop your API using the chosen versioning method. Ensure that your documentation clearly explains how clients can specify and use different versions.
- Test Thoroughly: Validate that the versioning works as expected. Test with various clients to ensure backward compatibility and smooth transitions between versions.
- Monitor and Iterate: Continuously monitor the usage of different API versions and gather feedback from clients. Be prepared to iterate on your versioning strategy as your API evolves and new requirements emerge.
By carefully selecting and implementing an appropriate versioning strategy, you can ensure that your API remains robust, flexible, and user-friendly as it grows and adapts to new challenges.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.