- Lab
- Core Tech
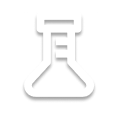
Guided: DOM Manipulation with Refs in React
Unlock the full potential of React by mastering DOM manipulation with refs in this hands-on Code Lab. Build an interactive and dynamic To-Do List Application from scratch. Designed for React developers, this Guided Lab will take you through the essentials of using refs to manage DOM elements directly, enhancing user interactions and improving your application's responsiveness.
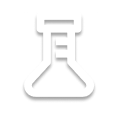
Path Info
Table of Contents
-
Challenge
Introduction
Introduction to the To-Do List Application
In this Code Lab, you are provided with a pre-built To-Do List Application. This interactive web application is designed to help you manage your tasks by allowing you to add, edit, and remove items from a task list. Your goal will be to implement the core functionalities using React to handle user interactions and manage state effectively.
Application Features:
- Add New Tasks: You can add new tasks to the list by typing into an input field and clicking the Add Task button.
- Edit Existing Tasks: You can edit tasks by clicking the Edit button next to a task, making changes, and then saving the changes.
- Remove Tasks: You can remove tasks from the list by clicking the Remove button next to a task.
You Will Work within the following Files:
Within the
src
directory, you will find:App.js
: Contains the main React component with skeleton functions for adding, editing, and removing tasksApp.css
: Provides initial styling for the application, ensuring a user-friendly interface
Provided React Components:
- App Component: The main component that manages the task list and renders the user interface
- State Management: Uses React hooks (
useState
,useRef
, anduseEffect
) to manage the state and handle user interactions - Input Fields: Contains input fields for adding new tasks and editing existing tasks
- Task List: Renders a list of tasks with options to edit and remove each task
- State Management: Uses React hooks (
CSS Styling:
App.css
: Defines styles for the task list, buttons, and input fields to enhance user interaction and provide a clean layout
JavaScript Functions to Implement:
handleAddTask
: Function to add a new task to the task listhandleRemoveTask
: Function to remove a task from the task listhandleEditTask
: Function to initiate the editing of a taskhandleSaveTask
: Function to save the edited taskuseEffect
: Hook to handle side effects, such as focusing the input field when editing a task
How Refs Are Used in Starter Code
-
Creation of Refs:
const newTaskRef = useRef(null); const editTaskRef = useRef(null);
useRef(null)
is called to create two refs,newTaskRef
andeditTaskRef
. These refs are initialized tonull
and will later be used to reference specific input fields.
-
Attaching Refs to Input Fields:
<input ref={newTaskRef} type="text" placeholder="Add new task" /> <input ref={editTaskRef} type="text" defaultValue={task} />
- The
ref
attribute is used to attachnewTaskRef
to the Add new task input field andeditTaskRef
to the input field used for editing tasks. This allows you to directly interact with these DOM elements.
- The
Starting the Server
You need to start the server by running the command below in the Terminal:
npm start
This will start the server on port 3000.
Head over to the Web Browser tab and use the Open in new browser tab icon to try out the application!
Excited? Time to dive in!
-
Challenge
Implementing `handleAddTask` Function
In this step, you will implement the
handleAddTask
function to allow users to add new tasks to the list. This will involve retrieving the value from the input field, validating the task, updating the state, clearing the input field, and refocusing the input field.First, you need to retrieve the value entered in the input field using the
ref
. This value represents the new task to be added to the to-do list. Next, you need to ensure that the task has a valid value before adding it to the list. Validation is important to ensure that users cannot add empty tasks to the list. By using anif
statement, you can control the flow of the function to only proceed when a valid task is entered. React state management allows you to update the component's state and trigger a re-render. By using thesetTasks
function, you can update thetasks
array with the new task. Clearing the input field provides a better user experience, making it ready for the next entry. Finally, you will refocus the input field after adding a task. This will improve the user experience by allowing the user to quickly enter another task without needing to manually click on the input field.Refocusing the input field using
ref
improves the usability of the application. The user can continuously add tasks without needing to manually focus the input field each time. -
Challenge
Implementing `handleEditTask` and `handleSaveTask` Functions
Refs provide a way to access DOM nodes or React elements directly, which allows for direct manipulation. Using refs, you can access input fields, focus them, and retrieve or set their values. This is particularly useful in scenarios where you need to interact with the DOM directly, such as form handling and dynamic updates.
In this step, you will implement the
handleEditTask
andhandleSaveTask
functions to allow users to edit tasks in the to-do list. This will involve setting the edit mode, retrieving the edited value, updating the state, and exiting the edit mode.You will begin by working on the
handleEditTask
function first, where you will set the edit mode for the selected task. This will allow you to edit the task. Now, you need to work on thehandleSaveTask
function. This is responsible for saving the edited task back into thetasks
state array and updating the UI accordingly. Now, you need to update thetasks
state array with the new task value. React state management allows you to update the component's state and trigger a re-render. After saving the edited task, you now need to exit the edit mode by resetting theeditIndex
state tonull
. Resetting theeditIndex
state ensures that the task list returns to normal mode, and the input field is reset for the next task. This improves the user experience by clearly indicating that the task has been saved. -
Challenge
Implementing `handleRemoveTask` Function and Using `useEffect` to Manage Focus
In this step, you will enhance the functionality of the to-do list application by allowing users to remove tasks and managing the focus of the input field when a task is being edited. This involves two main parts:
- Implementing the
handleRemoveTask
function to remove tasks from the state - Using the
useEffect
hook to automatically focus the input field when a task is selected for editing
First, you will start with the
handleRemoveTask
function, where you need to remove the selected task from thetasks
state array.When working with arrays, you use of array filtering with the filter method. This is a built-in JavaScript array method that creates a new array with all elements that pass the test implemented by the provided function. After removing the selected task from the state array, you need to update the state immediately to reflect the removal in the UI. Now, you will implement the
useEffect
hook to automatically focus the input field when a task is selected for editing. Now, you need to handle focus management correctly (i.e. only when a task is being edited). You can achieve this usingeditIndex
and checking if it is notnull
. Now, you need to focus on the input field, as this improves user experience by allowing the user to start typing immediately without having to click on it. Well, you are done! Head over to the Web Browser tab and use the Open in new browser tab icon to try out the application! Enter in different tasks in the input and even try performing operations like editing and deleting tasks to see how your application behaves. - Implementing the
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.