- Lab
- Core Tech
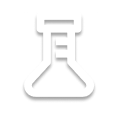
Guided: DOM Manipulation with JavaScript
In this Guided Code Lab, you’ll dive into creating a vibrant and interactive web application that allows users to generate and interact with color palettes. This lab covers essential web development techniques like DOM manipulation, JavaScript event handling, and dynamic CSS adjustments. By participating, you'll gain practical experience in crafting dynamic interfaces and providing real-time user feedback, skills crucial for any budding web developer.
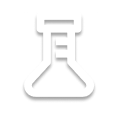
Path Info
Table of Contents
-
Challenge
Introduction
Welcome to ColorScape
In this Code Lab, you are provided with a pre-built web application that allows users to generate and interact with unique color palettes. The app provides an intuitive user interface, making it easy to discover new color combinations and copy them for use in other projects. Your goal is to implement the functionality to generate a color palette and enable user interaction with the colors.
You Will Work Within the Following Files:
Within the
src
Directory:script.js
: Contains skeleton functions that you will complete to generate vibrant colors and display them to your users.style.css
: Provides initial styling for the application.
Within the
public
Directory:index.html
: Features the main HTML structure, including the "Generate Palette" button and the color palette display.
Excited? Time to dive in!
-
Challenge
Beginning to Generate Color Palette
First, you need to retrieve the element where the generated palette will be displayed. This will be done inside the event listener function in the
src/script.js
file. This element will hold all the color blocks that make up the palette. Before generating a new palette, ensure that any previously generated colors are cleared. This prevents old colors from mixing with the newly generated ones and provides a fresh palette each time. You need to generate five colors in the palette, so you will need to set up a loop to iterate over the palette generation logic. -
Challenge
Implement Color Palette Generation
Now it's time that you build the functionality to generate and display a palette of random colors. Once you have the
palette
andfor
loop set up, you need to provide each color block with a unique color using a random hexadecimal color code. The hexadecimal format starts with#
and is a common representation for colors in web design, enabling the user to see each color in a familiar and universally accepted format.Hexadecimal Color Codes
Hexadecimal color codes consist of six characters, representing combinations of red, green, and blue values. Since each color component can have values ranging from 00 to FF (equivalent to decimal 0 to 255), the highest possible hexadecimal value for each component is FF. Therefore, the maximum hexadecimal color code, representing pure white (
#FFFFFF
), is equivalent to decimal 16777215. Since you have the color generator, now you need to create a<div>
element that would provide a visual representation of the generated color. Setting thebackgroundColor
property to the generated hexadecimal color code turns each<div>
into a color block, enabling users to interact visually. This is how colors are generated and represented in the form of a color palette. You now have<div>
representing the color generated. Next, you need a<span>
element that will hold the hexadecimal color code as text, providing additional information for each color block. By showing the hexadecimal code, users can easily identify and copy the color values for use in other projects. Awesome, right? You currently have a<div>
representing the generated color and a<span>
element containing the hexadecimal color code. To present them together as a unified visual element on the interface, combine them into what can be termed a Color Block. By doing so, each color block will include both the color and its corresponding code, enhancing the user experience through comprehensive information within a single element Next, you need to add each generated color block to the mainpalette
on the webpage by appending each<div>
element to thepalette
element. This enables users to view all generated colors together, creating a cohesive palette that they can interact with. -
Challenge
Implement Custom Message to Display the Copied Color
Now, you need to work on the
showNotification
function to create apopup
notification that appears and fades out after copying a color. You now need to ensure that the notification displays the message passed to theshowNotification
function. This is a message that you will be adding in subsequent tasks. You have a<div>
created and it has the message you need to display. It is time to add this to the document body to ensure that it appears on the webpage. Now that you have a notification that is visible, you need to ensure that it hides after two seconds. To do this, you will by using a timer that will ensure that the notification is visible for only a brief period before being hidden. You now need to hide the notification and remove it from the document body ensuring that the page remains uncluttered and only active notifications are visible. This logic needs to be handled insidesetTimeout
. -
Challenge
Enhance Interactivity by Displaying Notifications
Now its time for you to enable users to interact with the color segments, copy the color code, and provide visual feedback when a color code is copied by showing a notification. You will do this by adding an event listener that allows you to capture user interactions with each color segment. This way, you can execute the required code to copy the color code when a color segment is clicked. Well, you are done! Head over to the Web Browser tab and use the Open in new browser tab icon to try out the application! Click the Generate Palette button to see a new set of colors. Interact with the color blocks by clicking on them to copy the color code and see the notification that appears. Enjoy experimenting with different palettes and see the notifications in action!
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.