- Lab
- Core Tech
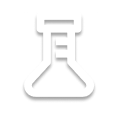
Guided: Debugging with Git
It's inevitable. Bad commits will enter your codebase! It's best to be prepared to find and fix bugs introduced by these bad commits. In this Guided Lab, you will learn the ins and outs of how to navigate commit history, use the bisect command to find bugs fast, and remediate bad commits once they are found.
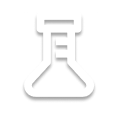
Path Info
Table of Contents
-
Challenge
### Introduction
It’s inevitable that bugs will be introduced into production. This is especially true in larger codebases that have many moving parts and contributors. One of the first questions asked when a bug gets into production is inevitably, “When did this bug get introduced into the codebase?”. Sometimes it is easy to answer this question especially when you know that the bug is contained within a new feature. But what about more subtle bugs? With these, it can be hard to figure out when exactly the bug was introduced. To make matters worse, when it comes to finding and fixing bugs, most people don’t know how to take advantage of Git’s debugging capabilities. In this Guided Lab, you’ll learn how to use Git to identify buggy commits and remediate them – a crucial portion of every Git workflow.
First up in this lab, you’ll learn the naive approach to debugging in Git. Namely, you’ll learn the slow way to go about debugging. Along the way, you’ll learn crucial aspects related to navigating Git history and inspecting commits.
From there, you’ll learn how to drastically speed up your buggy commit search by way of the lesser-known
git bisect
command. You’ll learn howgit bisect
takes advantage of the binary search algorithm to help you quickly find what commit a bug is originating from.Lastly, you’ll learn how to revert commits, amend commit messages and even use Git to fix a bug while retaining a clean (and linear) Git commit history. There are many approaches to fixing buggy commits in Git so in this step you’ll learn the approach that has the most utility.
Should you get stuck while following along, you will find a
solution.txt
file within themaster
branch. This file details the commands to run in order to successfully complete each task. To locate it simply rungit checkout master
and view it in the file explorer. Thesolution.txt
file in thebugfix
branch will be out of date (this is done intentionally to help with therebase
command used later in this lab).info> Note: Changes made to this file system using Git may not automatically be conveyed in the filetree at times. If this happens, you can visualize changes to files by exiting out of the file and reopening it.
-
Challenge
### Basic Git Debugging
Trying to find which commit introduced a bug into your codebase can be very, very time consuming. To fully appreciate the power of debugging with Git using
git bisect
, it’s important to understand alternative bug-hunting methods. It’s also important to understand how to search through Git history. There are four main Git commands that help with searching through your commit history. These commands include:-
git log
-
git show
-
git diff
-
git blame
Throughout this step, you’ll learn about and use each one of these commands as you attempt to find a buggy commit! When it comes to debugging with Git, it’s very important to understand how to navigate your Git history and inspect commits. The first critical command to understand is the
git log
command. The purpose ofgit log
is to display the public commit history of a given branch. Usinggit log
without any arguments or flags will display the commit history for your current branch. Displaying the history of a specific branch is as easy as specifying the branch name i.e.git log my-branch
. You can view this link to check out the fullgit log
documentation along with all possible arguments and flags. info> Note: The concept ofHEAD
in Git is an important one. To put it simply,HEAD
points to the tip (the latest commit) of the current branch. You can also be in what is known as a “detachedHEAD
state”. This basically means that you are no longer situated on a specific branch - instead you are currently pointing to a commit directly. This is typically not a place you want to stay as you can’t commit code in a detachedHEAD
state. Thegit log
command is great for getting a full picture of recent commit history. But what if you want to look a little deeper into a specific commit. This is wheregit show
comes in to play. Thegit show
command will display the message for a commit and the actual changes introduced by the commit compared to the commit prior (if its not a “merge” commit). This can come in handy when you want a quick look into the specifics of a given commit. Thegit show
command is great but what if you want to compare two commits specifically? Thegit diff
command will compare commits that you specify, giving you a text representation of the differences between those two places in history. Thegit diff
command is very useful when it comes to debugging in Git. Another quite useful command for debugging in Git is thegit blame
command. Thegit blame
command works by annotating a given file with information related to its latest changes line by line. Annotated information includes the date of the change, the commit SHA, and the name of the author of the change. It’s time for you to see if you can use it to try and identify the bug and maybe ask the person who brought the bug into the baseline for more specifics on how to fix it or for the reasons for the code change in the first place. In this step you learned several different commands:-
git log
-
git show
-
git diff
-
git blame
These are very general tools and can be very helpful for finding and fixing bugs in Git... but what if your commit history is extremely large? What if you have many development teams making lots and lots of commits every day? In the next step you’ll uncover some more advanced debugging techniques that can save you a lot of time!
-
-
Challenge
### Efficient Debugging Using Bisect
In the last step you gained a foundational understanding of debugging in Git. You know how to inspect and traverse Git commit history but you’ve also found that this manual approach is quite cumbersome and not very efficient. There is a better way! Enter
git bisect
. Thegit bisect
command takes advantage of a computer science searching algorithm – binary search. Binary search works by breaking the search surface area down into halves. By first sorting and then recursively making the searchable area smaller and smaller, what you are searching for can be found very fast. Thegit bisect
command is simply binary search for git commits! In this step, you are going to learn how to usegit bisect
to find buggy commits fast! Thegit bisect
command is an interactive one. To kick off a new debugging session you must run the commandgit bisect start
. After beginning a new debugging session withgit bisect
, the next step is to tell this command where a known “bad” state is. This step is mostly easy as usually the first commit you want to feedgit bisect
isHEAD
. After tellinggit bisect
of a known, “bad” commit, the command needs to know the first known “good” commit. That way,git bisect
can start to work its magic via binary search. Once you’ve identified the bug viagit bisect
you’ll want to ensure you copy the commit SHA of the bad commit and then you can use thegit bisect reset
command to exit. You’re now adept at finding bugs and finding them fast! But what should you do once you’ve identified a buggy commit? In the next step, you’ll learn a tried-and-true and also efficient method for fixing these bad commits. -
Challenge
### Fixing Bad Commits
When it comes to fixing buggy commits, the possibilities are almost endless. There are a lot of different ways to address a bad commit. In this step, you’ll learn how to:
-
Revert bad commits
-
Amend commit messages
-
Ensure your branch history is clean after fixing a bad commit with
git rebase
This portion of the lab is nicely paired with another lab:
Guided: Git Reflog and Recovery
. That lab coversgit revert
andgit reset
in depth. If you haven’t already, I highly recommend you check that lab out. When it comes to fixing bugs that are contained within a single commit, the quickest and easiest way to fix the bug is to revert the change. This is done with thegit revert
command. Thegit revert
command, given a commit hash, will create a new, inverse commit. For example, if you have a commit that adds the word “dog” to a file, reverting that commit will take away the word “dog” from the same file while leaving your commit history intact. Sometimes while debugging in Git you will accidentally create a commit message that isn't as descriptive as it could be. This can be a problem in large codebases as the descriptive commit messages really help when navigating commit history. Luckily, it’s easy to update the message of the most recent commit withgit commit --amend
. You’ve fixed the bug but where do you go from here? Well from here you would submit a merge or pull request to the remote repository so that the bugfix can eventually enter into production after a code review. But while you’ve been fixing this bug, themaster
branch has received some updates. You’ll need to update your current branch with the latest frommaster
branch. The best way to accomplish this while retaining a very clear Git history is to use thegit rebase
command. Thegit rebase
command will replay your bug fix on top of the latest changes frommaster
within yourbugfix
branch. This ensures that you cut down on ugly “merge” commits and makes your Git history easy to navigate and reason about. -
-
Challenge
### Conclusion
Nicely done! You’ve made it to the end of this Guided Lab on debugging with Git. In this lab, you first laid a foundation of simple debugging with Git where you learned how to traverse commit history and inspect commits.
From there, you learned how to improve the process of finding buggy commits by way of
git bisect
. You learned how, via the binary search algorithm,git bisect
can help you quickly find the root of a specific bug in your codebase.Finally, you learned how to remediate your code once the bug is found. You used
git revert
to quickly fix the bug,git commit --amend
to change commit messages to improve git history, andgit rebase
to ensure that your commit history is linear and easy to ready after fixing bad commits.In summary, you learned these primary, Git debugging techniques:
-
Find bugs by inspecting Git commit history with
git log
,git show
,git diff
, andgit blame
-
Find bugs fast via
git bisect
-
Revert bad commits via
git revert
-
Alter commit messages with
git commit --amend
-
Use
git rebase
to ensure a readable, linear commit history after fixing bugs
From here, you can be confident when it comes to integrating
git bisect
and other debugging techniques into your Git workflow. I recommend that you continue your journey learning Git by pursuing both video courses and more guided code labs here, at Pluralsight. -
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.