- Lab
- Core Tech
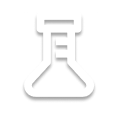
Guided: Creating a Word Guessing Game Using Python
This code lab is designed to boost your skills in Python development while writing a fun word game. Through a sequence of guided steps, you'll be introduced to the mechanics and logic of the word guessing game. You'll work through building the game's core functionalities, such as generating words, capturing and validating user guesses, and providing feedback. By the end of the lab, you will have successfully constructed a fully operational word game in Python!
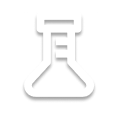
Path Info
Table of Contents
-
Challenge
Introduction and Rules of the Word Guessing Game
Hello there! 🎉 Welcome to this Word Guessing code lab.
What is a Word Guessing Game?
It is a word game where you have to guess a 5-letter word. You get six attempts to do so. After each guess, you'll receive feedback for each letter in your guess. A letter can be in the correct position, present but in a different position, or not in the word at all.
Rules of the Game:
- Five-Letter Words Only: Your guesses, as well as the word you have to guess, should always be 5-letter words.
- Feedback Mechanism: After each guess, each letter in your word will be evaluated:
- The letter shows: The letter is correct and in the right position.
- A ? is displayed: The letter is present in the word, but in the wrong position in the guess.
- A . is displayed: The letter is incorrect and not in the word.
- Limited Guesses: You only have 6 attempts to guess the correct word. Use them wisely!
- Unique Guesses: You can't guess the same word more than once.
What Will You Build?
During this code lab, you'll build a console-based version of a word guessing game. This will involve:
- Setting up the game: Loading words, picking a random word, and initializing the game board.
- Allowing, validating, and providing feedback to the player's guesses.
- Managing the flow of the game, tracking the number of guesses, and notifying the player of their game outcome.
What Skills Will You Practice?
- File I/O: Reading from text files in Python.
- Randomness: Using Python's
random
module to make choices. - String Manipulation: Parsing and analyzing strings to provide feedback.
- Loop Control: Implementing game loops and ensuring game rules are enforced.
- Functions: You'll split your code up in functions to structure it well.
Let's go: You'll set up the game in Step 2.
-
Challenge
Setting Up the Game
Before you can let players dive into the game, you have to make sure the game is ready. It's much like setting up a board game in real life. You wouldn't start playing chess or Monopoly without first arranging the board and pieces. That's no different for a code game, but how to set it up is a little different.
Let's break down the setup into three main tasks.
Load the List of Five-Letter Words
In order to play this word guessing game, the game needs to have words that can be guessed. For this console-based version, these words are stored in a text file. Before the game begins, you need to load these words into your program. The file is called
five_letter_words.txt
.Python Knowledge:
- File I/O: Python provides built-in functions to read and write files. You'll be using the
open
function with read mode ('r'
). This lets you access the content of the file. To read the content line by line, you can use afor
loop.
Randomly Pick a Word from the List
For every new game of this word guessing game, a random word is chosen from your list as the target word. This ensures that each game is a fresh challenge.
Python Knowledge:
- Randomness: Python's
random
module provides the tools you need. Specifically, thechoice
function can be used to select a random item from a list. #### Initialize the Empty Game Board
The game board is the player's visual guide to track their progress and adjust their guesses. In your version, it'll be a representation using strings, showing the player's guesses and the feedback.
Python Knowledge:
- List Comprehension: Python offers a concise way to create lists. For your board, you'll be initializing a list with default values.
- File I/O: Python provides built-in functions to read and write files. You'll be using the
-
Challenge
Handling Player Input and Providing Feedback
The setup of your game is done! It's time to allow the player to guess. In this step, you'll facilitate player interaction and provide instantaneous feedback on their guesses. This feedback loop is crucial in guiding the player to the correct word. You won't be dealing with the game flow yet, this will happen in the next step.
Allow Players to Input a Guess
In order to play the game, players must have a way to communicate their guesses to the program. This interaction will be achieved through Python's
input
function.Python Knowledge:
- Input Function: The built-in
input()
function in Python allows you to capture textual data typed by the user. It displays a prompt to the user and returns the input as a string. You can pass an argument to this function, this will be displayed to the user.
Validate the Guessed Word
It's very likely that the player won't guess the word correctly right away. You need a mechanism to ensure that the guessed word conforms to the game's rules: it should be five letters long and not a repeat of a previous guess. If the guess is invalid, you'll prompt the player to guess again until a valid word is entered. This happens in the next step.
Python Knowledge:
- String Length: You can determine the length of a string using the
len
function. - Lists & Membership: Python lists can be checked for membership using the
in
keyword. For instance,if word in guessed_words:
checks if value ofword
is a present in theguessed_words
list.
Provide Feedback for Each Letter of the Guess
Possibly the most challenging (and most important!) part of the game play is its feedback mechanism. After every guess, players need to know how close (or far) their guess is from the target word. This feedback guides their subsequent guesses.
Python Knowledge:
- String Iteration: Strings are sequences in Python, and you can iterate over them similarly to lists. This will be helpful in comparing the guessed word to the target word letter by letter.
- String Indexing: Strings can be indexed to fetch a particular character. For instance,
word[0]
retrieves the first character.
- Input Function: The built-in
-
Challenge
Coding the Game Flow
You have all the separate parts. Now it's time to bring everything together and turn it into a game. The core gameplay loop is what turns your collection of functions and tasks into an interactive game.
Loop Through the Game for a Maximum of 6 Guesses
Players have 6 attempts to guess the correct word. You need a structured way to keep the game progressing through these attempts, ensuring players get feedback after each guess.
Python Knowledge:
- Loops: A
for
loop is perfect for tasks that need to be repeated a specific number of times. In this case, you'll iterate up to 6 times, once for each guess. - Variables: Use a simple variable to keep a count of the number of guesses the player has made so far. #### The Game Outcome
At the end of the game, whether the player succeeded or ran out of attempts, they will need to know. Did they manage to guess the word in time, or has this round bested them?
Python Knowledge:
- Conditional Statements:
if
andelse
statements can be used to check conditions and execute specific actions based on those conditions. You can run your Word Guessing Game application by using the Run button or entering thepython3 word_game/game.py
command in the Terminal.
- Loops: A
-
Challenge
Congratulations and Skills Used
Congratulations, you've done it! 🥳 You've built a word guessing game from scratch! Did you play it?
You can run your Word Guessing Game application by using the Run button or entering the
python3 word_game/game.py
command in the Terminal.Skills used:
-
File Handling
-
User Interaction
-
Game Logic and Flow
-
String Manipulation
-
Problem Solving
Well Done!
The skills you've practiced here have a much broader application than just this game. Whether it's creating other games, developing tools, or building applications, the foundational coding practices of Python will get you far. 🐍
Remember, learning to code is as much about persistence as it is about knowing syntax. Keep building, keep learning, and most importantly, keep enjoying the process. 💪
Until next time, happy coding! 🚀💖
-
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.