- Lab
- Core Tech
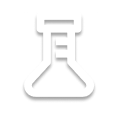
Guided: Creating a Vue 3 Animation Using CSS Transitions
Dive into Vue 3 animations! Learn the essentials of crafting eye-catching animations to enhance user experience. This Guided Code Lab covers Vue 3's animation system, exploring its built-in <transition> component.
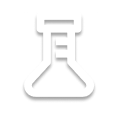
Path Info
Table of Contents
-
Challenge
Introduction
In this Vue guided lab, you have been given a demo app called Globomantics Doc Tracker. Launching the app will display a short list of documents that have an expiration date (i.e., passports, driver licenses, ID cards, etc.). Your task in this lab is to make the app visually more appealing by adding animations.
Animations are a way to make your application's UI more appealing, and capture the user's attention. Animations are also great for making a long-lasting, profound, and positive impact on your users.
The idea is not to animate everything within your application, but just the parts that are essential for the user to have a positive experience when using the application.
The guided steps in this lab will review most of the topics related to implementing transitions and animations using Vue 3. Tasks can be validated by clicking the checkboxes next to them.
If you are stuck or would like to compare solutions, a
solution
directory has been provided for you. Within thesolution
directory, you'll find a subfolder for every task. For example, the solution toTask 2
can be found undersolution/task02
file.You can launch the app at any time during the lab by doing the following:
-
Click the Run button at the bottom right of the Terminal tab to run the
npm run dev
command. -
View the web page by clicking on the internal Web Browser tab, and then use the arrow icon (Open in new browser tab) in the browser to open the web application in a new tab.
info> Important:
- By default, the app uses port 3000. If for some reason, the app doesn't run, it could be that port 3000 might be taken by another process. In that case, you may run thefuser 3000/tcp --kill
command in the Terminal to free up port 3000, and then run the app again.
- Within the FILETREE you can find the project files. TheRun.txt
file contains the commands to reset port 3000 and run the app using the Terminal. -
-
Challenge
Adding a Transition
A
transition
is nothing more than a Vue component that can be wrapped around another component, allowing the "wrapped up" component to become animated.Imagine you have the following:
<div> ... </div>
You can easily animate the above code in Vue by wrapping the
transition
component around it and giving it a name, for exampleanimated
:<transition name="animated" appear> <div> ... </div> </transition>
Take a look at the
Home.vue
file found in the code editor to your right and complete the following task. In the next steps, you'll bring this transition to life by adding the CSS animation classes. -
Challenge
Entry Transitions
Wrapping the component that you want to animate around a Vue transition component is the first step of creating an animation with Vue.
Now, you need to add an animation class to the transition component you just added.
Given that the transition's component name is
b
, in other words:<transition name="b">...</transition>
In this step. you will create different CSS classes that you can add to the
style
section of theHome.vue
file found within thesrc/views
folder, as follows:<style> << CSS classes go here >> </style>
CSS Class:
b-enter-from
This CSS class will create the animation when the transition component "enters from" a non-animated state and later transitions to an animated state. You can think this CSS class as the style that will be applied to the transition component at the beginning of the animation.
The CSS class needs to begin with the same name as the transition component, followed by the animation event (
enter-from
), so in this case:b-enter-from
.This CSS class will contains two properties:
opacity
andtransform
. The value ofopacity
should begin with0
and the value oftransform
indicates the actual type of animation that you'll perform. In this case, you want to create a vertical animation movement.To create a vertical animation movement, you can use
translateY
and pass the number of pixels that you want the animation to move.Having said this, your animation class should look something like this:
.b-enter-from { opacity: 0; transform: translateY(10px); }
This is an important and crucial step in the animation process, because it describes what the animation's initial state will be. ###### CSS Class:
b-enter-to
This CSS class will create the animation when the transition component "enters to" an animated state. You can think this CSS class as the style that will be applied to the transition component at the end of the animation.
The CSS class needs to begin with the same name as the transition component, followed by the animation event (
enter-to
), so in this case:b-enter-to
.This CSS class will contain two properties:
opacity
andtransform
. The value ofopacity
should be1
and the value of transform indicates the actual type of animation that you'll perform. In this case, you want to create a vertical animation movement.To create a vertical animation movement, you can use
translateY
and pass the number of pixels that you want the animation to move, from its initial position (10
) to its final position (0
).Having said this, you animation class should look something like this:
.b-enter-to { opacity: << The opacity value goes here >> transform: translateY(<< The final position goes here >>); }
This is an important and crucial step in the animation process, because it describes what the animation's final state will be.
Remember that the animation began with the following state:
.b-enter-from { opacity: 0; transform: translateY(10px); } ``` Now that you have added the ```.b-enter-from``` and ```.b-enter-to``` classes to the ```<styles>...</styles>``` section of the ```src/views/Home.vue``` file, you need to indicate what type of animation you want ```<transition name="b">...</transition>``` to have. To do that, you need to add a ```.b-enter-active``` class to the ```<styles>...</styles>``` section of the ```Home.vue``` file. ###### CSS Class: `b-enter-active` This class only requires a single property called ```transition``` which describes three things: * a) That it applies to `all` elements within the ```<transition name="b">...</transition>``` component. * b) That it will take 1.5 seconds (```1.5s```) to execute. * c) That it will appear as "easing-in" place (```ease-in```). It's important to know that you may use other values for the duration of animations in Vue, besides 1.5 seconds (```1.5s```). This specific value is just for this animation example. Besides, the ```ease-in``` option, the ```ease-out```, and ```ease``` options are also available. This specific ``ease-in``` option is just for this animation example. This property should be described as a one-liner property as follows: ```vue transition: a) b) c)
You will add the
.b-enter-active
class after the.b-enter-to
class. Make sure you add thetransition
property to it as follows:.b-enter-active { transition: a) b) c) }
This step is equally important because it is here that you specify what happens between the animation's initial state and the animation's final state. So this step is where you actually define what the animation does. This is the animation!
Obviously, you must replace the values of
a)
,b)
, andc)
. By the end of this step, if you execute the app, you should see that the input section now has a "sliding-in" animation. -
Challenge
Exit Transitions
Given that the transition's component name is
b
, in other words:<transition name="b">...</transition>
You will need to create other CSS classes that you can add to the
style
section of theHome.vue
file.CSS Class:
b-leave-from
This CSS class will create the animation when the when the transition component "leaves from" an animated state to an non-animated state. You can think this CSS class as the style that will be applied to the transition component before the components starts to exit its animated state.
The CSS class needs to begin with the same name as the transition component, followed by the animation event (
leave-from
), so in this case:b-leave-from
.This CSS class will contains two properties:
opacity
andtransform
. The value ofopacity
should be1
and the value of transform indicates the actual type of animation that you'll perform. In this case, you want to create a vertical animation movement.To create a vertical animation movement, you can use
translateY
starting at this position: (0
).Having said this, your animation class should look something like the following:
.b-leave-from { opacity: << The opacity value goes here >> transform: translateY(<< The position goes here >>); }
Remember that the animation now has the following state:
.b-enter-to { opacity: 1; transform: translateY(0); } .b-enter-active { transition: all 1.5s ease-in; }
You need to move away from the current state, starting from the current state. In other words,
.b-leave-from
should behave like.b-enter-to
. ###### CSS Class:b-leave-to
This CSS class will create the animation when the when the transition component "leaves to" a non-animated state, from an animated state. You can think this CSS class as the style that will be applied to the transition component when the animation finishes.
The CSS class needs to begin with the same name as the transition component, followed by the animation event (
leave-to
), so in this case:b-leave-to
.This CSS class will contains two properties:
opacity
andtransform
. The value ofopacity
should begin with0
and the value of transform indicates the actual type of animation that we'll perform. In this case, you want to create a vertical animation movement.To create a vertical animation movement, you can use
translateY
and pass the number of pixels that you want the animation to move.Having said this, your animation class should look something like the following:
.b-leave-to { opacity: 0; transform: translateY(10px); } ``` Now that you have added the ```.b-leave-from``` and ```.b-leave-to``` classes to the ```<styles>...</styles>``` section of the ```src/views/Home.vue``` file, you need to indicate what type of animation you want ```<transition name="b">...</transition>``` to have. To do that, you need to add a ```.b-leave-active``` class to the ```<styles>...</styles>``` section of the ```src/views/Home.vue``` file. ###### CSS Class: `b-leave-active` This class only requires a single property called ```transition``` which describes three things: * a) That it applies to `all` elements within the ```<transition name="b">...</transition>``` component. * b) That it will take 1.5 seconds (```1.5s```) to execute. * c) That it will appear as "easing-in" place (```ease-in```). This property should be described as a one-liner property as follows: ```vue transition: a) b) c)
You will need to add the
.b-leave-active
class after the.b-leave-to
class. Make sure you add thetransition
property to it as follows:.b-leave-active { transition: a) b) c) }
Obviously, you must replace the values of a) b) and c). By the end of this step, if you execute the app, you should see that the input section now has a "sliding-out" animation.
-
Challenge
Entry and Exit Transition Combinations
Transition Combinations:
b-enter-to
+b-leave-from
You may have noticed in the previous steps and tasks that some classes, although they have different names, actually do the same thing. For example,
b-enter-to
andb-leave-from
have the same properties:.b-enter-to { opacity: 1; transform: translateY(0); }
.b-leave-from { opacity: 1; transform: translateY(0); }
So you may be wondering, why not combine them together instead of duplicating this logic? Well, that's exactly what this task is about! ###### Transition Combinations:
b-enter-from
+b-leave-to
You may have noticed in the previous steps and tasks that some classes, although they have different names, actually do the same thing. For example,
b-enter-from
andb-leave-to
have the same properties:.b-enter-from { opacity: 0; transform: translateY(10px); }
.b-leave-to { opacity: 0; transform: translateY(10px); }
So you may be wondering, why not combine them together instead of duplicating this logic? Well, that's exactly what this task is about! ###### Transition Combinations:
b-enter-active
+b-leave-active
You may have noticed in the previous steps and tasks that some classes, although they have different names, actually do the same thing. For example,
b-enter-active
andb-leave-active
have the same properties:.b-enter-active { transition: all 1.5s ease-in; }
.b-leave-active { transition: all 1.5s ease-in; }
So you may be wondering, why not combine them together instead of duplicating this logic? Well, that's exactly what this task is about! By the end of this step, if you execute the app, you should see that the input section now has "sliding-in" and "sliding-out" animations.
-
Challenge
Animated Notification
The next thing you are going to do is animate the notification message that appears when you click on the Add document button and you haven't entered any details.
To get you started, open the
src/views/Home.vue
file and place the following classes within the<styles>...</styles>
section:.notification-leave-to { opacity: 0; transform: translateY(-80px); } .notification-leave-from { opacity: 1; transform: translateY(0); } .notification-enter-active { animation: shake 0.8s ease; } .notification-leave-active { transition: all 0.5s ease; }
The reason you need to copy these classes and paste them into the
<styles>...</styles>
section of thesrc/views/Home.vue
file is because you've covered what these classes do in the previous tasks. The only difference, is that the transition's name is nownotification
.Next you need to wrap the
<Notification v-if="showNotification" />
component around a transition, as follows:<transition name="notification"> <Notification v-if="showNotification" /> </transition>
info> Note: You can also open
solution/task13/Home.vue
and just copy the contents of this file and overwrite the contents ofsrc/views/Home.vue
, if that's easier.You'll notice in the code you copied from above that the
.notification-enter-active
class uses theshake
effect:.notification-enter-active { animation: shake 0.8s ease; }
The
shake
effect is what you'll use to give theNotification
component a unique shaking animation in the next step. -
Challenge
Shake Animation
Initial Shake
In order to create the shaking effect, you have to create a
@keyframes shake { ... }
class within the<style>...</style>
section of thesrc/views/Home.vue
file.This can be done as follows:
@keyframes shake { 0% { transform: translateY(-40px); opacity: 0; } ... 100% { transform: translateX(0); } }
The
shake
effect is created by going from0%
to100%
, where each step includes atransform
animation.Initially, you want to indicate what happens when you reach
45%
and55%
, which is what you will complete in this next task.Just to be clear, the initial shake is more like a vertical downward movement, rather than a shake itself. The actually shaking will be done in the next task, when you add a horizontal movement. ###### Final Shake
In order to finish creating the shaking effect, you have to finish creating the
@keyframes shake { ... }
class within the<style>...</style>
section of thesrc/views/Home.vue
file.This can be done as follows:
@keyframes shake { 0% { transform: translateY(-40px); opacity: 0; } 45% { transform: translateY(0); opacity: 1; } 55% { transform: translateX(15px); } ... 100% { transform: translateX(0); } }
The
shake
effect is created by going from0%
to100%
, where each step includes atransform
animation.Now, you want to indicate what happens when you reach
65%
and80%
, which is what you will complete in this next task. By the end of this step, you should see that the input section is animated when you run the application. If you click on the Add Document button, you should see a notification with a basic shaking animation. -
Challenge
Final Result
Congratulations! You have completed this lab.
You should now have a
Home.vue
that looks like the one found undersolution/task15/Home.vue
.You created an animation of the main input section of the Globomantics Doc Tracker application, and also an animated
Notification
component that has a shaking effect.When you run the application the two input fields and the button to add a document to the list should be animated when the application loads. The
Notification
should also appear to "shake".After reviewing these principles and guidelines, you should now have a foundation to build your own animations in Vue 3.
Now that you have completed this lab, you should see that the input section is animated when executing the app. If you click on the Add Document button, you should see a notification with a sliding animation, followed by a shaking animation before the notification disappears.
If you would like to dive deeper into Vue 3 Animations, feel free to check out the following course, and add additional features to the code base:
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.