- Lab
- Core Tech
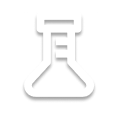
Guided: Control Flow and Function Implementation in C
In this Guided Code Lab, you will build a randomized Rock, Paper, Scissors game while practicing core programming concepts in C. You’ll learn how to use control flow, write conditional logic, and create functions to organize your code. By the end, you’ll have a fully interactive game that responds to user input and generates random computer moves.
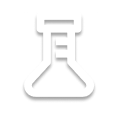
Path Info
Table of Contents
-
Challenge
What is Control Flow
In this lab, you will use conditionals and functions to control the flow of your program. Control flow refers to the order in which individual statements, instructions, or function calls are executed or evaluated.
You can have sequential, line-by-line execution like this:
int main() { printf("Step 1: You are an adult\n"); printf("Step 2: You need to pay taxes\n"); return 0; }
Output:
Step 1: You are an adult Step 2: You need to pay taxes
Conditionals can control what line gets executed based on the condition. Notice below that only one print statement is run based on which conditional is true.
int main() { int age = 20; if (age >= 18) { printf("You are an adult.\n"); } else { printf("You are a minor.\n"); } return 0; }
Output:
You are an adult
Use conditionals and functions to control the flow of your program as you create a game called Rock Paper Scissors. Control flow will help:
- Take player input
- Compare choices
- Decide the winner
How to play Rock - Paper - Scissors
Rock - Paper - Scissors is a quick hand game played between 2 people. Each person secretly picks one of three things:
- ✊ Rock
- ✋ Paper
- ✌️ Scissors
Then both players show their choice, using their hand, at the same time.
How to win:
Each option can beat one and lose to one:
- Rock beats Scissors (Rock smashes Scissors)
- Scissors beats Paper (Scissors cuts Paper)
- Paper beats Rock (Paper covers Rock)
If both players choose the same, it is a tie.
Recreate the game for the computer by letting the user pick Rock, Paper, or Scissors from the command line. The computer's pick is randomly generated. Then see who wins.
info> If you get stuck on a task, you can find solution code for each task in the
solution
folder in your filetree. -
Challenge
Let's create a Rock - Paper - Scissors game
Add your code to the RockPaperScissors.c file in the editor. Check your progress with the validate button.
In the code editor, there is a solution folder with solution code in case you get stuck.
If at any point you also want to compile and run your program, you can do that from the Terminal below the code window.
First, compile your program with:
gcc RockPaperScissors.c -o RockPaperScissors.out
Then you can run your program with:
./RockPaperScissors.out
-
Challenge
Validate Input with a Conditional
It's time to use your first conditional, or if statement. Here is an example of a simple if statement:
int tickets = 10; if (tickets == 10) { printf("Good luck in the raffle!"); } else { printf("You need 10 tickets to play!"); }
This would print the following:
Good luck in the raffle!
Multiple conditions can be linked with the OR operator, denoted by
||
, or the AND operator, denoted by&&
.Here is an example of checking multiple conditions. Assume you want the user to enter a decimal or float, between 1 and 10. Your conditional would look like this:
printf("Enter a decimal between 1 - 10: "); float userChoice; if (scanf("%d", &userChoice) != 1 || userChoice < 1 || userChoice > 10) { printf("Invalid input. Please run the program again and enter a decimal between 1 and 10.\n"); exit(1); // Exits the program }
-
Challenge
Refactor code into `readUserChoice()` function
So far, most of the code is inside the
main()
function. But code for specific tasks should live in their own functions. For instance, you can move what you have so far inmain()
into its own function calledreadUserChoice()
.Why? Well, here are some the benefits of creating functions for specific tasks:
- ✅ Reusability -- You can call the function anytime you need user input. Maybe you let the user play the game infinite times in a row and call
readUserChoice()
in a loop. - ✅ Readability -- Keeps main() short and simple
- ✅ Organization -- Each function does one clear job
- ✅ Testability -- Easier to debug and improve later
- ✅ Reusability -- You can call the function anytime you need user input. Maybe you let the user play the game infinite times in a row and call
-
Challenge
Create `generateComputerChoice()`
Now that the user's choice has been saved by calling the
readUserChoice()
function, you need to generate the computer's choice to figure out who wins. You should generate the computer's choice in a new function. -
Challenge
Determine the winner
Congratulations on completing your program!! 🎉 Your game is complete, feel free to play it out in the terminal.
Remember you can compile your program with:
gcc RockPaperScissors.c -o RockPaperScissors.out
Then you can run your program with:
./RockPaperScissors.out
You used control flow and functions in a few different ways:
- Conditionals decide how your game progresses.
- Did the user have invalid input? Then exit.
- Who won? Compare user and computer choices to decide the winner.
- Functions organize the code into specific tasks.
- And the program flows into each function when it's called.
Congratulations again!! 👏👏 You did an awesome job sticking through the tutorial — this kind of continued learning is exactly what makes great developers 👏👏
- Conditionals decide how your game progresses.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.