- Lab
- Core Tech
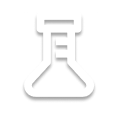
Guided: Building an e-commerce Platform in Laravel - Part 2
This lab will guide you through creating a sophisticated e-commerce platform. Learn how to integrate the category system in your web shop and how to let users make orders. This is the second part of the two-lab series, where you’ll set up the basic Order and Category models and allow authorized users to place their products in those categories and use the Order model to make product orders on the site. In this lab you will create a cart system and remember the user's orders. You will also learn how to implement categories which will help you create a filter for products on your e-commerce platform.
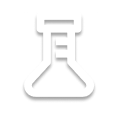
Path Info
Table of Contents
-
Challenge
Introduction
In this Guided Code Lab, you will continue working on a simple e-commerce prototype web shop. It is strongly recommended that you finish the Part 1 of this lab first, before starting this one. This lab builds on the first one by adding functionality for users to filter products by categories and place orders.
Each task can be validated individually by clicking on the Validate button next to it.
If you are stuck, you can always check out the details section of the task or the
solutions
directory. The task will give you feedback if you don't complete it correctly. A failed task will have one or more failed tests under the Checks section of the task. You can look the output of these failed tests to see what went wrong. Thesolutions
folder at the top of the workspace contains the final state of the application after finishing the lab.The starting point of the lab is a Laravel application named "shop". The current directory of the built-in Terminal will be set to the
shop/
directory.You can use the Terminal to run the development server with the Artisan CLI or to run other Artisan commands. The Run button will automatically start the Artisan development server.
info> Don't forget to migrate the database before running the server for the first time with
php artisan migrate
.There is also a web browser that you can use to access the development server endpoints and see the app. So when you get to the point where you need to run the server, you can open this {{localhost:8000}} link and see the running dev server.
The templates that you'll need are already prepared for this lab and placed inside of the
views/
directory. The application will use the MySQL server that is already running in the background. Other than these changes, the "shop" project is basically the same application you finished building in the first part of this lab series. However, as you will see, some things are changed and updated to better cater to the needs of this specific lab. Some view templates are changed and additional routes and controllers are added, but you will be aware of them when you get to the specific tasks.Click on the Next step arrow to get started!
-
Challenge
Creating the Category Model
You can start up the existing Laravel application by running
php artisan serve
in the Terminal or clicking on the Run button and opening the Shop application.On the home page, you will see the new Filter by category dropdown, which lets you filter products by category:
However, these categories are just hardcoded placeholders. You need to implement this feature in the app. The
Category
model is now ready, however, eachProduct
should belong to a specificCategory
. You need to define that relationship between these two models. TheCategory
model should now be connected to theProduct
model. Now it's time to create some basic categories and add them to products. -
Challenge
Seeding the Category Data
In most web shops, the categories will be defined by an administrator, not by users. You should create a seeder for categories to store some default categories in the database. After that, you can try the seeder by rebuilding the database, just in case there are some old products, and seeding it with the following commands:
php artisan migrate:fresh php artisan db:seed
You should now have a fresh new database with some default categories. A user should be able to select the category when creating a new product. You need to add the category option to the product creation page. The template
resources/views/products/form.blade.php
already contains the category dropdown, but you need to provide the categories to thecreate
action and store them in thestore
action of theProductController
. You're almost done with implementing categories! Each product should now also be related to a specific category.In the next step, you will add some finishing touches and make the categories filter functional.
If you want, you can try to create a few products now with the appropriate category. Start up the server, register, login, and create a couple of products. You can start the server from the Terminal and see it here: {{localhost:8000}}
If you don't see any categories in the dropdown, make sure to seed the database:
php artisan db:seed
-
Challenge
Filtering Products with Categories
Before implementing the filter, you should update the product's show template in
resources/views/products/show.blade.php
. The filter on the home page needs to get all of the available categories from the database. The filter dropdown lets you select a specific category and once you click on the Filter button, it will send the id of that category as a request query parameter. You need to receive that query parameter and only provide products with that specific category.Take a look inside of the
index
action of theProductController
, which is also the main home route of the whole application, inside of theapp/Http/Controllers/ProductController.php
file.You can see that there are two values passed to the template, aside from the products:
categories
andselectedCategory
. Both of the values are hard-coded, this needs to be changed. And that's it! Congratulations, you just implemented categories in your web shop! Filter should only show the products that belong to the selected category. -
Challenge
Defining Orders and Order Items
Once you start up the server and login, you might notice that there is a new menu item on the right. It is a cart system that allows you to add existing products to the cart and it is implemented with Alpine.js. You don't need to know how it works because this course is not about front-end technicalities like JavaScript and HTML markup.
The products you add to the cart are placed inside of the browser's local storage and once you click the Place Order button, the script will take all of that data and pass it to a specific endpoint as a form input. More on that later, for now, you need to create tables in the database that will actually store these orders. Each
Order
should belong to theUser
that placed the order in question, butOrder
should also be connected to all of theProducts
that were added to the cart. So everyOrder
belongs to someUser
and everyOrder
should also be related to all of the products you placed in the cart. You cannot simply create ahas many
relationship between orders and products because the same product can belong to multiple orders. It is also possible that each order can have a different quantity of the same product. You need to create some kind of an intermediary table to connect orders and products. A model that will connect them can be namedOrderItem
, for example, where each item represents a relationship between the product and a specific order. After defining these two models and their corresponding migrations, you can migrate the changes to the database:php artisan migrate
.Before you can use these models in your application, you need to define the relationship between them in their model classes. The models should now be ready! In the next step, you will use them to actually store orders in the database.
-
Challenge
Placing Orders from the Cart
The cart will store all of the added products as a JSON list, where each product is represented by an object. Each object contains the product's id and current price, together with the quantity you selected to buy. When you click Place Order, the JSON data will be sent to the
placeOrder
action of theCartController
. You can check it out inside of theapp/Http/Controllers/CartController.php
file.The existing
placeOrder
function already does something. It takes the JSON from the request's input and it decodes it inside of the$cartItems
. It also calculates the total price of the order, and finally, it redirects the user back to the home page. But nothing actually gets stored to the database and that is where you come in! The orders from the cart should now be stored in the database. There is also a new My Orders page that you can find under the Account menu item, which should be in charge of showing all of the orders made by the logged in user.The rendering of this template is implemented inside of the
orders
action of theUserController
class. For now, this page is empty, but you should pass all of the orders made by the current user to the template, together with all of the information about the products and items connected to that order. All of the orders should now be visible on the My Orders page. Congratulations on finishing this lab and your simple web shop in Laravel!Here are some suggestions of what you could implement to make this web shop even better:
- Notice that the delete functionality for the products is removed in this lab. That is because this would add additional complexity to the app, since products are now tied to orders. You could implement Laravel's soft delete functionality to allow users to remove products without actually removing them from the database.
- A placeholder message for when there are no products in a specific category could actually contain the selected category name.
- You could also allow users to change the category of the existing product by editing the edit and update action of the product controller.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.