- Lab
- Core Tech
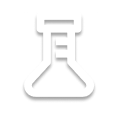
Guided: Building a Budgeting Application in Vue 3
This hands-on lab is designed to help you practice your understanding of core Vue concepts including rendering, data binding, event emissions, and parent-child component interactions. A series of guided steps will give you a walkthrough on how to apply your knowledge of these concepts to build multiple related Vue components and make them interact with each other. By the end of the lab, you will have demonstrated your ability to build a functioning multi-component Vue application.
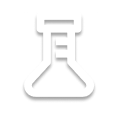
Path Info
Table of Contents
-
Challenge
Introduction
In this lab, you will be completing a simple budgeting application using the Vue front-end framework. You will be working with four files, which are
BudgetForm.vue
,BudgetItem.vue
,BudgetList.vue
, andApp.vue
. Completing this lab will involve the usage of essential Vue concepts such as event emissions, data binding, and interactions between parent and child components.The app can be run at anytime by clicking the Run button or by running the
npm run dev -- --host
command in the Terminal. You can view the application's webpage in the Web Browser tab by refreshing the page. -
Challenge
Complete the Budget Form
First, you will need to complete the
BudgetForm
component. This component contains the form and input elements that allow the user to specify a total budget amount and the cost of each item.Each instance of a component tracks its own state, or internal data, aptly stored within the
data()
function. This function returns an object containing the reactive (triggers an update when changed) state data. Components can emit data to other components. It's good practice to declare any emitted events through theemits
property. Themethods
property of the component details all of the functions it is able to perform. For theBudgetForm
component, it will need to have asubmitBudgetAmountForm()
andsubmitItemForm()
methods which will be responsible for emitting the user input data to be displayed. With these done, now you will need to complete the form element for the component in the htmltemplate
. Thebutton
elements have already been defined for you, but you will need to complete theform
andinput
elements with data-bindings. -
Challenge
Complete the Budget Item
With the
BudgetForm
component done, a user can now input a total budget amount as well an item that has a description and amount. Now, you will need aBudgetItem
component to display the item that a user inputs.The important thing to take note of here is the use of
props
. Data that is given to a child component for its use from a parent component is stored in theprops
field of the component, much likeemits
andmethods
are. Now thatprops
has been defined and takes anitem
, you can use theitem
to display its data within thetemplate
. This is done with declarative rendering, wherein data is enclosed within double brackets such as{{ exampleData }}
. When this data is changed, the component will update to display the new data. -
Challenge
Complete the Budget List
Now that
BudgetItem
is completed, you will need a component that displays all of them. This is whereBudgetList
comes into play. As you may notice at the top ofBudgetList.vue
, theBudgetItem
component has been imported.You will need to make it a child component of the
BudgetList
so that it can be used by theBudgetList
. LikeBudgetItem
, theBudgetList
will also needprops
because it needs to receive a list of objects containing adescription
anditemAmount
. It will then pass the data in these objects to eachBudgetItem
that it renders. Lastly, render theBudgetItems
as a single element within thetemplate
section. Although it's a single element, it will actually display all theBudgetItems
if you use thev-for
directive to iterate through theitems
prop. -
Challenge
Putting it all together in App
Now that all of the individual components have been completed, it's time to put them all together within
App.vue
. There's nothing new going on here and will mostly follow the same development paradigms seen in the previous steps. Now that the components are defined, you will need to create adata
property forApp
. Remember that theBudgetForm
is a child component that sends data to theApp
parent, and theBudgetList
is a child component that receives data from theApp
parent. Now you will needmethods
forApp
to perform based on the data it receives from itsBudgetForm
child component to dynamically update any changes to thetotalBudget
amount as well as any additional items to be budgeted. Lastly, you will need to render these child components withinApp
. Since theBudgetForm
component will be emitting data to theApp
, you will need model bindings(two-way) that are prefixed with an@
. TheBudgetList
component will be receiving data from app, so it will need an attribute binding(one-way) prefixed with an:
. With these steps completed, your application should be all set and ready to go! Refer back to the introduction on how to Run the application and see it for yourself in the Web Browser.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.