- Lab
- Core Tech
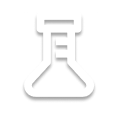
Guided: Building a Book Catalog App with Spring Boot 3
This hands-on lab is designed to help you complete a book catalog application with Spring Boot 3. A series of guided steps will give you a walkthrough on how to implement some of the core layers that compose a Spring Boot application such as the services in a business layer along with the controller and views of the presentation layer. By the end of the lab, you will have demonstrated your ability to build a fully functioning book catalog capable of adding, editing, and removing books within its inventory.
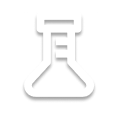
Path Info
Table of Contents
-
Challenge
Introduction
The purpose of this lab is to guide you through the basics of building an application in Spring Boot 3. This lab will cover the views and controller of the presentation layer as well as the service used in the business layer. Since you will be working with a local JSON file for data, the persistence and database layers will be outside the scope of this lab.
You can run the application after completing the lab by clicking the Run button in the terminal or by running the command ./gradlew bootRun. Once your application is running, you can view it by switching to the Web Browser tab and changing the port to 8081 instead of 8080.
-
Challenge
Implementing the Service
The first component of this application you will need to complete is the
BookCatalogService.java
. This service contains all of the logic and functions your application will need to perform such as adding, editing, and deleting books.The boilerplate code to the service already instantiates the catalog as a list of book data from a json file and sorts it by
id
for you. Next, you will need to implement thebinarySearch()
function to search for the index of a book in the book catalogue using the binary search algorithm.Details
- Set a
start
as the first index of the list. - Set an
end
as the last index of the list. - While
start
is less than or equal toend
, do steps 6-9. - Calculate
mid
as theaverage
ofstart
andend
. - If the value at index
mid
is equal to the target value, returnmid
. - If the value at index
mid
is greater than the target value, setend
tomid - 1
. - If the value at index
mid
is less than the target value, setstart
tomid + 1
. - Return
-1
outside of thewhile
loop
Details
- Create a new
index
value that is the size ofthis.books
incremented by 1. We increment by 1 because the catalog is 0 indexed but the first book has an id of 1. - Create a new
Book
using this index and the parameters for the method. - Add the created
Book
tothis.books
with theadd()
method.
Details
- Retrieve the
Book
to be modified fromthis.books
using theget()
method- The index for the
get()
method is thegetId()
of theupdatedBook
parameter decremented by 1(to account for 0-indexing).
- The index for the
- Update the retrieved
Book
with the values from theupdatedBook
.- Use the
setTitle()
,setAuthor()
,setGenre()
,setNumberOfPages()
, andsetPublishedDate()
methods of theBook
class to update the values of the retrievedBook
. - Use the
get
versions of these methods on theupdatedBook
to retrieve to values to use.
- Use the
Details
- Use the
binarySearch()
method you implemented with theid
parameter to find theindex
of the book to be removed. - If the
index
is found(not equal to -1) remove the book at thatindex
fromthis.books
. Optionally, you can add a print statement to confirm that the book was removed. - If the
index
is not found, don't modifythis.books
. Optionally, you could add a print statement that specifies a book with the givenid
could not be found.
- Set a
-
Challenge
Implementing the Controller
Now you need to complete the controller for the application, which is located in
BookController.java
. The controller is responsible for handling HTTP requests from the apps HTML views by using the methods implemented inBookCatalogService
. It then passes back data to the views to be displayed for the user.The first endpoint you will need to implement is the
homePage()
.Details
- Add a
@GetMapping()
annotation above the method signature. - Give the
@GetMapping()
a"/"
for its value. - In the method body, retrieve the list of books using the
getAllBooks()
method from thebookService
. - Use the
model.addAttribute()
method to bind your list of books to the view. Give this attribute name something like"books"
. - Return the string
"home"
, which is the name of the HTML file to be rendered at this endpoint.
Details
- Add a
@GetMapping()
annotation above the method signature. - Give the
@GetMapping()
a"/edit-book-page/{id}"
for its value. - In the method body, retrieve the list of books using the
getAllBooks()
method from thebookService
. - Use the
binarySearch()
method frombookService
to retrieve the index of the book with theid
parameter. - If an invalid index is found (-1), return the string
"redirect:/"
to redirect back to the home page. - Otherwise, use the index to
get()
the specifiedBook
from the list of books. - Create a string by using
format(DateTimeFormatter.ISO_LOCAL_DATE)
on thepublishedDate
of the retrievedBook
. - Use
model.addAttribute()
to bind the retrieved book to the model. - Use
model.addAttribute()
to bind the formatted date string to the model. - Return the string
"edit-book"
to render theedit-book
page.
Details
- Add a
@PostMapping()
annotation above the method signature. - Give the
@PostMapping()
a"/update-book"
for its value. - In the method body, call the
updateBook()
method from thebookService
on theeditedBook
parameter. - Return the string
"redirect:/"
which will redirect back to the home page after updating the book.
Details
- Add a
@GetMapping()
annotation above the method signature. - Give the
@GetMapping()
a"/add-book-page"
for its value. - In the method body, return the string
"add-book"
which will render the HTML page for adding a new book to the catalog.
Details
- Add a
@PostMapping()
annotation above the method signature. - Give the
@PostMapping()
a"/add-new-book"
for its value. - In the method body, call the
addBook()
method from thebookService
using all the parameters supplied in the method signature. - Return the string
"redirect:/"
which will redirect back to the home page after adding a new book.
Details
- Add a
@PostMapping()
annotation above the method signature. - Give the
@PostMapping()
a"/delete-book/{id}"
for its value. - In the method body, call the
removeBook()
method from thebookService
with theid
parameter. - Return the string
"redirect:/"
which will redirect back to the home page after removing the book.
- Add a
-
Challenge
Implementing the View
Lastly, you will need to add some finishing touches to the views layer. In this application, the Thymeleaf template engine from Spring is used to render HTML files such as
home.html
,add-book.html
, andedit-book.html
. The controller endpoints you implemented in the previous step dictates which of these HTML files the user is currently viewing at any given time.While the HTML files are mostly complete, you will need to add a few Thymeleaf specific attributes to certain elements that will dynamically render data from the catalog. Let's start with
home.html
.Details
- In the second
<tr>
element, add ath:each
binding set to each book of the${books}
model attribute. It's done the same way as aforEach
loop. - In each
<td>
sub element, use theth:text
binding and bind it to the respective property of theBook
class.- For example, the first
<td>
would have"${book.getTitle()}"
for the value ofth:text
. Follow the same order as shown in the first<tr>
element.
- For example, the first
Details
- In the
<form>
element, add ath:action
binding set to the"@{/add-new-book}"
route. - In the same
<form>
element, add amethod
binding set to"post"
.
Details
- In the
<form>
element, add ath:action
binding set to the"@{/update-book}"
route. - In the same
<form>
element, add amethod
binding set to"post"
.
- In the second
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.