- Lab
- Core Tech
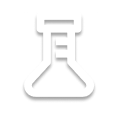
Guided: Build a Spaced Repetition System in PHP
Welcome to the Code Lab Guided: Build a Spaced Repetition System in PHP. This lab provides an immersive experience for beginner to intermediate web developers who want to expand their knowledge in PHP, PostgreSQL, and the MVC (Model-View-Controller) architecture. Through hands-on learning, you will build an interactive web application that helps users learn more effectively using spaced repetition. By the end of this lab, you will have a solid understanding of database interactions, user authentication, session management, and implementing algorithms for spaced repetition, preparing you to tackle real-world challenges in web development.
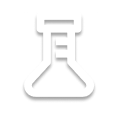
Path Info
Table of Contents
-
Challenge
Introduction
Creating a Spaced Repetition System with PHP
A Spaced Repetition System (SRS) is a proven technique for improving long-term retention of information by scheduling reviews of learned material at increasing intervals. This method leverages the psychological spacing effect, where information is more effectively memorized when reviews are strategically spaced over time. In this project, we will implement an SRS using PHP, enabling users to create, manage, and review flashcards in an optimized way. By combining a structured review schedule with dynamic intervals based on user performance, we can ensure that users reinforce their knowledge efficiently, reducing the time spent on material they already know well while focusing on areas where improvement is needed.
Steps to Build the Spaced Repetition System
1. Set Up the Database
We will begin by creating the necessary database structure to store user data, flashcards, and review schedules. The tables will include:
users
: to store user information for multi-user functionality.cards
: to store flashcard data including questions, answers, and review intervals.progress
: to track each user's progress on each card, including review counts and the next review date.user_cards
: to associate users with the flashcards they are reviewing.
2. Establish Database Connection
Next, we will establish a connection to the MySQL database using PHP's PDO class. This will enable secure and efficient communication with the database. The connection will be encapsulated in a
Database
class to implement the Singleton pattern, ensuring only one instance of the database connection is used throughout the application.3. Adding a New Flashcard
In this section, we will build the ability for users to add new flashcards to the system, introducing the basic Model-View-Controller (MVC) architecture.
- Model (Card): Handle the database operations for inserting a new card.
- Controller (CardController): Handle form submission and input validation for adding a card.
- View (add_card.php): Provide a form for users to input the question and answer for a new flashcard.
4. Listing All Flashcards
In this section, users will be able to view a list of all flashcards they’ve created. This slice builds on the MVC pattern by retrieving data from the database and displaying it to the user.
- Model (Card): Fetch all flashcards from the database.
- Controller (CardController): Handle the request to list all cards.
- View (list_cards.php): Display the list of flashcards in a table.
5. Associating Cards with a User
In this section, we’ll introduce the concept of user-specific flashcards. Users will be able to add flashcards to their list for review, setting the stage for personalized reviews.
- Model (UserCard): Handle associations between users and flashcards.
- Controller (CardController): Enable users to add flashcards to their own list.
- View (list_cards.php): Add functionality to allow users to add cards to their review list.
6. Reviewing Flashcards
This section introduces the core functionality of spaced repetition, allowing users to review flashcards and mark them as correct or incorrect, which will dynamically update the review intervals.
- Model (UserCard): Track card review progress, calculate next review dates, and update card intervals.
- Controller (CardController): Handle the review process and calculate the next review date based on user performance.
- View (review_cards.php): Display a card to the user for review, allowing them to mark it as correct or incorrect.
7. Tracking User Progress
In this section, users will be able to track their progress, including the number of reviews they’ve completed and their accuracy, displayed in a progress dashboard.
- Model (UserCard): Track and retrieve progress data, such as review counts and correct answers.
- Controller (CardController): Handle requests to view user progress.
- View (progress.php): Display the user’s progress in a table format, summarizing their performance.
8. User Authentication
This final section adds user authentication to the system, allowing multiple users to log in, register, and manage their own sets of flashcards.
- Model (User): Handle user registration and authentication.
- Controller (login.php, register.php, logout.php): Manage user sessions, login, and registration processes.
- View (login.php, register.php): Provide forms for user login and registration.
Conclusion
By following these steps in slices, we will build a fully functional Spaced Repetition System in PHP that supports multiple users and provides an efficient way to manage and review flashcards. Each feature will be built incrementally, ensuring that students can see the immediate impact of their work while reinforcing how the MVC architecture connects the model, view, and controller components. This approach makes the learning process more engaging and helps students understand the complete flow of the application.
-
Challenge
Setting Up the Database
In this section, we’ll focus on creating the foundational tables for the Spaced Repetition System. These tables will store data about users, flashcards, and user progress. We will break down each task into separate SQL files so that they can be executed individually using the MySQL viewer.
We will:
- Create the
cards
table to store flashcard data. - Create the
users
table to store user information. - Create the
progress
table to track each user's progress with flashcards. - Create the
user_cards
table which will track individual users data across cards.
For each task we will have a corresponding SQL file that you will create and execute using the SQL viewer.
The benefit of this approach is that the files can be ran against any future database if you had to migrate.
- Create the
-
Challenge
Establish Database Connection
In this step, we will establish a connection to the MySQL database using PHP's PDO (PHP Data Objects) class. PDO provides a consistent interface for interacting with databases in PHP and offers features such as prepared statements to prevent SQL injection attacks. To ensure that the connection is reused throughout the application, we will encapsulate it within a
Database
class. This class will implement the Singleton pattern, ensuring that only one instance of the database connection is created, reducing resource usage and potential errors. - Singleton Pattern: TheDatabase
class uses the Singleton pattern to ensure only one connection is made.- PDO Connection: A PDO connection to the MySQL database is established using the
mysql:host
,port
, anddbname
attributes. Errors during connection will throw an exception that is caught and handled. - Private Constructor: The constructor is private to prevent direct instantiation of the class from outside, ensuring the Singleton pattern.
- getInstance(): This method returns the single instance of the class, creating it if it doesn't exist yet.
- getConnection(): This method provides access to the PDO connection object.
- PDO Connection: A PDO connection to the MySQL database is established using the
-
Challenge
Adding a New Flashcard
In this section, we will build the ability for users to add new flashcards to the system. This will introduce you to the basics of the Model-View-Controller (MVC) architecture, where:
- The Model interacts with the database to perform operations.
- The Controller processes user input, communicates with the model, and updates the view.
- The View presents the form to the user and sends their input back to the controller for processing.
We will follow these steps to build the feature that allows users to add new flashcards.
In the next slice, we'll focus on listing all flashcards so users can see the cards they've added.
We'll build the Model method to retrieve all cards, update the Controller to handle listing, and create a View to display the cards.
-
Challenge
Listing All Cards
In this section, we’ll build on the Model-View-Controller (MVC) architecture you set up earlier by enabling users to view a list of all flashcards they’ve created. This slice demonstrates how the controller retrieves data from the model and passes it to the view for display.
The key learning objectives for this slice are:
- Model: Fetch data (the list of flashcards) from the database.
- Controller: Request the list of flashcards from the model and pass the data to the view.
- View: Display the list of flashcards to the user in a readable format (e.g., an HTML table).
-
Challenge
User Authentication
In this section, we will implement the User Authentication system, enabling users to register, log in, and manage their own flashcards. User authentication is essential for supporting multiple users, as each user needs to access their own flashcards, track their progress, and review their cards independently.
The user authentication system will involve two key features:
- Registration: Allowing new users to create an account with a username and password.
- Login: Enabling users to log in with their credentials and access their personalized set of flashcards.
For security, we will use PHP’s password_hash() function to securely store user passwords and password_verify() to validate credentials during login.
-
Challenge
Associating Cards with a User
In this step, we’ll focus on allowing users to associate flashcards with their accounts. This will enable each user to have their own set of cards, separate from other users. This functionality is essential for ensuring that users only review and manage their own flashcards.
The key learning objectives for this slice are:
- Model: Create the logic to associate a card with a specific user.
- Controller: Enable users to add cards to their personal list.
- View: Add the necessary UI elements that allow users to associate flashcards with their accounts.
-
Challenge
Reviewing Flashcards
In this step, we’ll implement the core functionality that allows users to review the flashcards they’ve added to their list. This is the essential part of a spaced repetition system where users answer questions, and based on their performance, the review intervals are adjusted accordingly.
The key learning objectives for this slice are:
- Model: Calculate the next review interval based on the user's performance and update the review schedule.
- Controller: Retrieve the next flashcard for the user to review and handle their review response (correct/incorrect).
- View: Display the flashcard for review and collect the user's response. We are going to user the SuperMemo 2 algorithm to help us score each answer and determine when to schedule the next review.
The SuperMemo 2 algorithm is a spaced repetition algorithm that calculates optimal intervals between reviews based on how well you remember each item. Here's how it works:
-
Each item has three main components:
- Interval: Days until next review
- Easiness: A factor that determines how quickly intervals increase
- Repetitions: Number of successful reviews
-
When reviewing an item, you grade your recall quality from 0-5:
- 0: Complete blackout
- 1: Wrong answer, but you remembered once shown
- 2: Wrong answer, but it felt familiar
- 3: Correct but difficult to remember
- 4: Correct with some hesitation
- 5: Perfect recall
-
The algorithm then:
- Adjusts the easiness factor based on performance
- Resets progress if quality < 3
- Calculates the next interval using the formula: interval * easiness
For this implementation we are going to limit the quality to 2 (incorrect) and 5 (correct) but you could enhance this going forward.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.