- Lab
- Core Tech
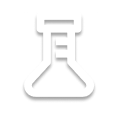
Guided: Build a Personal Finance Tracker Using React and TypeScript
In this hands-on lab, you will navigate through a carefully crafted sequence of instructions designed for aspiring front-end developers looking to gain practical experience. Your objective is to transform a basic React application into a fully-fledged personal finance management tool. Each phase of the project concentrates on refining your abilities in specific areas while utilizing React and TypeScript. By the conclusion of the lab, you'll showcase your proficiency in crafting dynamic user interfaces, resulting in a polished Personal Finance Tracker ready for real-world use.
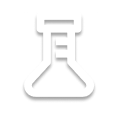
Path Info
Table of Contents
-
Challenge
Handling Routing
In this lab we are going to create a simple personal finance tracker using ReactJS and TypeScript. At the minimum, we will need two different pages - one for creating a new transaction, listing transactions and another to visualize the expenses. We will also need a way to navigate between these two pages. We will use React Router for this purpose.
Please remember that the app can be run at anytime by clicking the Run button or by running the
npm run dev -- --host
command in the Terminal. You can view the application's webpage in the Web Browser tab by refreshing the page.To make the process easier, the necessary dependencies have already been added to the project. So, in order to enable routing, we just need to add the necessary code to the App.tsx file. With your app running, you can visit the app at anytime by visiting {{localhost:5173}}
-
Challenge
Form Input & Validation
Now your app is running and you will see two links on the header, called
Transactions
andSummary
.Click on Transactions and you wil see Transactions page. Since there are no transactions added yet, you will see the button called
+ New Transaction
. Click on it and you will see the form to add a new transaction.At this time, if you click on Submit button, you will see that it adds a new transaction with empty values. You can confirm this by the table on the Transactions page. The
Name
is empty and theAmount
is 0. This is not what we want. We only want to enable the Submit button when the form is valid. -
Challenge
Styling Forms
As you may have noticed, the form we created in the previous step is not very pretty. Let's fix that.
We are going to leverage Tailwind CSS to style our form. Tailwind CSS is a framework for quickly constructing user interfaces. It is a very popular framework and is used by many companies, including Amazon, Microsoft, and Atlassian.
The nice thing about Tailwind CSS is that it is very easy to use. You don't need to know CSS to use it. You just need to know how to add classes to your HTML elements.
This project already has Tailwind CSS installed. Let's use it to style our form.
First, we need to make title
Transactions
look like a heading. Currently, it looks like a regular text. Now, as you click on the+ New Transaction
button, you will notice that theName
andAmount
labels are not looking bold, while theCategory
label is. Let's fix that. Now, let's repeat the same for theAmount
label. Great! This all looks good. Finally, if you look at theCancel
button, it looks more like a link than a button. Let's fix that. This will add a rounded border to the button and change its background color to white. It will also change the background color to gray when you hover over it.Fantastic. So as you can see, Tailwind CSS is very easy to use and using a few utility classes, you can create a beautiful UI for any app. If you are interested in learning more about Tailwind CSS, you can check out their documentation.
-
Challenge
Enable Currency Conversion
It is common these days to pay for services in different currencies. For example, if you are in the US and you are paying for a service that is based in Canada, you will pay in CAD. If you are in US and pay for services in New Zealand, you will pay in NZD. At any given time an amount in one currency is equivalent to an amount in another currency. This is called the exchange rate. Exchange rates change all the time.
We are going to be updating our project to use a function that exchanges rate between two currencies. Now, when you click on the
+ New Transaction
button, you will see a new radio button calledCurrency
. This will allow you to choose a currency for each transaction. The way this works is known as Conditional Rendering. We are rendering theCurrency
radio button only when theENABLE_CURRENCIES
constant is set totrue
. -
Challenge
State Management using Hooks
We now need to use currency conversion when adding a transaction. Currently, if you add a transaction and select a different currency, the amount does not change. This is because we are not calling the exchange rate function and using it in our calculations. You just need to use it in the right place. This rate is used to calculate
convertedAmount
which takes the amount inUSD
and multiplied by therate
to get the equivalent amount in the USD. We use thisconvertedAmount
and save in the state usingsetTransaction
. ThesetTrasaction
method is created by theuseState
hook. It takes in the new value for the state and updates it.Now that we have a conversion working, it would be great if we can also "see" the conversion rate when the currency is not USD. Let's do that next. Now, when you add a new transaction, the default currency is still USD. However, with the change we made now, whenever you change the currency, the amount will be converted into equivalent USD amount and saved in the state. We will also be able to see the conversion rate when the currency is not USD.
-
Challenge
Visualizing Transaction Data
Now that we have the ability to add transactions, including the transactions in different currencies, we should now work on visualizing the data. The most common way to see expenses is by which cateegory they belong to. In our form, there are 3 categories:
Food
,Transportation
andEntertainment
.The most simple and effective way to visualize this data is by using a bar chart. We will be using recharts for this purpose. Recharts is a charting library built on React and D3. It is very easy to use and has a lot of features.
This project is already using recharts. If you are curious, you can check out the code in
src/components/Summary.tsx
and look atShowBarChart
component. The visualization happens on a/summary
route. We added this route in the first step. We will be using the same route to show the bar chart.The
/summary
route is gets the Transaction data from theTransactionContext
and passes it to theShowBarChart
component. There are 2 situations that can happen when we visit the/summary
route:- There are no transactions
- There are transactions
We need to handle both situations Now, we need to handle the case when there are transactions. We will be using the
ShowBarChart
component for this purpose. With this, now when you visit the/summary
route, you should see a bar chart. If you don't see a bar chart, it might be likely that you have not added any transactions. Add some transactions and then visit the/summary
route.Remember to not do a page reload between changing routes. This will cause the state to reset and you will not see any transactions. This can be fixed with multiple strategies that are not in the scope of this lab.
That's it! You have successfully completed this lab.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.