- Lab
- Core Tech
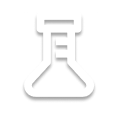
Guided: Build a Library System with Ruby
In this Guided Code Lab, you will construct an object oriented "library system" with Ruby. You will design three core classes — Book, Borrower, and Library — to simulate fundamental library operations, such as adding books, checking them out, and returning them.
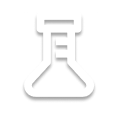
Path Info
Table of Contents
-
Challenge
Introduction
In this Guided Code Lab, you will construct an object oriented "library system" with Ruby.
You will design three core classes —
Book
,Borrower
, andLibrary
— to simulate fundamental library operations, such as adding books, checking them out, and returning them.Class Structures
Book Class
- The
Book
class will represent individual books. Each book will have attributes such astitle
,author
, andavailable
.
Borrower Class
- The
Borrower
class will represent people who come to borrow books. EachBorrower
will have attributes likename
andborrowed_books
, which will hold a list of books currently borrowed by them.
Library Class
- The
Library
class will act as the main hub where the operations take place. This class will have attributes such asbook_list
(a list of all books in the library) and methods to add books, check out books to borrowers, and receive returned books.
Interactions Between Objects
As you develop these classes, you'll also implement methods that allow these objects to interact. For example, when a borrower checks out a book, the
availability
of the book changes, and the book gets added to the borrower'sborrowed_books
list. Similarly, when a book is returned, itsavailability
changes again, and it gets removed from the borrower's list.When you are finished, click Run inside the Terminal to see the application's output.
If you're ready to get started, click the arrow below.
- The
-
Challenge
Creating a Book Class
The
Book
class creates the books inside the library.Attributes:
title
author
availability
Methods:
initialize
All of your code will be written inside the
book.rb
file. -
Challenge
Creating a Borrower Class
The
Borrower
class keeps track of who borrowed what books.Attributes:
name
borrowed_books
Let's get started!
All of your code will be written inside the
borrower.rb
file. -
Challenge
Creating a Library Class
The
Library
class will let you keep track of the inventory and peform all of the typical tasks of a library such as add books, checkout books, return books, and see what books are available.Attributes:
inventory
Methods:
initialize
add_book
checkout_book
return_book
list_available_books
Let's get started!
All of your code will be written inside the
library.rb
file. -
Challenge
Library Methods
Overview
This section will cover the helper methods inside the
Library
class that give the app it's main functionality.Methods:
add_book
checkout_book
return_book
list_available_books
Let's get started!
All of your code will be written inside the
library.rb
file. -
Challenge
Using the Library System
If you're classes are complete, you can click Run in the Terminal to see the following output:
The Great Gatsby has been checked out to John Doe. The Great Gatsby is not available. Available books: 1984 The Great Gatsby has been returned by John Doe. Available books: The Great Gatsby 1984
Feel free to experiment, add books, check them out, and use the methods from the
Library
class.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.