- Lab
- Core Tech
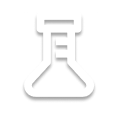
Guided: Build a Library System with Python
In this Guided Code Lab, you will construct an object-oriented "library system" with Python. You will design three core classes—`Book`, `Borrower`, and `Library`—to simulate fundamental library operations, such as adding books, checking out, and returning items. When you are finished you will have a solid grasp of Python's class structures, methods, and object interactions.
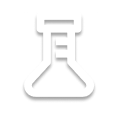
Path Info
Table of Contents
-
Challenge
Introduction
Lab Overview
In this hands-on project, you will construct an object-oriented "library system" with Python.
You will design three core classes—
Book
,Borrower
, andLibrary
—to simulate fundamental library operations, such as adding books, checking out, and returning items.When you are finished you will have a solid grasp of Python's class structures, methods, and object interactions.
When you are done click
Run
to see the application's output.Book Class
- The
Book
class will represent individual books. Each book will have attributes such astitle
,author
andavailable
.
Borrower Class
- The
Borrower
class will represent people who come to borrow books. EachBorrower
will have attributes likename
andborrowed_books
, which will hold a list of books currently borrowed by them.
Library Class
- The
Library
class will act as the main hub where the operations take place. This class will have attributes such asbook_list
(a list of all books in the library) and methods to add books, check out books to borrowers, and receive returned books.
Interactions Between Objects
- As you develop these classes, you'll also implement methods that allow these objects to interact. For example, when a borrower checks out a book, the availability of the book changes, and the book gets added to the borrower's
borrowed_books
list. Similarly, when a book is returned, its availability changes again, and it gets removed from the borrower's list.
If you're ready to get started, proceed to the next steps.
- The
-
Challenge
Creating a Book Class
Book Class Overview
The
__init__
method:This is the initializer (or constructor) method in Python, automatically invoked when you create a new instance of the
Book
class.Parameters:
-
self
: A reference to the instance of the classBook
being created. In Python, it's explicitly declared, whereas in many other object-oriented programming languages, it's a hidden parameter passed to methods and defined by the system. -
title
: An external parameter provided when you create a new instance of theBook
class. It represents the title of the book. -
author
: Another external parameter you'd provide when instantiating theBook
class. It represents the author of the book.
Attributes:
Within the
__init__
method, three instance attributes are initialized:-
self.title = title
: Sets thetitle
attribute of theBook
object to the value passed during its instantiation. -
self.author = author
: Sets theauthor
attribute of theBook
object to the value passed during instantiation. -
self.available = True
: Initializes theavailable
attribute of theBook
object toTrue
, indicating that the book is available by default when it's created.
-
-
Challenge
Creating a Borrower Class
Borrower Class Overview
The
__init__
method: This is a special method in Python that is automatically called when you create a new instance of a class (i.e., when you instantiate the class).Parameters:
-
self
: This is a reference to the instance of the classBorrower
being created. In most object-oriented programming languages, this is passed to the methods as a hidden parameter that is defined by the system. But in Python, it must be explicitly declared. -
name
: This is an external parameter that you would provide when you create a new instance of theBorrower
class. It represents the name of the borrower.
Attributes:
Within the
__init__
method, two instance attributes are being set:self.name = name
: This sets thename
attribute of theBorrower
object to the value passed when the object is instantiated.self.borrowed_books = []
: This initializes an empty list for theborrowed_books
attribute. This list is meant to store books that the borrower has currently borrowed. -
-
Challenge
Creating a Library Class
Library Class Overview
The
__init__
method:This is the initializer (or constructor) method in Python, automatically invoked when you create a new instance of the
Library
class.Attributes:
self.inventory = []
: Initializes theinventory
attribute of theLibrary
object as an empty list. This list will hold all the books in the library.
Methods:
add_book(self, book)
checkout_book(self, book, borrower)
return_book(self, book, borrower)
list_available_books(self)
-
Challenge
Library Class Methods
Adding and Checking Out Books
add_book(self, book)
:-
Purpose: Adds a book to the library's inventory.
-
Parameter:
book
: The book object that you want to add to the library's inventory.
-
Action: Uses the
append
method to add the provided book to theinventory
list.
checkout_book(self, book, borrower)
:-
Purpose: Allows a borrower to check out a book from the library, provided the book is available.
-
Parameters:
book
: The book object that the borrower wants to check out.borrower
: The borrower object representing the person checking out the book.
-
Action: If the book is available, it changes the book's
available
attribute toFalse
, adds the book to the borrower'sborrowed_books
list, and displays a message if the book is not available.
-
-
Challenge
Library Methods Continued
Returning and Listing All Books
return_book(self, book, borrower)
:-
Purpose: Allows a borrower to return a book they've checked out from the library.
-
Parameters:
book
: The book object being returned.borrower
: The borrower object representing the person returning the book.
-
Action: If the book is in the borrower's
borrowed_books
list, it changes the book'savailable
attribute toTrue
and removes the book from the borrower's list. If the borrower didn't borrow that particular book, a message is displayed.
list_available_books(self)
:-
Purpose: Lists the titles of all available books in the library.
-
Action: Uses list comprehension to return a list of titles of all books in the
inventory
that are currently available.
-
-
Challenge
Using the Library System
Using The Library
If you're classes are complete ,you should be able to click
Run
in your terminal and see the following output.Initial books in the library: - The Great Gatsby - 1984 - Brave New World - Pride and Prejudice John tries to borrow 'The Great Gatsby'... Jane tries to borrow 'The Great Gatsby'... The Great Gatsby is not available. Books John has borrowed: - The Great Gatsby Available books in the library: ['1984', 'Brave New World', 'Pride and Prejudice'] Jane tries to return 'The Great Gatsby'... Jane Smith didn't borrow The Great Gatsby. John returns 'The Great Gatsby'... Jane tries again to borrow 'The Great Gatsby'... Books Jane has borrowed: - The Great Gatsby Current available books in the library: ['1984', 'Brave New World', 'Pride and Prejudice']
Feel free to experiment, add books, check them out and use the methods from the
Library
class.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.