- Lab
- Core Tech
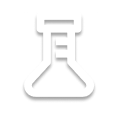
Guided: Build a Fitness Tracking App in React
Tailored for aspiring front-end developers eager to gain hands-on expertise, this practical tutorial will guide you through a carefully curated series of steps. As you progress, transform a basic React application into a comprehensive personal fitness tracker. Each phase of the project is designed to refine your skills in specific areas, leveraging React and TypeScript. By the end of the guide, you'll demonstrate your proficiency in crafting dynamic user interfaces and seamlessly integrating business logic, resulting in a polished Fitness Tracker ready for real-world use.
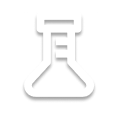
Path Info
Table of Contents
-
Challenge
Handling Routing
In this lab, we are going to create a simple personal finance tracker using ReactJS and TypeScript. We need to add a few routes—specifically, four routes. One route is already available at the home page, but we need to enable three additional routes for the following pages:
- Adding Personal Information
- Adding Activity Information
- Adding Meal Logs We will use React Router for navigation. This library is already installed in the project.
The app can be run at anytime by clicking the Run button or by running the
npm run dev -- --host
command in the Terminal. You can view the application's webpage at the following location: {{localhost:5173}}.To make the process easier, all the necessary dependencies have already been added to the project. So, we are only going to focus on the writing the code for the project. With this your application should have four menu items. You can verify this by running the application and checking the menu items. However, if you click on the menu items, you will see blank pages. This is because we have not yet added the routes for these pages. Let's do that next, but first click on the "back" button to go back to the project home page.
-
Challenge
Adding Personal Information
The first thing we need to do is to allow users to add their personal information. This includes their age, gender, height in cm, weight in kg, among other important elements that a fitness tracker needs. At the current stage, if you open the
/personal
route, you will see a form that takes all this information from the user. However, there are a few things that are not working, so let's work on them.To start with, the save button is always enabled. The user must provide all the information before they can save it. So let's disable the save button until all the information is provided. With this change, the save button will be disabled until all the information is provided. You can verify this by visting the application on
/personal
route and checking the save button. When you have provided the information, the save button will be enabled.Now, with the save button enabled, you can click on it, however nothing will happen. This is because we have not yet implemented the save functionality. Let's do that next. You can verify this by visiting the application on
/personal
route, filling out the form and clicking on save button. You will see that the information is saved and available in the form as is. Additionally, you will see a banner that displays the daily calorie requirement.There is one final thing we must do. Currently, when you click on Cancel button, the form is not cleared. Let's fix that. You can verify this by visiting the application on
/personal
route. After filling out the form and clicking on the Cancel button, you should see that the form is cleared. -
Challenge
Adding Activity Information
The basic information helps us identify how many calories a user needs to consume in a day. The activity information helps us identify how many calories a user burns in a day. This is important to calculate the net calories consumed by the user.
In this step, we will add the ability for the user to add their activity information. The available activities are Walking, Running, and Swimming. The user can add the duration of each activity in minutes. The application will calculate the total calories burned by the user in a day. With this change, we now have a working function that calculates the estimated calorie burn for a given activity. Let's use this function to calculate the total calorie burn for a day. With this change, if you visit the application on
/activity
route, and enter the information, the save button will be enabled. You can click on it and the information will be saved. However, you won't see the information on the screen. To see the information, you need to refresh the page and once you do that, you will see the information with the total calorie burn for that activity you added earlier. However, this is inconvenient, we want to see the information as soon as we save it. Let's fix that now. With this change, if you visit the application on/activity
route, and enter the information, the save button will be enabled. You can click on it and the information will be saved. You will see the information on the screen without having to refresh the page. -
Challenge
Adding Meal Information
The basic information helps us identify how many calories a user needs to consume in a day. The activity information helps us identify how many calories a user burns in a day. The meal information helps us identify how many calories a user consumes in a day. This is important to calculate the net calories consumed by the user.
In this step, we will add the ability for the user to add their meal information. This is a simple form. A user can add the name of the meal and the number of calories consumed. Since the meals are not predefined, the user can add any meal they want. Also calculating the caloris in a recipe is out of scope for this application. This is because the recipe can be complex and the user may not know the exact ingredients and their quantities. So, we will assume that the user knows the number of calories in the meal they are adding.
If you head over to the application and visit the
/meal
route, you will see a form to add the meal information. However, the save button is disabled. This form is working. If you enter the information and click on the save button, the information will be saved in the database. You also see the information on the screen as soon as you save it. However, when you refresh the page, the information is gone. This is because we are not loading the information from the database when a page is refreshed or when a user visits the route from a different route. Let's fix that. With this change, the meal logs will be loaded from the database when the page is refreshed or when a user visits the route from a different route. -
Challenge
Enable Tracker Dashboard
This is the final step in this lab. So far, we have done the following:
- We enabled user to enter their basic information.
- We enabled the user enter their activity information.
- We enabled the user to enter their meal information.
Now, we must add the ability for a user to see all the information in one place. A single dashboard that shows all their activity and meals information. To make it useful, we must also calculate the net calories consumed by the user. Finally, we must show them the balance for the day. This is the number of calories they need to consume to maintain their weight. This is calculated by subtracting the calories burned from the calories consumed and finally subtracting the output with their daily calorie requirement.
This will be available on the application home page. So, when a user visits the application, they will see the dashboard. The project has all the code to display the tabular information, but we need to write application logic for the information to be displayed. With your change, we can now merge the activity logs and meal logs into a single array of
TableRow
. This will allow us to display the information in a tabular format. Now, the final step. If you visit the application, you will see the dashboard. However, the "Net Calories Consumed" is0
. This is because we have not calculated the net calories consumed by the user. Let's fix that. Fantastic! You have completed the lab. You can now visit the application and see the dashboard, add activity and meal information, and see the dashboard update in real-time.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.