- Lab
- Core Tech
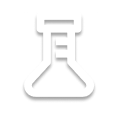
Guided: Build a Drum Machine with Vue
In this guided lab, learners will use the Vue.js framework to construct an interactive drum machine. This application will enable users to create unique rhythms by setting different drum elements such as the kick, snare, and hihat. Each drum track is visually represented, and users can easily toggle beats between active ('x') and inactive ('-') states. The application provides controls to adjust the BPM (beats per minute) and play or stop the drum sequences. To produce the actual drum sounds, we'll be utilizing HTML audio samples.
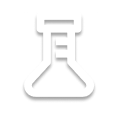
Path Info
Table of Contents
-
Challenge
Overview
In this guided lab, you will use the Vue.js framework to construct an interactive drum machine. This application will enable users to create unique rhythms by setting different drum elements such as the kick, snare, and hihat. Each drum track is visually represented, and users can easily toggle beats between active (
'x'
) and inactive ('-'
) states. The application provides controls to adjust the BPM (beats per minute) and play or stop the drum sequences. To produce the actual drum sounds, we'll be utilizing HTML audio samples.Using the Drum Machine
-
Click the Run button in the bottom right of the Terminal and then visit the Web Browser tab. This provides a real-time preview of your drum machine as you work on it.
-
Adjust BPM: Locate the BPM input field and try setting different values.
-
Toggling Beats: On the visual representation of the tracks, click on any beat to toggle its state.
-
Control Playback: Use the Start and Stop buttons to initiate or halt the drum loop playback.
Tips:
- To ensure the changes in rhythm patterns are audibly reflected, stop the playback before modifying a beat pattern and then restart it.
Happy coding!
-
-
Challenge
Creating the Vue Template
Task: Generate Drum Track Divs.
Iterate over the
trackList
and create a div for each track.👣 Steps
- Use the
v-for
directive to loop overtrackList
. - Bind each track's name to the
key
attribute to ensure uniqueness. - Assign the class
track
to each generated div.
🔍 Hint
In Vue, the
v-for
directive allows you to iterate over items in an array and render a block of code for each item.🔑 Solution
<div v-for="track in trackList" :key="track.name" class="track"> </div>
Task: Display the Track Label.
Display the capitalized name of the drum track followed by a colon.
👣 Steps
- Inside the div from the previous task, use the
{{ }}
syntax to bind and display the track's name. - Use JavaScript string methods to capitalize the first letter of the name.
- Follow the name with a colon.
🔍 Hint
You can capitalize the first character of a string and add the rest of the string using string manipulation methods like
charAt()
andslice()
.🔑 Solution
<span>{{ track.name.charAt(0).toUpperCase() + track.name.slice(1) }}:</span>
Task: Generate Beat Divs for Each Drum Track.
For every drum track, iterate over its pattern and create a div for each beat.
👣 Steps
- Inside the main track div, use another
v-for
to loop overtrack.pattern
. - Bind the index of each beat to the
key
attribute. - Conditionally assign the
active
class if the beat equals 'x' using the object-syntax for class bindings.
🔍 Hint
Vue allows nested loops using
v-for
. For conditional class bindings, Vue's class binding syntax{ 'class-name': condition }
can be useful.🔑 Solution
<div v-for="(beat, index) in track.pattern" :key="index" :class="['step', { 'active': beat === 'x' }]" @click="toggleStep(track.name, index)"> </div>
Task: Add BPM Input.
Provide an input field for users to set the BPM (Beats Per Minute).
👣 Steps
- Use the
v-model
directive to bind the BPM value. - Specify the input type as
number
. - Assign an ID to the input for possible further referencing.
🔍 Hint
v-model
in Vue provides two-way data binding on aninput
form control.🔑 Solution
<input v-model="bpm" type="number" id="bpmInput" />
Task: Add Control Buttons.
Add buttons to start and stop the drum machine.
👣 Steps
- Create two button elements.
- Assign click events using the
@click
directive to callstartDrumMachine
andstopDrumMachine
methods respectively.
🔍 Hint
In Vue, the
@click
directive is a shorthand forv-on:click
, which is used to listen to DOM events and execute some JavaScript when they're triggered.🔑 Solution
<button @click="startDrumMachine">Start</button> <button @click="stopDrumMachine">Stop</button>
- Use the
-
Challenge
Create the Drum Machine Functionality
Task: Initialize Component Data.
Set up the initial data properties for the Vue component.
👣 Steps
- Declare a
data
function that returns an object. - Initialize
drumInterval
tonull
. This will store the interval reference for the drum machine. - Set up a
trackList
array that contains objects with information on each drum track. - Initialize
bpm
(Beats Per Minute) to 120.
🔍 Hint
In a Vue component, the
data
function returns an object that holds the initial values for the properties.🔑 Solution
data() { return { drumInterval: null, trackList: [ { name: 'kick', pattern: 'x-------x-------', sample: new Audio('/kick.wav') }, { name: 'snare', pattern: '----x-------x---', sample: new Audio('/snare.wav') }, { name: 'hihat', pattern: 'x-x-x-x-x-x-x-x-', sample: new Audio('/hihat.wav') } ], bpm: 120 }; }
Task: Implement the
toggleStep
Method.Create a method to toggle a step (from '-' to 'x' or vice versa) in the pattern of a specific drum track.
👣 Steps
- Declare a
toggleStep
method that acceptstrackName
andindex
as parameters. - Find the specified track in
trackList
. - Modify the track's pattern at the specified index.
🔍 Hint
Use the
find
method on arrays to locate the desired track based on its name.🔑 Solution
toggleStep(trackName, index) { let track = this.trackList.find(t => t.name === trackName); let pattern = track.pattern; let active = pattern[index] === 'x' ? '-' : 'x'; track.pattern = `${pattern.slice(0, index)}${active}${pattern.slice(index + 1)}`; }
Task: Implement the
startDrumMachine
Method.Create a method to start playing the drum machine.
👣 Steps
- First, stop any running drum machine using the
stopDrumMachine
method. - Calculate the time between each drum beat using the
bpm
. - Use
setInterval
to loop through each drum track and play the associated sample if its pattern has an 'x' at the current step. - Update the step counter and loop it back to the start when it reaches the end.
🔍 Hint
Remember to use
this
to refer to the component's data and methods within the methods.🔑 Solution
startDrumMachine() { this.stopDrumMachine(); const step_time = 60000 / this.bpm; let step = 0; this.drumInterval = setInterval(() => { this.trackList.forEach(track => { if (track.pattern[step] === 'x') { track.sample.currentTime = 0; track.sample.play(); } }); step = (step + 1) % this.trackList[0].pattern.length; }, step_time); }
Task: Implement the
stopDrumMachine
Method.Create a method to stop the drum machine.
👣 Steps
- Check if
drumInterval
is not null. - Use
clearInterval
to stop the currently running drum machine. - Reset
drumInterval
to null.
🔍 Hint
clearInterval
is a built-in JavaScript function that stops an interval started bysetInterval
.🔑 Solution
stopDrumMachine() { if (this.drumInterval) { clearInterval(this.drumInterval); this.drumInterval = null; } }
- Declare a
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.