- Lab
- Core Tech
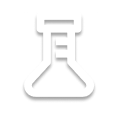
Guided: Build a CSV File Viewer in Svelte
This Guided Code Lab will help you get up to speed with several of the core concepts of the popular JavaScript framework - Svelte. You will be guided along a series of steps as you build a real application that will help you learn about Svelte components, reactive primitives, and directives. By the end of the lab, you will have a fully functioning Svelte application that will allow you to upload and visualize a CSV file.
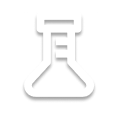
Path Info
Table of Contents
-
Challenge
### Introduction
Handling file uploads and viewing the data within those files is an integral part of many applications. In this Guided Code Lab you will be building a CSV File Viewer in Svelte. You will receive a partially complete application that you will finish up as you follow each step in this code lab. By the end of this lab, you will have built yourself a Svelte application that can visualize CSV files within a data table.
Svelte is gaining popularity as a JavaScript framework primarily because of its simplicity and fast, compile-time optimizations. In this lab, you will get a taste of the simplicity of Svelte as you focus on learning about events, passing data between components, and visualizing data within HTML tables.
info> In the Terminal, run
npm run dev -- --host
to start a development server. The application will be available, with hot module replacement, at {{localhost:5173}}. Open this URL in a different tab so you can observe your changes in real time.
Completed Svelte components have been added to the
solutions
directory. You can view these at any time if you get stuck! -
Challenge
### Reactive Statements and Dispatching Events
At its core, the Svelte framework is just simple HTML, CSS. and JavaScript. Svelte components are comprised of a single file that contains places for each of the above technologies.
Each component contains:
- A
script
block for JavaScript code - A
style
block for CSS styles - A place to put arbitrary HTML markup
In this step, you will be working on implementing the existing
FileInput
component located atsrc/components/FileInput.svelte
. This component will be responsible for:- Providing the file upload input that will upload a CSV file
- Using the
papaparse
third-party library to parse incoming CSV files by way of a Svelte reactive statement - Dispatching an event that sends the data upstream to a parent component Now you need to connect this input HTML element to the
files
component property that exists within yourFileInput.svelte
component script. To do this, use abind
directive.
The
bind
directive let's you connect local component properties to data from child components or arbitrary HTML elements. Thebind
directive will keep your component properties in sync! Svelte includes reactive capabilities by default within the framework. Reactive statements make it extremely easy to execute some code any time one of your component properties changes!In Svelte
$:
marks a statement as reactive. TheFileInput
component contains a reactiveif
statement that you will use to parse an uploaded CSV file. Great! Now that you have your actual CSV file data, you need to inform your parent component that a successful upload happened by using an event dispatcher.Event dispatchers do just what the name implies - they dispatch events! Event dispatchers allow your components to create and send out custom events. You can name these events anything you'd like and they can contain a generic payload as well. Great job! After all of the above tasks are complete, you should now have a
FileInput
component that renders a file upload input on to the page that will let you upload a CSV file into the application.info> There is a CSV file containing some Wall Street financial market data available to test with within the
static
folder when it comes time to upload CSV files to your application! To download this file, you can simply click on the ellipses to the right of the file and select Download.But you aren't doing anything with the uploaded CSV file yet... let's move on to the next step!
- A
-
Challenge
### Component Event Binding and Props
It is often necessary to pass data between Svelte components. You can pass data from parent components to child components by using component properties or "props". You can also pass data to parent components from child components via event binding.
In this step, you will:
- Bind an event handler to the
FileInput
component'supload
event. - Import a child component into a parent component and pass data to it via props.
- Learn how to connect a CSS class in a component's style block to HTML markup. In the last step you set up an event dispatcher such that your
FileInput
component will fire off anupload
event containing the parsed CSV file data. You now must bind to this event in the top level page component. Well done! You should now be able to navigate to the web browser containing the running application ({{localhost:5173}}) and see a basic data table showing up once a CSV file is uploaded. But where are the rows that are supposed to populate within the table? Let's get to that in the next step!
- Bind an event handler to the
-
Challenge
### Populating Tables with Data
Tables are perfect for visualizing CSV files in the browser. In this step, you will learn how to use Svelte to create and populate HTML tables.
In this step, you will:
- Style the
table
housed within theDataTable
component. - Learn how to use
#each
logic blocks to iterate over data and create HTML markup. - Bind component event handlers to HTML click events.
- Learn how to use
#if
logic blocks to render different text and HTML onto the page based on the value of a component variable. Every major JavaScript framework has the ability to generate HTML elements for a given dataset. Svelte is no different! It provides this ability via the#each
logic block. Equally as important as the#each
logic block, the#if
logic block allows you to conditionally render a chunk of HTML. Usually you will want to check the value of a component prop or internal variable and render some HTML elements or text differently depending upon whether the value of this variable istrue
orfalse
. Well done! You should now be able to view the full working version of this application at {{localhost:5173}}.
Try to upload a CSV file and visualize the data in your table! Remember, there is a CSV file containing some Wall Street financial market data available to test with within the
static
folder. - Style the
-
Challenge
### Conclusion
Great job!
You now have a CSV File Viewer application built via Svelte. To continue your learning, view the other Svelte Code Labs and Video Courses that Pluralsight has to offer!
If you want to keep exploring and take this app even further, here are some questions to consider:
-
Can you add a button next to the file upload input that will allow you to clear the data currently within the table?
-
Can you add a button to each header element in the table that will allow for erasing a given column?
-
How might you use a store to hold the parsed CSV data and consume this data directly within the DataTable component, no longer needing to pass the data to this component as a prop?
-
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.