- Lab
- Core Tech
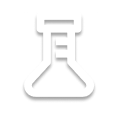
Guided: Build a CLI Password Generator with Go
In this lab, you’ll build a personalized password generator using the Go programming language and the command-line interface (CLI). You’ll start from scratch and complete various tasks to create a fully operational application that can create customized, hard-to-guess passwords. Your tool will accommodate differing user needs, such as passwords of specific length or without certain types of characters. This lab teaches you to build an application that adapts to specific requirements, providing a flexible solution for password generation.
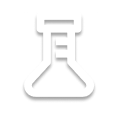
Path Info
Table of Contents
-
Challenge
Introduction
In this lab, you will create a command-line interface (CLI) password generator using Go. You'll use Go's standard library and CLI interaction to make strong and random passwords based on what the user wants.
There are three files you'll work with:
main.go
,password.go
, andrandom.go
. To finish this lab, you need to understand essential Go language concepts like Go syntax and structure, CLI interaction, randomness in Go, and handling errors.Before we proceed, it's important to note that you will initially not be able to run the lab as it is. As you progress through and complete various tasks, different components of the lab will start working. Once you've successfully implemented all parts, you'll be able to run the app at any time by clicking the Run button or entering
go run .
in the Terminal.Additionally, you will be able to specify certain criteria for password generation using flags in the command prompt:
-
To generate a password with a specific length (e.g., 12 characters), use:
go run . -l 12
-
To exclude uppercase letters from the password, use:
go run . -nu
-
To exclude lowercase letters from the password, use:
go run . -nl
-
To exclude numbers from the password, use:
go run . -nn
-
To exclude special characters from the password, use:
go run . -ns
You can combine these flags based on their preferences. For example, to generate a 10-character password excluding lowercase letters and special characters, you can use:
go run . -l 10 -nl -ns
These options provide flexibility for you to create passwords tailored to their specific requirements.
-
-
Challenge
Importing Modules and Defining Command Line Flags
First, you will need to import the necessary packages for your
main
program.The
fmt
package, used for formatting input and output is already added in the program. You will need to add theflag
package, which is essential for handling command-line arguments. This will allow you to define and parse command-line flags and is crucial for user input. Next, you will define different command-line flags that will allow users to customize their password generation process. By using theflag
package, you will be able to define an integerflag
for the passwordlength
andboolean
flags for excluding different character types from the password. -
Challenge
Generating Password with Different Character Sets
In this step, we will delve into the
Generate
function inpassword.go
. It accepts parameters likelength
,includeUpper
,includeLower
,includeNumbers
, andincludeSpecial
to customize the generated password. The function constructs a charset string based on the included character types. It then builds the password string, ensuring each selected type is represented. -
Challenge
Building Randomness in Password Logic
In this step, we look at the
random.go
file. It has two main functions:StringWithCharset
andShuffle
.StringWithCharset
makes a random string. It uses the length we want and the characters we give it.Shuffle
changes the order of the characters in a string to make it random. These two functions help us create random strings. After finishing all tasks successfully, you can now execute your Go program to generate random passwords. This tool is valuable as it boosts security by producing robust and unpredictable passwords.You can run the app at any time by clicking the Run button or entering
go run .
in the Terminal.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.