- Lab
- Core Tech
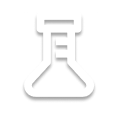
Guided: Build a CLI Game with Go
In this lab, you'll construct an immersive text-based adventure game using the Go programming language. Starting from an initial understanding of the game's structure, you'll progressively build a comprehensive, interactive gaming environment. You'll introduce new rooms, items, and engaging puzzles to entertain and challenge your player. This lab is a practical guide to building an interactive application that adapts to varying player commands and choices, providing a flexible, engaging solution for text-based gaming. You'll walk away with a deep understanding of structuring and implementing a text-based adventure game in Go, and the skills to expand the game with more rooms, items, and puzzles.
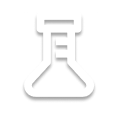
Path Info
Table of Contents
-
Challenge
Introduction
In this lab, you will build a text-based adventure game called The Dungeon of Mysteries using Go. You'll use Go's standard library and text interaction to make an interactive game where the player navigates rooms and solves puzzles.
Much of the core logic for the game has already been supplied within the
main.go
,room.go
,player.go
andutils.go
files. The main files you will be working with to complete the game are thegame.go
andpuzzles.go
files. All files can be found within thesrc/main
folder in your file tree. If at any time you get stuck, you can find completed versions of each file within thesolutions
folder in your file tree.To complete this lab, you'll need to understand Go concepts like
structs
,interfaces
,control flows
,text interaction
, anderror handling
.Before we proceed, it's important to note that you will initially not be able to run the lab as it is. As you progress through and complete various tasks, different components of the lab will start working. Once you've successfully implemented all parts, you'll be able to run the game at any time by clicking the Run button or entering
go run .
in the Terminal.The player will be able to navigate through dungeon rooms using commands like
go north
andgo east.
Rooms can contain puzzles that must be solved before the player can advance. The player can also find and use items by typing commands likeget key
anduse key
.The core gameplay loop will read player text input, parse the commands, update the game state, and print output. You'll build this loop in
game.go
and expand it to add items, puzzles, and more as you complete the lab.The
Room
,Player
, andPuzzle
structs defined inroom.go
,player.go
andpuzzles.go
will be key components. Handling errors and text interaction will also be important to make a robust, playable game.Let us start building The Dungeon of Mysteries.
-
Challenge
Modifying the Game State
The
game.go
file contains the Gamestruct
which managesstate
and theStart()
method which runs themain
game loop.Game
handles initializing rooms, connecting them, creating puzzles, and tracking player inventory. TheStart()
loop gets player input, parses commands, changes state, and outputs text. The player wins by solving puzzles to reach theTreasureRoom
and unlocking the door with akey
.game.go
handles core gameplay - player actions affectGame
state which then prints outputs. The file sets up and runs the interactive text adventure game. -
Challenge
Mechanics and Player Interactions
The adventure game engine orchestrates a rich array of mechanics and player interactions. When a player enters the
TreasureRoom
without a key, they're relocated to theEntrance
, demonstrating the game's end conditions.Puzzle
handling is alsokey
: a solved puzzle alters player interactions in that room. Movement commands likeGo
direct the player's path through thegame
. Additionally, the engine managesitem
interactions: when a player picks up anitem
, it's removed from the room's inventory and added to theirs, reflecting real-world object interactions. This blend of mechanics and player interactions creates an engaging gameplay environment. -
Challenge
Refining User Interaction Mechanisms
Once you complete all the steps successfully, you'll be able to run the game by clicking the Run button or entering
go run .
in the Terminal.Available commands:
Go East
Go North
Go South
Go West
Get Key
Use Key
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.