- Lab
- Core Tech
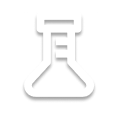
Guided: Build a CLI File Reader in C
This hands-on code lab is designed to assist you in completing a basic CLI (Command Line Interface) text file reader application in C . Following these guided steps will walk you through how to implement some of the core aspects of the application, such as open a text file for reading, loading its content into memory, searching for text, and processing command line input. Upon completion of the lab, you will have showcased your capability in constructing a fully operational basic text file reader, capable of loading a text file passed via the command line, showing its content in the terminal, searching for text, and processing command line arguments.
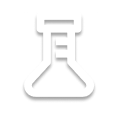
Path Info
Table of Contents
-
Challenge
Opening and Reading a File Line-by-Line in C
Welcome to this C++ guided lab!
Code lab organization
This lab is organized in steps, and each step has one or more tasks to be completed. Start with the
FileReader.cpp
source file. (If, for some reason, you need to undo your modifications, and resume the initial state of that source file, you can copy-and-paste the content ofstarter_files/FileReader1_initial.cpp
).For your convenience, if you are stuck in one task, partial solution files have been provided, so that you can use them to unlock and make progress. The solution files are provided under the
solution
directory. There are subdirectories for each step (e.g. step1, step2, etc.). Under each step subdirectory, you'll find solution files for each task of that step.When you start a new step, you may want to copy the
starter_files/FileReaderN_initial.cpp
(where N = 1, 2, 3... is the step number) file content intoFileReader.cpp
, to be sure that you and the tool are on the "same page" for the current code status at the beginning of the step.How to build C++ code
To compile the C++ source code, you can use the GNU C++ compiler from the terminal. For example, you can issue the command:
g++ -Wall -std=c++14 FileReader.cpp
This will compile the C++ source code FileReader.cpp, and produce the executable a.out. You can run the compiled code launching the executable file
a.out
previously generated:./a.out
Of course, you can pass parameters via the command line. For example, you can specify the input text file to process:
./a.out test.txt
Note: The code of this code lab is written in modern C++, and can be compiled with C++ compilers supporting C++11/14/17 and next.
Enjoy the lab! Let's start with this simple C++ skeleton code for a console application. As you can see, a
std::string
variable has already been created to represent the input file name, and an instance of thestd::ifstream
object to read the file.Now, you need to read the lines from that file. You can use the
std::getline
function for that. Now that you have successfully invokedstd::getline
to read a single line from the file, create awhile
loop to read all the lines from the input file, line by line.The expected output are all the lines read from the input file.
-
Challenge
Generate Memory Representation from Input File
You know how to read the content of the input text file line by line. Now, you need to create a memory representation for the file. You can use the
std::vector
combined withstd::string
. Basically, each line from the input file will be represented using astd::string
, and you'll store all these lines into astd::vector<std::string>
. In the code in FileReader2.cpp such a vector of strings has already been created to store the lines read from file. Now let's try to better organize your code. Instead of having the loading code directly insidemain()
, write a functionLoadFileLines()
that takes as input the file name, and returns astd::vector<std::string>
containing the file lines. Invoke that function frommain()
to print out the file lines. -
Challenge
Searching for Text in an Input Text File
Now that you have the input text file loaded into memory using a
std::vector<std::string>
, you'll implement a search feature. In particular, you'll write C++ code to search for an input word in the memory representation of the input text file.In FileReader3.cpp, you'll see the prototype of a function named
SearchText
that takes as input the string vector that stores the lines of the text file, and a search string. This function will returntrue
if the input string was found in the text file,false
otherwise. -
Challenge
Processing the Command Line
So far both the file name and the search text have been hard-coded inside the C++ source code. In this step, you'll read those from user input, as parameters passed via the command line. The syntax for your program will be the following:
a.out <text file name> [<search text>]
The first parameter represents the input text file name.
The second parameter is optional, and represents the text to search for. If not present, no search is done.
Moreover, if nothing is specified from the command line, an help screen will be printed out. Continuing with the processing of the command line, if one parameter was passed, interpret it as the input text file. Add an
if
block to print out the file content if one parameter is passed to the command line (in this case, remember thatargc
is 2: the executable, and the first parameter). Now add another branch to theif
statement, like anelse if
, to check if two parameters are passed on the command line. In this case, intepret the first parameter as the file name, and the second parameter as the text to search. Invoke theSearchText
function already written and available in the source code, to print out the lines with the found text. If that function returns false, it means that no text was found. In this case, print out the message"Text not found in the input file."
-
Challenge
Handling Exceptions
In this step, you'll see how to handle exceptions to process error conditons. Now, to make things nicer, you can catch the exceptions inside
main
, and print an error message, instead of abruptly aborting the program with a core dump (as happens in the current state of the program). Congratulations on completing your text file reader app written in C++! :-)
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.