- Lab
- Core Tech
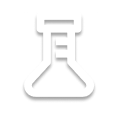
Guided: Build a Blog Platform in Next.js
Embark on a journey to create your own blog platform using Next.js with this Guided Code Lab. Whether you're a seasoned developer or just starting out, this Lab covers essential steps to set up and customize a powerful blog engine. By the end, you'll have a functional, visually appealing blog platform, empowering you to confidently create, customize, and maintain your blog.
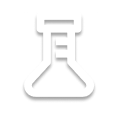
Path Info
Table of Contents
-
Challenge
Introduction
In this Guided Code Lab, you will create a blog platform using Typescript and Next.js. The blog will be written using the files in the Filetree.
The lab's environment has already been set up with all of the necessary dependencies and styles to get your blog up and running. To start your project, open the Terminal and run the following command:
npm run dev
This command will start the project in the development mode. You can then navigate to your blog platform homepage, where the project will now be running.
info> For the duration of this lab, please make sure that the project is running in the Terminal. As you make changes, the project will automatically reload and show the changes in your blog platform.
When you start the project, the blog page will be empty because there are no posts yet. Your first task is to provide a message to inform users about this. This is known as a conditional rendering. In React, you can conditionally render a component. In this case, you are checking if the
allPostsData
array is empty. If it is empty, you are rendering theNoBlogPosts
component. This component will display a message that says "No blog posts found!".Once you add the code, the page will automatically reload and you will see the message since there are no blog posts added yet.
-
Challenge
Adding Blog Posts
In the Filetree, you will see an empty
posts
directory. You need to add some posts to your blog platform. These posts should be written in a markdown (.md
) file. The goal of this step is to have files listed on the home page of your blog platform.If you look inside of the
backup
directory, you will see there are two sample posts already created. You should now see two blog posts on the homepage, each containing a title and date. Since there are only two blog posts, it is easy for the learner to scan and see which one was posted more recently. As you continuing adding more and more blog posts, this will get more difficult for the viewer. As a best practice, you should make sure your blog posts are listed chronologically, with the most recent blog posted at the top. -
Challenge
Adding Details to Each Post
Now that the two blog posts are listed in the correct order, you notice that they are not clickable. Before they can be accessed, you need to create detail pages for each blog post. There are a few steps you need to take in order to achieve this. Next, you need to systematically process the markdown content. Open each of the markdown files and look at how the content is structured. At the top of each markdown file, you will see the following content:
--- title: "First Post" date: "2021-01-01" ---
This metadata is known as frontmatter. After the frontmatter, you will see the body of the content. You will need to read all of this markdown content and display it correctly in the blog post details page. Locate the
getGrayMatterContent
function in thelib/post.ts
file. Currently, this function takes the file content as a string and returns an empty string wrapped in a call to thematter()
function.The
getGrayMatterContent
function is called by thegetPostData
function in the same file, which reads the content of the markdown file. Subsequently, thegetPostData
function is invoked by thegetStaticProps
function in thepages/posts/[id].tsx
file to fetch data for individual blog post pages. Thematter()
function is utilized to read the frontmatter of the markdown file. Great! You now have unique ids for each blog post and can read the content of the markdown file. Next, you need to ensure that the markdown content can be displayed as HTML content. Now, you have implemented the following:- Unique ids for each blog post
- Reading the content of the markdown file
- Converting the markdown content to HTML content
Your individual blog pages are ready! However, you will see an error on the console similar to the following:
⨯ TypeError: getStaticPaths is not a function at buildStaticPaths (/Users/harit/code/ts/ps-next-blog/node_modules/next/dist/build/utils.js:786:39) at Object.loadStaticPaths (/Users/harit/code/ts/ps-next-blog/node_modules/next/dist/server/dev/static-paths-worker.js:62:46) at process.processTicksAndRejections (node:internal/process/task_queues:95:5) { type: 'TypeError', page: '/' }
This typically happens because the cache is not cleared automatically. Restart the project in the Terminal and you should now see your blog post pages at the /posts/pre-rendering and /posts/ssg-ssr links.
However, these pages are empty. This is because you have the data, but you haven't rendered it anywhere. Therefore, the individual blog post pages do not show the title, date, and content of the blog post. The next step is to render this data. You should now see the title of the blog post on the individual blog post page. You can validate it by visiting the /posts/pre-rendering and /posts/ssg-ssr links.
Great! Next, you will add the date of the blog post. With this change, you should see the title and date of the blog post on the individual blog post page. You can validate it by visiting the /posts/pre-rendering and /posts/ssg-ssr links.
Next, you will add the content of the blog post. You should now see the title, date, and content of the blog post on the individual blog post page. You can validate it by visiting the the /posts/pre-rendering and /posts/ssg-ssr links.
Finally, you will enable the links from the homepage to the individual blog post pages. Now when you visit the homepage on the blog, you should see the links to the individual blog post pages. You can validate it by visiting the homepage link.
Moving forward, you can add a new blog post to the platform by adding a new markdown file in the
posts
directory. You will then see a new link on the homepage. -
Challenge
Adding SEO to Blog Pages
In this step, you will add SEO meta tags to the blog post details page. This will help search engines to understand the content of the page and display it in search results. The search engine optimization (SEO) meta tags are added to the
<Head>
component in the Next.js application. These help search engines to understand the content of the page and display it in search results.There are many SEO meta tags that can be added to the page. However, for this project, you will add the following tags:
<title>
tagtitle
meta tagdescription
meta tag
Once you know how to add these meta tags, you can add more meta tags to the page as needed. You can validate this by opening the /posts/pre-rendering and /posts/ssg-ssr links. With this change, the title of the blog post will appear in the tab, aiding users in identifying the page, especially when they have multiple tabs open. To validate this change, perform the following steps:
- Open the /posts/pre-rendering and /posts/ssg-ssr links.
- Right-click on the page and select View page source.
- Search for the
title
meta tag in the page source. This is usually found in the<head>
section of the page. Thehead
is the first section of the page and contains meta tags, links to stylesheets, and other resources. You should see thetitle
meta tag with the title of the blog post.
With this change, the title of the blog post in the search results. The search engine will display the title of the blog post in the search results. This will help users to identify the content of the page before they click on the link.
The difference between the
<title>
tag and thetitle
meta tag is that the<title>
tag is displayed in the browser and thetitle
meta tag is displayed in the search results.The final step is to add the
description
meta tag to the page. You can validate this task by performing the same steps as before:- Open the /posts/pre-rendering and /posts/ssg-ssr links.
- Right-click on the page and select View page source.
- Search for the
description
meta tag in the page source. This is usually found in the<head>
section of the page. Thehead
is the first section of the page and contains meta tags, links to stylesheets, and other resources. You should see thedescription
meta tag with the description of the blog post.
With this change, the description of the blog post will be available in the search results. The search engine will display the description of the blog post in the search results. This will help users to understand the content of the page before they click on the link.
Congratulations! You have completed this Guided Code Lab on creating a blog platform using Next.js. You have learned how to:
- Create a blog using markdown files.
- Add a list of blog posts to the homepage.
- Add individual blog post pages.
- Add SEO meta tags to the blog post details page.
You can now use this knowledge to create your very own blog using Next.js. Happy coding!
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.