- Lab
- Core Tech
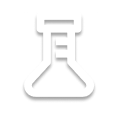
Guided: ARIA Foundations for Web Accessibility
In order to be as inclusive as possible, it's important to ensure that your web applications adhere to ARIA (Accessible Rich Internet Applications) principles and best practices. In this Guided Lab, you will take a small website with an action button and make it accessible using ARIA attributes. You will also learn how to write unit tests for accessible web applications in order to ensure that they conform to the accessibility standard.
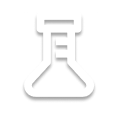
Path Info
Table of Contents
-
Challenge
### Introduction
In this lab, you are going to learn how to use the principles and techniques of ARIA (Accessible Rich Internet Applications) to make your web applications more accessible. This is important from a business perspective, since you want as many people as possible to interact with your website. But it’s just as (if not more) important from an ethical standpoint. In this lab, you’ll be learning how to use ARIA foundations by building a button that pops open an action menu which the user can select a list of action items from. This sort of feature is something that you might take for granted when it comes to most websites and it’s important to ensure that experiences like these are available to people with disabilities as well.
What exactly is ARIA? You can think of ARIA as a term that encompasses all of the accessibility functions within the HTML specification that allow users of a website to interact with it via a screen reader. Functionally, this means that ARIA is comprised of HTML roles and attributes. By assigning certain roles, states and properties to different HTML elements, you can be very explicit about the function of your website and ensure that users with disabilities can interact with your website in an accessible fashion.
Some of the things that you’ll learn about in this lab include:
- Using ARIA roles to declare the structure of your website
- Declaring ARIA states and properties that link elements together and allude to the functional elements of your web application
- Unit testing ARIA applications
By the end of this lab, you will be confident in the fundamental principles of ARIA and should be ready to start implementing ARIA from a practical standpoint in your application or organization.
info> Note: The
solution directory
contains the final solution. Feel free to view the file in that directory should you get stuck! -
Challenge
### Using Roles for Accessibility
In this step, you are going to learn about the ARIA
role
attribute and how you can use it to improve web accessibility. ARIA roles are a set of attributes that you can assign to HTML elements in order to provide more information about the overall structure of a webpage to screen readers and other assistive technologies. These roles are meant to augment the existing accessibility that comes with using HTML semantic elements likebutton
or<main>
.There are five different types of roles available to you to use:
- Document structure roles: Exist to better describe the structure of your webpage where existing semantic HTML elements fall short.
- Widget roles: Are like document structure roles except, that most of them rely upon JavaScript to make them work – you'll be using a widget role in this step!
- Landmark roles: Are used by screen readers to help with keyboard navigation, so make sure to use these sparingly, as you can clutter up the experience for users with disabilities.
- Live region roles: Are especially important for users with impaired vision, as they exist to let the user know where things are updating on the page.
- Window roles: Exist to let the user know when a sub-window (like a pop-up modal) has been opened.
Each of these different types of roles come with their own benefits and best practices.
Here is an example of using an ARIA role to enhance the accessibility provided by an existing semantic HTML element:
<section role=”feed”>
This bit of HTML tells screen readers – here is a self-contained
section
of the webpage that is specifically afeed
. Afeed
is role that can be assigned to an HTML element that contains a list of articles that users can scroll through. In this way, you can make your page that much more accessible.It is important when using ARIA roles that you have a firm understanding of existing semantic HTML elements such as
<button>
,<main>
, and<figure>
. Many of the document structure roles need not be used in favor simply using the semantic HTML element equivalent.In the following tasks, you are going to start creating a menu button that, when clicked displays a list of action items that the user can interact with.
info> Note: It is important to not use ARIA roles when you don’t have to. For example, don’t use the “button” role on a
div
element when you could just use abutton
element instead! Many semantic HTML elements have built-in ARIA roles. Roles are a crucial part of implementing accessible web applications. In particular, structural roles are very helpful to users when used with non-semantic HTML elements such as thediv
element as they can give the user context concerning the actual function of thediv
in question. Whereas structural roles provide context around the structure of specific elements to the user, widget roles provide information regarding the interactivity of specific elements. Semantic elements contain their own, implicit roles that are used for accessibility. But, in certain cases where it might provide more context to the user, you can override the implicit role of the semantic element or remove the default role entirely. -
Challenge
### Making Your Web App Accessible Using States and Properties
In this step, you’re going to learn about the other side of ARIA attributes – states and properties. Whereas roles describe the inherent structure of your web page, states and properties describe the interactivity of your webpage. The ARIA state attributes are dynamic HTML attributes that change over time (usually via JavaScript). The ARIA property attributes are static, ARIA attributes that describe the inherent characteristic of an element in terms of accessibiliity.
Here is an example of the ARIA state attribute
aria-expanded
in action:<button aria-expanded=”false”>
This state attribute tells screen readers that this is an expandable button that is not currently expanded.
Here is an example of the ARIA property attribute
aria-label
:<button aria-label=”Open”>
This ARIA property attribute informs screen readers that this button is labeled “Open” - thus letting the user know that this button is used for opening another experience on the page.
The ARIA state and property attributes are vital to improving web accessibility when it comes to dynamic and interactive changes that take place. They are also an important means of providing more context to the user about how elements on the page are connected and how interactions are wired together. Another very useful ARIA property is the
aria-controls
attribute, as it lets users with disabilities know that certain elements (usually buttons) are in charge of the state of other elements. Yet another very useful ARIA attribute, thearia-label
attribute lets you associate a custom label to an element. This provides even more context for users around what a given element is and does. So far in this step, you’ve seen examples of ARIA properties, but ARIA states are also extremely useful when it comes to giving more context about the current state of elements on the page. Thearia-expanded
attribute governs the current open/close state of an element. Usually,button
elements contain this attribute and, in tandem with thearia-controls
attribute mixed with a little JavaScript, can accurately describe whether an element is expanded or not. -
Challenge
### Writing Tests for Accessible Web Apps
Unit testing ARIA implementations is absolutely crucial for ensuring that your web application is setup for success when it comes to accessibility. In this step, you are going to learn how to test ARIA roles, states and properties and ensure that they are being used properly in your web application. By unit testing ARIA implementations of various features, you are ensuring:
- That web accessibility is at the forefront of the considerations of your development team
- That your web application is truly accessible to people with disabilities
- That your application is guarded against regressions in accessibility
When writing good unit tests for ARIA you should be focused on not only ensuring that the correct roles exist for certain elements, but that the right states and properties exist and change appropriately based on your application state.
info> You can run these tests yourself in the console by running the command
npm test
in theworkspace
directory. When it comes to testing ARIA functionality on the webpage, one of the first things that you need to do is ensure that tested HTML elements have the correct IDs attached to them. This will not only help you to find these elements during programmatic testing, but it will also ensure that certain ARIA attributes, like thearia-expanded
attribute, work properly. It is also crucial to test your HTML elements and the ARIA roles that they are using. This will ensure that you encode your usage of ARIA roles into your test suite for your application which will help to guard against regressions and/or roles accidentally being removed from elements. In order to test attributes on HTML elements, you will need to use thegetAttribute
function that is available on HTML elements. Here is an example:myElement.getAttribute(“aria-controls”)
. -
Challenge
### Conclusion
Nicely done! You’ve made it to the end of this guided code lab on ARIA foundations.
In summary, you've learned:
-
How to use ARIA roles to ensure that the structure of your web application is accessible
-
How to declare ARIA attributes to link elements together and declare the function of your elements in an accessible manner
-
How to write unit tests to ensure that your website is adhering to ARIA principles correctly
You've barely scratched the service in terms of building applications using ARIA. As a follow up to your learning, it is recommended that you learn some of the more advanced topics within the ARIA ecosystem. Here are a just a few:
From here, you can be confident when it comes to using ARIA to help you build accessible and inclusive web applications. Please continue your journey learning ARIA by pursuing both video courses and more guided code labs here, at Pluralsight.
-
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.