- Lab
- Core Tech
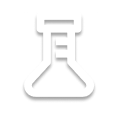
Guided: Angular Making HTTP Requests
Ready to power up your Angular skills? In this Guided: Angular Making HTTP Requests code lab, you’ll master RESTful API integration with HttpClient, perform CRUD operations, handle errors like a pro, and secure requests with interceptors. Through step-by-step tasks, you’ll build a task management app that connects to a mock API—perfect for beginners eager to create secure, data-driven Angular apps. Jump in and make your apps dynamic!
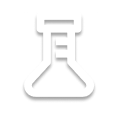
Path Info
Table of Contents
-
Challenge
### Introduction to RESTful APIs in Angular
Welcome to this Angular 19 lab where you’ll build a task management app from scratch! You’ll use Angular’s
HttpClient
to connect to a RESTful API, enabling your app to fetch, create, update, and delete tasks. You’ll perform CRUD operations (Create, Read, Update, Delete) to manage tasks, handle errors, and secure requests with HttpInterceptors to add authentication headers and log API calls. The goal is to create a dynamic app that interacts with an API, equipping you with skills for real-world Angular development.RESTful APIs are essential for modern web applications, allowing your app to retrieve and send data to servers. In this task manager,
HttpClient
will let you fetch tasks or save new ones instantly. Without API integration, your app would be static, unable to manage dynamic data.HttpInterceptors
and error handling are vital for secure and reliable apps. Interceptors will help you add authentication tokens and log requests, while error handling ensures your app gracefully recovers from API failures. Together, these tools help you build robust Angular applications.info> Should you get stuck at any time, the
solution
folder contains the full code solution for this lab!You can launch the app at anytime during the lab by doing the following;
- Click the Run button at the bottom right of the Terminal or run the command directly via
NG_CLI_ANALYTICS="false" npm start
. - Then view the web page by clicking this link to the Angular website: {{localhost:4200}}
- You can also view the webpage by clicking the Web Browser tab's Refresh button, once the app is running. It may take a moment for the page to load.
- Click the Run button at the bottom right of the Terminal or run the command directly via
-
Challenge
### Setting Up for RESTful API Integration
You’ll begin by setting up your project to integrate with a RESTful API. This step focuses on configuring the
HttpClient
provider and creating a service to handle API requests, laying the foundation for your task manager. These skills are critical for connecting your app to external data sources. Your task manager needs to communicate with an API to fetch and manage tasks. ProvidingHttpClient
enables HTTP requests, forming the backbone of API integration. You can provide theHttpClient
by way of theprovideHttpClient()
function out of the@angular/common/http
library. A dedicated service keeps your API logic organized and reusable. TheTaskService
- located in theapps
folder attask.service.ts
- will handle all task-related HTTP requests for the app. Creating data-access libraries like this is a best practice in Angular so that your application components are decoupled from your data layer. To interact with a RESTful API, your service needs the API’s endpoint. Configuring a mock API URL ensures your app can send requests to a reliable source. -
Challenge
### Performing CRUD Operations
Now you’ll implement CRUD operations to manage tasks in your app. This step focuses on using the
HttpClient
to perform GET (Read), POST (Create), PUT (Update), and DELETE (Delete) requests, enabling your task manager to fully interact with the API. These operations are the core of RESTful API integration. Fetching tasks is the first step in displaying them. A GET request retrieves the task list from the API. A GET request can be created via theget()
function on theHttpClient
instance. Users need to add new tasks. A POST request sends a new task to the API. A POST request can be created via thepost()
function on theHttpClient
instance. Editing tasks keeps the list up-to-date. A PUT request updates an existing task. A PUT request can be created via theput()
function on theHttpClient
instance. Removing tasks clears completed items. A DELETE request removes a task from the API. A DELETE request can be created via thedelete()
function on theHttpClient
instance. -
Challenge
### Handling Errors and Securing Requests
Next, you’ll enhance your task manager with error handling and secure API requests. This step focuses on catching API failures with
HttpErrorResponse
, retrying failed requests, and usingHttpInterceptors
to add authentication headers and log requests. These skills ensure your app is robust and secure. APIs can fail due to network issues or invalid requests. Handling errors withHttpErrorResponse
keeps your app user-friendly. Handling HTTP errors in Angular is as easy as using thecatchError
RxJs operator within the pipedObservable
returned by calling any one of the CRUD methods on theHttpClient
.This usually looks something like:
this.http.get('api').pipe( catchError((error: HttpErrorResponse) => { console.error(error); return throwError(() => new Error('Error!')); }) )
Note that the
catchError
operator returns anObservable
itself. It is customary to either rethrow the error usingthrowError
or to return a sane default using the RxJsof
operator. Retrying failed requests can recover from temporary issues. Using RxJS operators likeretry
, you can easily retry failed requests. Secure APIs often require authentication tokens. An AngularHttpInterceptor
can automatically add headers to requests. -
Challenge
### Conclusion and Next Steps
Great job! You’ve built a task management app with Angular's HTTP capabilities! You’ve completed a practical project that demonstrates your ability to integrate RESTful APIs, perform CRUD operations, handle errors, and secure requests. This lab has equipped you with valuable skills for building dynamic web applications.
You’ve learned how to integrate RESTful APIs using
HttpClient
, enabling your app to fetch and manage tasks seamlessly. With CRUD operations, you implemented GET, POST, PUT, and DELETE requests to fully interact with the API. Error handling withHttpErrorResponse
and retries ensured your app remains reliable, whileHttpInterceptors
added security and debugging capabilities with authentication headers and request logging. These skills are essential for creating real-world Angular applications.Now, consider your next steps to enhance this task manager. You could add a user interface with Angular Material to display tasks in a polished table. Another option is to explore advanced RxJS operators like
switchMap
for chained API calls or integrate a real API for a more complex project. If you’re up for a challenge, try implementing refresh tokens in the interceptor to handle expired authentication tokens.May this lab inspire you all to explore more Angular video courses and code labs to continue your learning journey, here at Pluralsight!
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.