- Lab
- Data
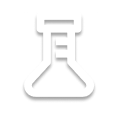
Create Dynamic Data Visualizations in R
In this lab, you’ll create advanced and dynamic scatterplot visualizations in R using ggplot2. You’ll enhance your visualizations with layering, annotations, and themes to produce polished plots, animated GIFs, a Shiny app, and a dashboard.
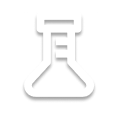
Path Info
Table of Contents
-
Challenge
Step 1: Load `ggplot2`
📊 Dynamic Visualizations in R
In this Code Lab, you'll work with advanced visualization capabilities to create dynamic visualizations all within R.
Dynamic in this case means moving! You'll be making GIFs, interactive visualizations, an App, and even a dashboard. ## 📚 Code Lab: Table of Contents
In this Code Lab, you will get hands-on experience with:
- Installing and loading packages
- Data preparation
- Layering with
ggplot2
- Scatter plots
- Labels and themes
- Interactive plots with
plotly
- GIF creation with
gganimate
- Shiny App
- Shiny Dashboard
If you're ready to go beyond a basic bar graph and to making your data move and be fully interactive, this is the place to be!
Overview of Step 1
In this step, you'll prepare the foundations of RStudio and how to set it up for data visualizations. You'll see the essential install package and load the data set you'll be working with today.
Installing and loading packages are foundational skills in R. Packages allow you to leverage powerful libraries for data analysis, visualization, and more.
✅ Note: Set Up for Success!
R packages expand the capabilities of your R environment by providing specialized functions, datasets, and tools. By installing and loading the right packages, you can tackle a variety of visualization projects. ### Main Package and Data Set:
ggplot2
andgapminder
📊
ggplot2
is a powerful R package for data visualization, built on the “Grammar of Graphics”. It makes creating and customizing plots straightforward by layering code, customizations, and the ability to animate graphs.🌍
gapminder
is an open-source data set. It provides real-world country-level data, life expectancy, GDP per capita, and population, which makes it ideal for practicing data manipulation and showcasing yourggplot2
skills!Basics: Install and Load Package and Data
When you do a project setup, typical package installation consists of the two parts of installing and bringing the package into the library:
install.packages("ggplot2") library(ggplot2)
You would install packages and load them into the library for usage.
Setup and Ready to Go!
In this Code Lab through the Pluralsight console, all packages have been preinstalled for you. Keep in mind, in your local environment, you would need to do each install separately. ## Navigating the RStudio IDE For all tasks, you will be using the corresponding files on the right. To run your code, do the following:
- Click your cursor on the line you want to run.
- Press
Control
+Enter
on your keyboard:
- On Windows/Linux: Hold the
Control
key and pressEnter
. - On Mac: Hold the
Command
(⌘
) key and pressEnter
.
Installation Code for ggplot2
andgapminder
install.packages("ggplot2") install.packages("gapminder")
Task 1.1: Load
ggplot2
andgapminder
In the
dynamicPlots.R
file, loadggplot2
andgapminder
, then print the data set.Task Instructions:
- Load
ggplot2
andgapminder
using thelibrary()
function. - Print the
gapminder
data set to the console.
🔍 Hint
- Use
library(package)
to load the package. - Type
gapminder
and run to display the dataset.
🔑 Solution
library(ggplot2) library(gapminder) gapminder
✅ Avoid Common Issues
- Use a
.R
file and save it for the future to reuse code! - Know which packages have what functionality and add comments so they're easy to reference later.
- Before spending lots of time debugging, check you have loaded all of the right packages. It's an easy and common error that eats time.
- Want to see demo recordings of this course or review its concepts? Check out the companion course to this Code Lab, Advanced Data Visualization Techniques in R. Pluralsight has you covered!
With the packages and data set ready, you can create dynamic visualizations and work with various datasets.
Click Next step to keep building your data visualization skills!
-
Challenge
Step 2: Prepare Data
Prepare Data
Overview
In this step, you'll get the data ready to be graphed. In the
gapminder
package, there is a data set calledgapminder
that includes data on life expectancy, GDP per capita, and population by country.✅ Note: Data Sets!
ggplot2
graphs data. The code that customizes the graphs can get very long and unwieldy! By formatting data effectively, you can be efficient with what you plot.Task 2.1: Map Data to Variable
In the
dynamicPlots.R
file, make your code more efficient by assigning the data setgapminder
to a new variable.Task Instructions:
- Assign
gapminder
to a variable namedd
.
🔍 Hint
- Use the assignment operator
<-
in R. - The syntax looks like:
new_variable <- existing_data
.
🔑 Solution
d <- gapminder
✅ Data Sets
- Know what is in your data set.
- Preview your data to see column headers.
- Clean data if needed.
- Create different variables for data subsets. This is helpful for efficient code and keeping many versions ready to go!
You’ve got your packages installed, loaded, and your data set ready.
Click Next step to create a scatter plot!
- Assign
-
Challenge
Step 3: Create Scatter Plot and Introduce Layering
Create a Scatter Plot and Introduce Layering
Overview
In this step, you'll create a scatter plot and learn the layering technique used throughout
ggplot2
.✅ Note: Layering
ggplot2
syntax uses a technique called layering. This allows the code to get longer and longer and customize different aspects of graphs. ## Syntax of Scatter Plots inggplot2
You can create your first scatter plot with these packages and data set.
To create a scatter plot in
ggplot2
, use the following syntax:variable_name <- ggplot(dataset, aes(x = variable1, y = variable2)) + geom_point()
This
ggplot2
code is your base layer for your visualizations.ggplot2
needs a dataset, requires you to specify which variables to map on to the x and y axis, and what type of graph you want to make.To make a scatter plot, you can use
geompoint()
.Layering syntax in
ggplot2
is a new line of code connected by+
. You can type it out long, but most people prefer to have each line of code underneath each other with the line ending in+
for readability.✅ Note:
ggplot2
vsggplot
The current package is named
ggplot2
, the main function in the code isggplot
. The2
indicates it is the second version of the package.Now you can make your first scatter plot!
Task 3.1: Create a Simple Scatter Plot
In the
dynamicPlots.R
file, usesimple
as the name of your scatter plot. Be sure to follow theggplot2
syntax.Task Instructions:
- Reference the syntax for a scatter plot in
ggplot2
. - Set
gdpPercap
as the x-axis variable. - Set
lifeExp
as the y-axis variable. - Assign the plot to a variable named
simple
. - Print the graph.
🔍 Hint
- Use
ggplot(data, aes(x = ..., y = ...)) + geom_point()
for a scatter plot. - Use
simple
as the object name. - Print the plot by typing
simple
on a new line.
🔑 Solution
simple <- ggplot(d, aes(x = gdpPercap, y = lifeExp)) + geom_point() simple
<p>Congrats! You've printed your scatter plot. Is it the most beautiful graph you've ever seen? </p> <p>Probably not, but it's a good starting graph for all the customizations you're going to make in later steps. That's why you named it <code>simple</code>.</p>
Pro Tips
✅ Layering
- Give you graphs descriptive names to help remember what they are.
- Customize graphs and create new variable names for subsequent changes. This helps avoid bugs and keep different versions easy to access.
- Comment and document which layers and customizations work for you for future reference.
Now customize your graph with more layers. Click Next step to embellish your graph!
- Reference the syntax for a scatter plot in
-
Challenge
Step 4: Add Labels, Colors, and Themes
Add Labels, Colors, and Themes
Overview
In this step, you'll add labels to the scatter plot. You'll create a graph title and label the x and y axes. You will add color to your graph. Finally, you'll load
ggthemes
to expand the number of themes you can use to add to the graph.You've got several tasks in this step, but none of them are hard. They build on each other.
✅ Note: Labels, Colors, and Themes
Labeling a graph effectively can make it more professional and easy to read by others! Themes are an efficient way to polish graphs. Colors are great if they're descriptive and not distracting. ## Labels
The syntax of labels is a layer in
ggplot2
code that starts withlabs()
, see below.The graph's title is assigned as well as the labels for the x axis and y axis.
labs( title = "Life Expectancy Over Time by Continent", x = "Year", y = "Life Expectancy" )
Reviewing Scatter Plot Syntax
By using this efficient code above, you can retain the main scatter plot underlying code and layers and make other customizations.
All of the code is now in that variable you've seen before:
simple <- ggplot(d, aes(x = gdpPercap, y = lifeExp)) + geom_point() simple
Task 4.1: Add Labels to Scatter Plot
In the
dynamicPlots.R
file, enhance the scatter plot by adding a title, axis labels, and adjusting point transparency. Assign the result to a new variablelabeledPlot
.Task Instructions:
- Use the code from the
simple
scatter plot as a reference. - Add a title, x-axis label, and y-axis label using
labs()
. - Assign the plot to a new variable named
labeledPlot
. - Print the
labeledPlot
plot.
🔍 Hint
- Use
labs(title = ..., x = ..., y = ...)
to add labels. - The syntax follows the same pattern as the
simple
plot, just with more layers.
🔑 Solution
labeledPlot <- ggplot(d, aes(x = gdpPercap, y = lifeExp)) + geom_point() + labs(title = "Life Expectancy Over Time by Continent", x = "Year", y = "Life Expectancy") labeledPlot
Notice your code getting longer and longer and connected with
## Colors+
syntax. This is why staying efficient and organized with code is helpful as the complexity of plots increases!The
ggplot2
package makes it easy to add color to your plots with an extremely small amount of code.You’ve already created scatter plots with scaling and labels. Now, you can take them further by assigning a variable to the color aesthetic. This makes the plot more informative and visually engaging.
Syntax: Adding
color = variable
inaes()
maps colors to the values in that column. This is especially useful for distinguishing groups, categories, or continents, for example.Here's an example:
ggplot(data, aes(d, x = x_var, y = y_var, color = group_var)) + geom_point()
This tells
ggplot2
to automatically assign a color to each unique value.Task 4.2: Add Color to the Plot
In this task, you’ll make your scatter plot more informative by mapping a variable to color.
Instructions:
- Create a new scatter plot using the
d
dataset. - Map
gdpPercap
to the x-axis andlifeExp
to the y-axis. - Use
color = continent
insideaes()
to visually separate data points by continent. - Assign the plot to a new variable named
colorPlot
. - Print
colorPlot
to see your colorful plot in action!
🔍 Hint
- Use
color = continent
inside theaes()
mapping. - Be sure to use
geom_point()
to create the scatter plot.
🔑 Solution
colorPlot <- ggplot(d, aes(x = gdpPercap, y = lifeExp, color = continent)) + geom_point() colorPlot
Wow, does that make a world of difference! Get it? 🌍🎨
## Themes The R package, `ggthemes`, provides pre-built themes that make plots look more professional without requiring complex custom styling and many lines of layering code.You have now created scatter plots with scaling and labels. You can easily make them look better.
🎨 Themes add 🖼️ borders, 📜 backgrounds, and 🔠 font. Instead of adding many layers, themes are efficient in making plots look professional.
There are many themes to choose from in these packages.
If you type
theme_
a list of options will appear that are compatible withggplot2
.Installation Code for
ggthemes
Packageinstall.packages("ggthemes")
Adding a theme to a graph can be even more efficient if you save your graph to a variable. With the scatter plot p2, you can then add a theme by adding the theme afterward.
You can assign this additional layer plus p2 to a new plot,
clean
.cleanPlot <- colorPlot + theme_clean() cleanPlot
This is a helpful last step when adding a graph. An issue with this technique is you cannot see all the code within
cleanPlot
if changes need to be made to that code.Naming Variables for Readability
Variable names that are descriptive, such as
cleanPlot
, can make the code more readable and help you know which theme is incorporated if you put down and pick up work later.Task 4.3: Add a Theme
In this task, you'll enhance the scatter plot by applying a visual theme using
theme_economist()
.Instructions:
- Load
ggthemes
. - Reference the code above. Use the plot
colorPlot
and create a new plot calledeconPlot
, - Add the layer
theme_economist()
to the scatter plot. - Assign the updated plot to a new variable named
econPlot
. - Print
econPlot
to see the graph.
🔍 Hint
- Use
library(package)
to load it into your R session. - Use the
+
operator to addtheme_economist()
to the end of your ggplot chain. - Ensure the
ggthemes
package is installed and loaded before using the theme.
🔑 Solution
library(ggthemes) econPlot <- colorPlot + theme_economist() econPlot
<p>What changes do you see with the theme?</p>
🎨 Applying
# Pro Tipstheme_economist()
adds a blue background and simplifies the visual style that is clean and professional! Feel free to test out other themes while you're here.✅ Themes
- Try typing
color =
in your plot code. RStudio will help autocomplete variable names available in your dataset! - On the same plot, test out many themes.
- Do research on which themes are appropriate for your field and workplace.
- Document the themes that work well for you and use them consistently.
Now you will take these professional plots on the road! You can make them interactive.
Click Next step to make your first interactive plot.
- Use the code from the
-
Challenge
Step 5: Make Visuals Interactive with `plotly`
Interactive Plots with
plotly
Overview
In this step, you'll work with
plotly
to take static plots and make them interactive! R is best known for making advanced plots, but fewer people know about the dynamic and interactive visualizations you will see in this Code Lab.✅ Note: Interactive
A great way to engage stakeholders is by including them in the process. Gamifying content by making it interactive can help you get buy-in about your projects and conclusions. ### Syntax of
plotly
The syntax for making a plot interactive in
plotly
builds on top of an existingggplot2
object using the functionggplotly()
.This function wraps your
ggplot
chart and transforms it into an interactive version with tool tips, zoom, pan, and more. Imagine you're working with a graph calledp2
.You could make it interactive with this code:
ggplotly(p2)
This approach helps you build once, then enhance with interactivity using just one line of code.
Installation Code for
plotly
install.packages("plotly")
Next, make your plots interactive! ✨📊
Task 5.1: Make an Interactive Plot
In the
dynamicPlots.R
file, you will useplotly
to make your existingggplot2
chart interactive.Task Instructions:
- Load the
plotly
library usinglibrary()
. - Make the plot
colorPlot
interactive using the syntaxggplotly(plotName)
.
🔍 Hint
- Use
library(package)
to make it available for use. - Use syntax
ggplotly(graph)
.
🔑 Solution
library(plotly) ggplotly(colorPlot)
Now in the bottom right quadrant of RStudio, you can interact with your plot!
🖱️ Hover: When you hover your cursor over the data points, what do you notice?
🔍 Zoom: When you zoom in to specific data points, what do you see?
🖼️ Pop-up: Feel free to click Zoom for a pop-up that is larger than what you see in RStudio.
🗣️ Communicate: How could you leverage this to speak to other stakeholders about the data?
Export the Graph 💾📈
plotly
allows you to export the graph to PNG. Test this out for yourself to get a specific lasso around some data and export it for later use! # Pro Tips✅ Interactive Plots: Helpful or gimmicky?
- Interactive plots can be helpful, but avoid them if it seems too gimmicky for your workplace.
- A key component of whether this is the right fit for the context is how stakeholders react and interact with plots they can change.
- See if interactive plots or dashboards can be helpful to your work. Sometimes interactive plots are overkill for your stakeholder and a static plot is a better fit.
Nice! You've made our first interactive plot. Now, you will go further and make a GIF!
Click Next step to make a time-based dynamic GIF in R.
- Load the
-
Challenge
Step 6: Create GIF with `gganimate`
Create a GIF with
gganimate
Overview
In this step, you'll work with
gganimate
to create a GIF of a time-based plot! Seeing data in action can help with data storytelling and effective presentations.✅ Note: GIF
While most people think about GIFs in the context of social media memes, they also can be a powerful tool in professional settings for showing data across time.
Installation Code for GIF Packages
install.packages("gganimate") install.packages("gifski")
Task 6.1: Load GIF Packages
In the
dynamicPlots.R
file, load thegganimate
andgifski
packages to enable GIF creation for animated plots in R.Task Instructions:
- Load the
gganimate
package. - Load the
gifski
package.
🔍 Hint
- Use syntax
library(package)
to load packages.
🔑 Solution
library(gganimate) library(gifski)
To format your plot to become a GIF, you need to define which variable changes the graph over time. In this example, you use year as your changing variable.
The code
labs()
is used to label the graph overall. Notice how you can use a dynamic variable that changes with the graph for the label also. How fancy!plotGIF <- p + transition_time(timeVariable) + labs(title = "Year: {frame_time}") plotGIF
Graph Speed
Frame per second (fps) is default if you do not define it in your code. You can make the GIF go faster or slower by defining that later.
Task 6.2: Create GIF
In this task, you'll use
gganimate
to make your main plotcolorPlot
a GIF.Task Instructions:
- Create a variable called
plotGIF
that animates the existing plotcolorPlot
. - Use syntax
transition_time(year)
to animate across theyear
variable. - Use
labs(title = "Year: {frame_time}")
to dynamically show the year in the title. - Print
colorPlot
to see the animated GIF play at the default frames per second (fps).
🔍 Hint
- Your code will look something like:
- Then just type
plotGIF
to run it.
plotGIF <- plotName + transition_time(year) + labs(title = "Year: {frame_time}")
🔑 Solution
plotGIF <- colorPlot + transition_time(year) + labs(title = "Year: {frame_time}") plotGIF
🎉 Watch your data come to life with animation magic!
Task 6.3: Change GIF Speed
In this task, you'll adjust the speed of your animated GIF by changing the number of frames per second (fps).
Task Instructions:
- Use the
animate()
function to control the animation speed. - Create a new variable called
fast
using theplotGIF
from the previous task. - Set
nframes
to200
andfps
to50
to increase the playback speed. - Print the variable
fast
to see the faster animation in action.
🔍 Hint
- You're using
animate()
to control how many frames play per second. - More
fps
= faster animation!
🔑 Solution
fast <- animate(plotGIF, nframes = 200, fps = 50) fast
⚡ Nice work! You’ve just made your animation speed up, fast, and smooth!
Task 6.4: Export GIF
You've created a great animated plot — now it's time to save it as a GIF file so it can be shared or used outside of R!
Task Instructions:
- Use the
animate()
to export the GIF. - Use
gifski_renderer("nameOfGIF.gif")
to define the file name you're exporting. - Export the
plotGIF
animation. - Name the output file
plotGIF.gif
.
Note: Yes, the name
plotGIF.gif
includes the word "GIF" and the file extension.gif
. It may feel a little redundant, but it's correct for this context!🔍 Hint
- The GIF name can be anything, just make sure it ends with
.gif
. gifski_renderer()
tells R how to render and save the file.
🔑 Solution
animate(plotGIF, renderer = gifski_renderer("plotGIF.gif"))
💾 Sweet! Your animated masterpiece is now saved and ready to share!
# Pro Tips✅ GIF Tips
- A common issue related to creating a GIF can be about directories.
- Make sure your data is set up correctly to be formatted to create changes over time that can be made into a GIF.
- It is not ideal to make a GIF just for the sake of making a GIF in professional contexts. If it's helpful, you now know how to make them!
Ready to take it a step further? Next, you will make a Shiny app.
Click Next step to make an app through R using Shiny.
- Load the
-
Challenge
Step 7: Create Basic Shiny App
Create Shiny App
Overview
In this step, you'll make a Shiny App through R that allows you to toggle an interactive visualization app, all through R!
✅ Note: Shiny Apps
Apps are easier to create than you might think! Another way of making content interactive is by creating a lightweight Shiny app in R.
Installation Code for Shiny App Packages
install.packages("shiny") install.packages("ggplot2") install.packages("gapminder") install.packages("dplyr")
Task 7.1: Load Shiny App Packages
In the
07ShinyApp.R
file, you'll load the packages needed to build interactive web apps using Shiny, along with data visualization and manipulation tools.Task Instructions:
- Load the following packages:
shiny
,ggplot2
,gapminder
, anddplyr
.
🔍 Hint
- Use
library(packageName)
to load them into your session.
🔑 Solution
library(shiny) library(ggplot2) library(gapminder) library(dplyr)
Awesome! You’ve got all the tools ready to build something interactive and data-driven.
Task 7.2: Create Shiny UI
In this task, you'll define the user interface (UI) for your Shiny app that allows users to interact with Gapminder data.
You might find this code is longer and more cumbersome. That's the reality of building an app!Follow the task instructions to define what the user will see, titles, which variables you're visualizing, and what happens when you move the slider to visualize data differently.
Task Instructions:
- Create a variable named
ui
using thefluidPage()
function. - Within the
fluidPage()
function, usetitlePanel()
and give the title of the application to be"Gapminder Data Visualization"
. - Next, use
sidebarLayout()
to create a layout with inputs on the left and a plot on the right. - Inside
sidebarPanel()
, include: - A
selectInput()
for choosing the continent, with the option "All" and unique values fromgapminder$continent
. This shows how the app will filter the data by continent. - A
sliderInput()
for selecting the year, with min and max values fromgapminder$year
, default value of2007
, step size of5
, and animation set toTRUE
. - Inside
mainPanel()
, add aplotOutput("scatterPlot")
to display the plot. Be sure to separate each UI section with commas.
🔍 Hint
- Make sure to wrap your UI components inside
fluidPage()
. - Use
gapminder$continent
andgapminder$year
to set up the input options dynamically.
🔑 Solution
# Define UI ui <- fluidPage( # Application title titlePanel("Gapminder Data Visualization"), # Sidebar layout with input controls sidebarLayout( sidebarPanel( # Dropdown to select continent selectInput("continent", "Select Continent:", choices = c("All", unique(gapminder$continent))), # Slider to select year sliderInput("year", "Select Year:", min = min(gapminder$year), max = max(gapminder$year), value = 2007, step = 5, animate = TRUE) ), # Main panel for displaying the plot mainPanel( plotOutput("scatterPlot") ) ) )
Nice! You’ve created a user-friendly interface for your app. Up next: add the server logic! 🛠️
Task 7.3: Add Server Logic
Now that you've set up the user interface, it's time to define what your Shiny app actually does behind the scenes. In this task, you'll write the server logic that responds to user inputs and renders a dynamic plot based on the Gapminder dataset.
Remember: This is where your app comes to life! 🧠✨ You're telling Shiny how to filter data and create visuals depending on what the user selects.
Task Instructions:
- Start by creating a variable named
server
. Set it equal to a function with two inputs:input
andoutput
. This is how Shiny knows what users are doing and what it should show on the screen. - Inside the server function, you want to build the plot. Use
output$scatterPlot <- renderPlot({ ... })
to tell Shiny: “Here’s what to draw on the screen when things change.” - Now filter the data so it shows the right info:
- Start with all rows where the
year
matches what the user picked (input$year
). - If the user chose a specific continent (not "All"), filter it again to only include that continent.
- Start with all rows where the
- Use
ggplot()
to draw a scatter plot using this filtered data:- X-axis:
gdpPercap
(you’ll log scale it so the values are easier to compare) - Y-axis:
lifeExp
- Color: Based on
continent
- Size: Based on population (
pop
)
- X-axis:
- Add labels and use a clean style with
theme_minimal()
so the plot looks nice and easy to read.
🔍 Hint
- Use
if (input$continent != "All")
to check whether the user chose a specific continent. scale_x_log10()
will make the GDP values easier to compare — since GDP varies a lot across countries.
🔑 Solution
#Define server logic server <- function(input, output) { output$scatterPlot <- renderPlot({ # Filter data based on user input filtered_data <- gapminder %>% filter(year == input$year) if (input$continent != "All") { filtered_data <- filtered_data %>% filter(continent == input$continent) } # Create the scatter plot ggplot(filtered_data, aes(x = gdpPercap, y = lifeExp, size = pop, color = continent)) + geom_point(alpha = 0.7) + scale_x_log10() + # Log scale for GDP labs(title = paste("Gapminder Data for", input$year), x = "GDP per Capita (Log Scale)", y = "Life Expectancy", color = "Continent", size = "Population") + theme_minimal() }) }
Awesome job! Your app can now respond to users and visualize the data interactively. Move on to the next task to run your app! 🚀
Task 7.4: Run the Shiny App
In this task, you'll run your complete Shiny application by connecting the UI and server components together.
Task Instructions:
- Use the
shinyApp()
function to launch your app. - Pass in the UI and server variables you’ve defined in the previous tasks:
ui
andserver
, make sure to separate by comma.
🔍 Hint
- The function takes two arguments:
ui
andserver
. - Make sure both
ui
andserver
have already been defined before this step.
🔑 Solution
shinyApp(ui = ui, server = server)
To run your app, click the Source button and then Run App.
🎉 That’s it! Your interactive Shiny app is now live and ready to explore. Great work!
Pro Tips
✅ Shiny App
- Apps might be easier to create than you think!
- Interact with your data in a new way.
- Get feedback from stakeholders about how useful this type of plot and interactivity are to others.
Last step up next! Go one step further on the Shiny app and make a Shiny dashboard.
Click Next step to make a professional dashboard!
- Load the following packages:
-
Challenge
Step 8: Create Shiny Dashboard
Create Shiny Dashboard
Overview
In this step, you'll go from a Shiny app to a Shiny dashboard with R. Shiny can make a basic app. In this step, you'll make a dashboard that is a more professional and looks closer to Tableau and Power BI product dashboards.
This is one of the most complicated steps because you're building on your knowledge from the last steps and also incorporating some sophisticated data filtering techniques.
You don't have to understand each line of code here. The concept is that you see it's possible to create a dashboard from R that opens your mind to possibilities for projects for yourself.
✅ Note: Shiny Dashboards
These dashboards can be done offline and can also be moved to a browser. See your data move across time in this new UI.
Installation Code for
shinydashboard
Packageinstall.packages("shinydashboard")
Task 8.1: Load
shinydashboard
In the
08ShinyApp.R
file, you'll load theshinydashboard
package, which helps create professional-looking dashboards in Shiny.Task Instructions:
- Load the
shinydashboard
package using thelibrary()
function.
🔍 Hint
- Use
library(shinydashboard)
to load it for use in your project.
🔑 Solution
library(shinydashboard)
Note: The Shiny dashboard builds on the Shiny app. It requires all the same packages. If you didn't install and load the packages from the last step,
shiny
,ggplot2
,gapminder
, anddplyr
, this step won't run properly.
- Load the
- Create a variable named
ui
usingdashboardPage()
. - In the
dashboardHeader()
, set the title to"Gapminder Dashboard"
. - Inside
dashboardSidebar()
: - Use
sidebarMenu()
with amenuItem()
labeled "Plots", settabName = "plots"
, and use an appropriate icon (likeicon("chart-line")
). - Add a
selectInput()
with:inputId = "continent"
label = "Select Continent(s):"
choices = unique(gapminder$continent)
selected = "Asia"
multiple = TRUE
to allow multiple selections
- Inside
dashboardBody()
: - Use
tabItems()
and include atabItem
withtabName = "plots"
. - Inside the tab, create a
fluidRow()
containing abox()
: - Set
title = "Life Expectancy Over Time"
- Set
width = 12
for full width - Include
plotOutput("lifeExpPlot")
inside the box - Wrap
menuItem()
insidesidebarMenu()
. box()
helps organize content with a header and border.- Use
plotOutput()
to display plots rendered from the server. - The code uses the
%>%
pipe operator fromdplyr
, which allows you to write clean, readable code that chains operations together. gapminder %>% filter(continent %in% input$continent)
means:- Start with the
gapminder
dataset. - Filter it to only include rows where the
continent
is one of the values selected by the user (via theselectInput
in the UI). - The result is saved to a new variable called
data_filtered
.
- Start with the
- This filtered data is then passed to
ggplot()
to create the visual output. - Define a
server
function withinput
,output
, andsession
as arguments. - Inside the server, create a
renderPlot()
output namedlifeExpPlot
. - Use
req(input$continent)
to ensure the user has selected at least one continent. - Use
dplyr::filter()
to create adata_filtered
object that only includes the selected continents. - Use
ggplot2
to create a line plot showing life expectancy over time, grouped by country and colored by continent. req()
is useful for stopping the plot from rendering if no input is selected.geom_line(aes(group = country))
helps keep country-level lines separate within each continent.- Call the
shinyApp()
function. - Pass in your
ui
andserver
objects as arguments. - Once run, this will open your dashboard in the RStudio Viewer or in your web browser.
- You must define both the
ui
andserver
first — this function combines them to launch the app. - If you’ve followed all previous steps, you can run this at the end of your script.
- Dashboards might be easier to create than you think!
- Similar to Shiny apps, get feedback from stakeholders about this experience. Is it helpful? Confirm before investing lost of time in them.
- Installing and loading packages
- Data preparation
- Layering with
ggplot2
- Scatter plots
- Labels and themes
- Interactive plots with
plotly
- GIF creation with
gganimate
- Shiny app
- Shiny dashboard
✅ Awesome! You're now ready to build dashboards that look sleek and interactive! 📊
Task 8.2: Create Dashboard UI
In this task, you'll use shinydashboard
to build a clean and organized dashboard layout.
Task Instructions:
🔍 Hint
🔑 Solution
ui <- dashboardPage(
dashboardHeader(title = "Gapminder Dashboard"),
dashboardSidebar(
sidebarMenu(
menuItem("Plots", tabName = "plots", icon = icon("chart-line"))
),
# Sidebar input to select continent(s)
selectInput(
inputId = "continent",
label = "Select Continent(s):",
choices = unique(gapminder$continent),
selected = "Asia",
multiple = TRUE
)
),
dashboardBody(
tabItems(
tabItem(tabName = "plots",
fluidRow(
box(
title = "Life Expectancy Over Time",
width = 12,
plotOutput("lifeExpPlot")
)
)
)
)
)
)
🎉 Well done! You've structured a dynamic and interactive UI. Now it’s time to wire up the server logic and bring your dashboard to life! 🚀
Task 8.3: Define Server Logic
In this task, you'll write the server-side logic for your Shiny dashboard to render a dynamic plot based on user input. This is where the actual data processing and plotting happen.
The server
function connects your UI components with data and computations. It listens for user inputs and updates outputs like plots in real time. Here, you’ll use the dplyr
package to filter data based on the selected continent(s), and ggplot2
to create the plot.
Understanding the Flow with dplyr
:
Task Instructions:
🔍 Hint
🔑 Solution
# Define server logic
server <- function(input, output, session) {
output$lifeExpPlot <- renderPlot({
req(input$continent) # Ensure there's at least one continent selected
# Filter the gapminder data by selected continents
data_filtered <- gapminder %>%
filter(continent %in% input$continent)
ggplot(data_filtered, aes(x = year, y = lifeExp, color = continent)) +
geom_line(aes(group = country), alpha = 0.5) +
labs(
title = "Life Expectancy Over Time",
x = "Year",
y = "Life Expectancy",
color = "Continent"
) +
theme_minimal()
})
}
🎉 Well done! You’ve just connected user input to a fully dynamic plot using the power of dplyr
and ggplot2
! 🌍📈
Task 8.4: Run the Shiny Dashboard
Now that you’ve defined both the ui
and server
components of your dashboard, it’s time to launch your app and see everything come together!
This step uses the shinyApp()
function, which takes the user interface and server logic you’ve built and runs the app locally in your R session.
Task Instructions:
🔍 Hint
🔑 Solution
shinyApp(ui, server)
To run your app, click the Source button and then Run App.
🚀 That’s it! You’ve built and launched an interactive dashboard using Shiny and shinydashboard
. Amazing work! 🎯📊
✅ Shiny Dashboard
--- # More ggplot2
✅ ggplot is powerful!
With this knowledge of
ggplot2
, you can build on more layers and customization for meaningful and helpful plots.See more documentation for ggplot2.
--- # Congrats! 🎉 Wow, congrats! You completed the Create Dynamic Data Visualizations in R!
You've learned so much about ggplot2
's capabilities.
ggplot2
is customizable and flexible to make advanced visualizations that are dynamic and interactive.
In this Code Lab, you got hands-on experience with:
With GIFs, animations, and dashboards, now you can create impressive, customized, professional plots that are dynamic for projects at work and beyond.
Congrats on making interactive plots in R.
Ready to try the code with your own data set?
Load the data set in and begin customizing the graphs for your own projects!
See you in the next Code Lab! 🎉 # Demos and Visualizations
✅ See demos and learn more?
Want to learn more about advanced visualizations in R and see live demos? Check out the companion course to this Code Lab, Advanced Data Visualization Techniques in R.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.