- Lab
- Core Tech
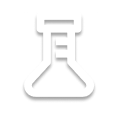
Challenge: Building an Auction Application with Spring Framework 6
This lab is designed as a Challenge Lab, you'll start with several requirements and build out a basic Spring Framework application and gradually move to more complex concepts. By the end of this Lab you will have a working auction application that you can use as a reference for future projects.
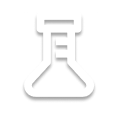
Path Info
Table of Contents
-
Challenge
Introduction
In this Lab, you'll build a comprehensive Spring Boot application for an auction system. Starting with the
AuctionApplication
class as your foundation, you'll define theItem
entity class that represents an auction item in a Java Persistence API (JPA) repository. You'll also create aCrudRepository
interface to handle CRUD operations for our Item entity.Next, you'll construct two Spring MVC Controllers:
ItemController
for fetching items and populating the model, andAdminController
for managing administrative tasks for items.Finally, you'll create Thymeleaf templates to dynamically render your list of auction items (
index.html
), present an admin view with functionalities (admin.html
), and provide a form for creating or editing items (form.html
). This Lab will give you a thorough understanding of building a functional Spring Boot application, combining Spring MVC, Spring Data, and Thymeleaf templates.Running the Application: This project uses gradle so to run the application use the command
gradle bootrun
from the Terminal. There may be some points where you run into compiler errors that will prevent the application from starting. If that happens check the solutions. Once the application is running it can be view in the Web Browser tab. -
Challenge
Application Class
The
AuctionApplication
class lies at the heart of your Spring Boot application. Annoted with@SpringBootApplication
, this class seamlessly configures Spring for you while enabling component scanning. TheSpringApplication
class from the Spring Boot framework is your key to bootstrapping a Spring application from a Java main method. To run your application, simply call therun()
method from theSpringApplication
class. This nifty method returns aConfigurableApplicationContext
that you can tuck away for later use.Relevant File: /src/main/java/com/pluralsight/auction/AuctionApplication.java
Instructions
- Import the SpringApplication Class: Import the
SpringApplication
class from the Spring Boot framework. This class is used to bootstrap and launch a Spring application from a Java main method. - Run the Application: Start the Spring application. This is done by calling the static method
run()
from theSpringApplication
class. This method returns aConfigurableApplicationContext
that you can use later if needed. But for now, just start the application by passing two arguments to therun()
method.
- Import the SpringApplication Class: Import the
-
Challenge
Item Entity Class
The
Item
entity class is a crucial part of our auction setup, serving as an Entity class for a JPA (Java Persistence API) repository. This class represents an auction item, complete with properties likename
,description
,seller
,price
, andreserve
. To access these properties, you'll equip your class with getters and setters. Plus, you'll design a constructor that accepts parameters for each property, making it a breeze to create newItem
instances.Relevant File: /src/main/java/com/pluralsight/auction/Item.java
Instructions
- Define Properties:
Define the properties for the
Item
entity. These properties should includename
,description
,seller
,price
, andreserve
. Remember to specify the correct data types for each property. - Create Getters and Setters: After defining the properties, your next task is to create getters and setters for each property. These methods will allow other parts of your code to read (get) or modify (set) the values of your properties.
- Define a Parameterized Constructor:
Finally, you should define a constructor that accepts parameters for each property (except the
id
, which is automatically generated). This constructor will make it easy to create newItem
instances with specific values.
- Define Properties:
Define the properties for the
-
Challenge
Spring Data CrudRepository Interface
In this step, you'll be crafting a Spring Data repository interface that can handle the CRUD operations for your
Item
entity. TheCrudRepository
interface from Spring Data is your ticket to these operations, and yourItemRepository
interface will extend this, specifyingItem
andLong
as the type parameters.Relevant File: /src/main/java/com/pluralsight/auction/ItemRepository.java
Instructions
- Import the CrudRepository Interface: Import the
CrudRepository
interface from Spring Data. This interface provides several methods for common CRUD operations. You can do this by typingimport org.springframework.data.repository.CrudRepository;
at the top of your file. - Define the ItemRepository Interface: Define an interface named
ItemRepository
. This interface should extendCrudRepository
, which requires two type parameters: the entity class and the type of the entity ID. In this case, you'll useItem
andLong
.
- Import the CrudRepository Interface: Import the
-
Challenge
Item Controller
Next, you'll build a Spring MVC Controller that utilizes the
ItemRepository
to fetch items. It then populates the model with these items for rendering in the view. To achieve this, you'll rely on theAutowired
,Model
,GetMapping
, and theItemRepository
classes and interfaces.Relevant File: /src/main/java/com/pluralsight/auction/ItemController.java
Instructions
- Import Required Classes: Import all the required classes and interfaces. These include
Autowired
,Model
,GetMapping
, and theItemRepository
interface that you created in the previous module. - Inject the ItemRepository: Use dependency injection to get an instance of
ItemRepository
. You can use the@Autowired
annotation to do this. Also, create a constructor to set theItemRepository
instance to a class level variable. - Define the listItems Method: Define a method named
listItems
that will handleGET
requests at the root URL ("/"). This method should use theItemRepository
to fetch all items, add them to the model, and then return the name of the view that should be rendered ("index").
- Import Required Classes: Import all the required classes and interfaces. These include
-
Challenge
Thymeleaf template: `index.html`
In this step, you'll create a Thymeleaf template named
index.html
that will serve as the canvas for rendering our list of auction items. The body of this page will house a<main>
element, within which you'll nest a<section>
. This section will then generate an<article>
for each item in theitems
attribute of the model.Relevant File: /src/main/resources/templates/index.html
Instructions
- Create the Main Content Area: In the body of the page, create a
<main>
element. Inside this element, create a<section>
. - Display the Auction Items: In the section, create an
<article>
for each item in theitems
attribute of the model (which was set in theItemController
). For each article:- Display the item's name in a
<h3>
. - Display the item's description in a
<p>
. - Display the item's price and seller in a
<footer>
. Use theth:utext
attribute to generate the content.
- Display the item's name in a
- Create the Main Content Area: In the body of the page, create a
-
Challenge
Admin Controller
Now, you'll put together a Spring MVC Controller named
AdminController
. This controller will oversee administrative tasks for items, including listing all items, creating a new item, saving an item, editing an item, and deleting an item.Relevant File: /src/main/java/com/pluralsight/auction/AdminController.java
Instructions
- Import Required Classes: Import all the required classes and interfaces. These include
Autowired
,Model
,ModelAttribute
,PathVariable
,GetMapping
,PostMapping
, and theItemRepository
interface that you created in the previous step. - Inject the ItemRepository: Use the
@Autowired
annotation to get an instance ofItemRepository
. Also, create a constructor to set theItemRepository
instance to a class level variable. - Define Controller Methods: Define the following methods:
listItems
: HandlesGET
requests at the "/admin" URL, fetches all items, and adds them to the model.newItem
: HandlesGET
requests at the "/admin/new" URL, creates a newItem
instance, adds it to the model and renders theform.html
template.saveItem
: HandlesPOST
requests at the "/admin/save" URL, saves an item to the repository, and redirects to the "/admin" URL.editItem
: HandlesGET
requests at the "/admin/edit/{id}" URL, fetches an item by its ID, adds it to the model and renders theform.html
template.deleteItem
: HandlesGET
requests at the "/admin/delete/{id}" URL, deletes an item by its ID, and redirects to the "/admin" URL.
- Import Required Classes: Import all the required classes and interfaces. These include
-
Challenge
Thymeleaf template: `admin.html`
Here, you'll construct a Thymeleaf template that presents auction items in a table format for an admin view. This view will provide additional functionalities like creating a new item and editing or deleting existing items.
Relevant File: /src/main/resources/templates/admin.html
Instructions
- Create a Table to Display the Auction Items: Add a table. The table should have headers for ID, Name, Price, Reserve, Seller, and an empty header for actions.
- Add a Link to Create a New Item: Above the table, add a link that navigates to "/admin/new" to create a new auction item.
- Display the Auction Items in the Table: In the table body, create a row for each item in the
items
attribute of the model. For each row:- Display the item's ID, name, price, reserve, and seller in separate cells.
- In the last cell, add links to edit and delete the item. The edit link should navigate to "/admin/edit/{id}", and the delete link should navigate to "/admin/delete/{id}". The
{id}
in the URLs should be replaced with the item's ID.
-
Challenge
Thymeleaf template: `form.html`
In your final step, you'll create a Thymeleaf template for an auction item form. This form will give users the ability to create a new item or edit an existing one.
Relevant File: /src/main/resources/templates/form.html
Instructions
- Create the Form
- In the body of the page, create a
<main>
element. Inside this element, create a<section>
. - In the section, create a
<form>
with the action set to "/admin/save" and the method set to "post". The form should use theitem
attribute of the model as the object.
- In the body of the page, create a
- Add Input Fields to the Form
- In the form, add input fields for the item's ID, name, price, reserve, description, and seller. Use the
th:field
attribute to bind each input field to a property of the item. - The ID field should be a hidden input field.
- Each input field (except for the ID field) should be wrapped in a
<label>
.
- In the form, add input fields for the item's ID, name, price, reserve, description, and seller. Use the
- Add Buttons to the Form
- At the bottom of the form, add a submit button with the text "Save".
- Next to the submit button, add a link with the text "Cancel". The link should navigate back to the admin page.
When submit the form you may receive an error message that says
This content is blocked. Contact the site owner to fix the issue.
. This is a function of the form being submit in an iframe. If you refresh you should see the changes. - Create the Form
-
Challenge
Completion
Congratulations 🎉, on successfully completing this Spring Lab! You've taken important steps in your journey to learn Spring Boot.
Throughout the lab, you've built a complete auction system, starting from the ground up with the AuctionApplication class, moving to defining the Item entity class, and setting up a CrudRepository interface to handle CRUD operations.
You've also gotten hands-on experience with building Spring MVC Controllers, specifically the ItemController and AdminController, each with its own responsibilities in managing auction items.
Moreover, you've learned how to create dynamic Thymeleaf templates for various views, such as listing auction items (
index.html
), presenting an admin view with functionalities (admin.html
), and providing a form for creating or editing items (form.html
).These skills are not only critical for building Spring applications but also lay a strong foundation for your journey in Java web development. Well done on your achievement and keep up the good work!
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.