- Lab
- A Cloud Guru
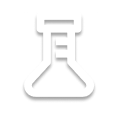
Using the Java Client Library for Prometheus
Prometheus is a powerful tool for monitoring your applications. However, before Prometheus can provide useful data about your applications, your applications need to be instrumented to provide that data. Luckily, client libraries are available to make this significantly easier in a number of programming languages. In this lab, you will be able to get hands-on with the Prometheus Java client library. You will use the client library to instrument a Java application to provide metrics to a Prometheus server.x
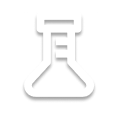
Path Info
Table of Contents
-
Challenge
Add Prometheus client library dependencies to the Java project.
-
Log in to the Prometheus server.
-
Change the directory to the
root
directory for the Java project:
cd /home/cloud_user/content-prometheusdd-limedrop-svc
- Run the project to verify that it is able to compile and run before making any changes:
./gradlew clean bootRun
-
Once you see the text
Started App in X seconds
, the application is running. Usecontrol + c
to stop it. -
Edit
build.gradle
:
vi build.gradle
- Locate the dependencies block and add the Prometheus client library dependencies:
dependencies { implementation 'io.prometheus:simpleclient:0.8.1' implementation 'io.prometheus:simpleclient_httpserver:0.8.1' ... }
- If you want, you can run the project again to automatically download the dependencies and make sure it still works:
./gradlew clean bootRun
-
-
Challenge
Add instrumentation to the /limesAvailable endpoint.
- Edit the controller class:
vi src/main/java/com/limedrop/svc/LimeDropController.java
- Add the requested counter and gauge metrics:
package com.limedrop.svc; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import io.prometheus.client.Counter; import io.prometheus.client.Gauge; @RestController public class LimeDropController { static final Counter totalRequests = Counter.build() .name("total_requests").help("Total requests.").register(); static final Gauge inprogressRequests = Gauge.build() .name("inprogress_requests").help("Inprogress requests.").register(); @GetMapping(path="/limesAvailable", produces = "application/json") public String checkAvailability() { inprogressRequests.inc(); totalRequests.inc(); String response = "{\"warehouse_1\": \"58534\", \"warehouse_2\": \"72399\"}"; inprogressRequests.dec(); return response; } }
- Run the application again to make sure it compiles:
./gradlew clean bootRun
-
Challenge
Add a scrape endpoint to the application.
- Edit the main application class:
vi src/main/java/com/limedrop/svc/App.java
- Use the Prometheus
HTTPServer
to set up a scrape endpoint on port8081
:
package com.limedrop.svc; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import io.prometheus.client.exporter.HTTPServer; import java.io.IOException; @SpringBootApplication public class App { public static void main(String[] args) { SpringApplication.run(App.class, args); try { HTTPServer server = new HTTPServer(8081); } catch (IOException e) { e.printStackTrace(); } } }
- Rerun the application to make sure it compiles:
./gradlew clean bootRun
While the application is still running, you should be able to access the
/limesAvailable
endpoint athttp://<Prometheus Server Public IP>:8080
. You can view the metrics athttp://<Prometheus Server Public IP>:8081/metrics
.You should be able to access the
/limesAvailable
endpoint and see thetotal_requests
counter increase each time you access the endpoint. -
Challenge
Test your setup by configuring Prometheus to scrape from your application.
- Edit the Prometheus config:
sudo vi /etc/prometheus/prometheus.yml
- Add a scrape config to scrape metrics for your app:
scrape_configs: ... - job_name: 'LimeDrop Java Svc' static_configs: - targets: ['localhost:8081']
- Restart Prometheus to reload the config:
sudo systemctl restart prometheus
- Run your Java app and leave it running to allow Prometheus to collect metrics:
./gradlew clean bootRun
- Access Prometheus in a browser at
http://<Prometheus Server Public IP>:9090
. Run queries to see the metrics you are collecting for your Java app:
total_requests inprogress_requests
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.
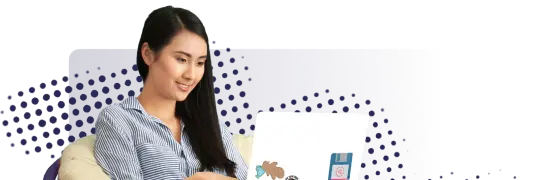
