- Lab
- Cloud
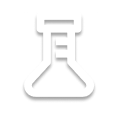
Making Calculations from User Input with Python
After learning about data structures, user input and output programming becomes a lot more powerful. There are many things that we can accomplish by simply taking user input, running it through a process, and returning some useful output. To put these skills to use, we'll write a simple tool to perform temperature conversions from Fahrenheit to Celsius. To feel comfortable completing this lab you'll want to know how to do the following: * Handling user input using the `input` function. Watch the videos from the "Input and Output Operations" section of the Certified Entry-Level Python Programmer Certification course. * Type casting from strings to floats. Watch the "Type Casting" video from the Certified Entry-Level Python Programmer Certification course. * Printing to the screen. Watch the videos from the "Input and Output Operations" section of the Certified Entry-Level Python Programmer Certification course.
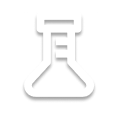
Path Info
Table of Contents
-
Challenge
Create the ~/to-celsius.py Script and Make It Executable with python3.7
For the time being, we're going to write our script in our home directory (
~
) and we want to be able to run it right away. To make sure that we're not completely tied to the path of ourpython3.7
binary, we need to we set up our shebang properly.Let's create the file and set the shebang:
~/to-celsius.py
#!/usr/bin/env python3 # Python implementation here
Now that the file is created, we need to also make it executable by using
chmod
:$ chmod u+x ~/to-celsius.py
Now we can run our script by running
./to-celsius.py
from our home directory. -
Challenge
Prompt and Store Fahrenheit Value from User
When we run our script, we would like to prompt the user for a temperature value in Fahrenheit. Let's do this using the
input
function:~/to-celsius.py
#!/usr/bin/env python3 # Python implementation here fahrenheit = input("What temperature (in Fahrenheit) would you like converted to Celsius? ")
By default, this variable will be a string, so we'll need to cast it to be a float:
~/to-celsius.py
#!/usr/bin/env python3 # Python implementation here fahrenheit = float(input("What temperature (in Fahrenheit) would you like converted to Celsius? "))
Now we're ready to use this value in our calculation.
-
Challenge
Calculating the Celsius Value
The Fahrenheit to Celsius formula is
celsius = (temp - 32) * 5 / 9
and this converts almost perfectly to Python. We only need to changetemp
to be our variablefahrenheit
.~/to-celsius.py
#!/usr/bin/env python3 # Python implementation here fahrenheit = float(input("What temperature (in Fahrenheit) would you like converted to Celsius? ")) celsius = (fahrenheit - 32) * 5 / 9
-
Challenge
Print the Calculated Value to the Screen
Now that we have our final value we're ready to print it to the screen in the form of:
FAHRENHEIT F is CELSIUS C
We can do this by passing our values into the
print
function:~/to-celsius.py
#!/usr/bin/env python3 # Python implementation here fahrenheit = float(input("What temperature (in Fahrenheit) would you like converted to Celsius? ")) celsius = (fahrenheit - 32) * 5 / 9 print(fahrenheit, "F is", celsius, "C")
Now if we use our script we should see the following:
$ ./to-celsius.py What temperature (in Fahrenheit) would you like converted to Celsius? 68.18 68.18 F is 20.100000000000005 C
We're showing more decimal places in our Celsius value than we might like and these can be hidden by using the
round
function to round to 2 decimal places like thisround(celsius, 2)
.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.