- Lab
- Cloud
- Security
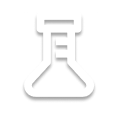
Implementing IAM Policies for AWS Lambda
IAM (Identity and Access Management) policies play a crucial role in AWS Lambda access control. These policies allow you to define fine-grained permissions for different AWS resources, including Lambda functions. In this lab, you will attach an IAM policy that provides an Amazon Lambda function with permission to interact with an Amazon S3 bucket.
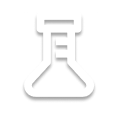
Path Info
Table of Contents
-
Challenge
Create a Lambda function
Create a Lambda function using the code below. Be sure to include the S3 bucket name.
import boto3 def lambda_handler(event, context): # Specify the S3 bucket name s3_bucket = "" # Specify the new blank file key new_file_key = "blank_file.txt" # Initialize the S3 client s3_client = boto3.client('s3') # Create a blank text file content blank_content = '' try: # Put the blank text file into the specified S3 bucket s3_client.put_object(Body=blank_content, Bucket=s3_bucket, Key=new_file_key) print(f"Blank file created: s3://{s3_bucket}/{new_file_key}") return { 'statusCode': 200, 'body': 'Blank file creation complete!' } except Exception as e: print(f"Error: {str(e)}") return { 'statusCode': 500, 'body': 'Error creating blank file' }
-
Challenge
Add an event trigger to the Lambda function
Add an event trigger to the Lambda function that triggers for "all new object create events" within the S3 bucket.
-
Challenge
Attach an IAM policy to the Lambda function
Navigate to the IAM dashboard and attach the AmazonS3FullAccess AWS managed IAM policy to the Lambda function's role.
-
Challenge
Upload file object to S3 bucket
Upload a file of your choice to the S3 bucket.
-
Challenge
Confirm that the S3 event triggered the Lambda function to create a file within the S3 bucket
Confirm that the upload of a file object to the S3 bucket triggered the Lambda function to create a blank text file in the same S3 bucket.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.