- Lab
- Cloud
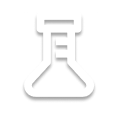
Building a Go Command Line Tool
Go is a great language for building tools. In this learning activity, you'll go through the process of building a complete command line application. By the time we've finished this activity, you'll have gone from a list of features and a desired user experience to having a fully built CLI written in Go. You'll learn how to create custom structs and work with JSON along the way.
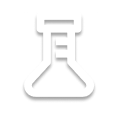
Path Info
Table of Contents
-
Challenge
Create `hr` Package within the `$GOPATH`
Our project needs to be created. We'll do so by creating a directory for us to add our source files.
-
Challenge
Create `main` Package with `User` Struct to Hold Information Parsed from `/etc/passwd`
Our list of users has a very specific shape, and we can represent that shape using a struct. Let's create a
main.go
file and add aUser
struct to it that handles thename
,id
,home
, andshell
values. -
Challenge
Parse Information from `/etc/passwd` into a Slice of `User` Structs
We're going to create a function called
collectUsers
that parses the/etc/passwd
file and returns a slice ofUser
structs. Now we'll be able to use the user information in the main portion of our program. -
Challenge
Define and Parse the `path` and `format` Flags
To keep things manageable, we're going to define our flags in a separate function that performs the following steps:
- Defines the
path
andformat
flags - Parses flags
- Validates the user-provided information. If there is an error, then exits and displays an error.
- Returns
path
andformat
values
- Defines the
-
Challenge
Write User Information as CSV or JSON Information Either to a File or stdout
We want to write the content to either a file or stdout, and thankfully they both adhere to the
io.Writer
interface. Because of this interface, we can keep our writing logic ignorant of whether we're writing to a file or stdout. Here's what we need to implement, in order:- Parse flags into variables using
parseFlags
. - Gather users using
collectUsers
. - Create an
io.Writer
variable. - Assign a value to the
io.Writer
variable based on thepath
variable. This variable should be a file if thepath
variable is set oros.Stdout
otherwise. - If the
format
variable is"json"
, utilize thejson.MarshalIntent
function to pretty-print the users to a slice of bytes before using theWrite
function on ourio.Writer
variable. OR - If the
format
variable is"csv"
, write the header line to theio.Writer
, create a newcsv.Writer
from theio.Writer
, and write a line for each of the users.
- Parse flags into variables using
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.